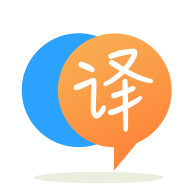
[英]How do I convert unicode characters (ASCII 10) to text in Python
[英]How do I use ASCII (and not Unicode) in Python?
我正在嘗試通過獲取字母的 ASCII 索引並從中添加/減去,在 Python 中制作凱撒密碼加密器/解密器。 但是,它似乎正在使用 Unicode 代替,因為我得到帶有重音符號的字母而不僅僅是英文字母。 例如,如果我輸入單詞“weasel”並將其加密 2,我得到的是“yÞĿƲȗʃ”。 如何使用 ASCII?
這是我的代碼:
def manipulate(text, key, e_or_d):
ciphertext = ''
for i in text:
asciiCode = ord(i)
if e_or_d == 'e':
key = ord(i) + key
elif e_or_d == 'd':
key = ord(i) - key
newChar = chr(key)
ciphertext += newChar
if e_or_d == 'e':
print('Here is your encrypted text: ')
elif e_or_d == 'd':
print('Here is your decrypted text: ')
print(ciphertext)
text = input('Enter text: ')
e_or_d = input('Enter \'e\' for encryption or \'d\' for decryption: ')
key = int(input('Enter encryption key: '))
manipulate(text, key, e_or_d)
我這里使用了chr
和ord
來將一個索引變成一個字符,並分別標識一個字符的索引。
對不起,如果這是不連貫和雜亂無章的。
它正在使用ascii。 對於循環中的每次迭代,您都通過ord(i)
增加key
。 所以它不是恆定的。 使用新變量而不是key
,它應該可以工作。
def manipulate(text, key, e_or_d):
ciphertext = ''
for i in text:
asciiCode = ord(i)
if e_or_d == 'e':
temp = ord(i) + key
elif e_or_d == 'd':
temp = ord(i) - key
newChar = chr(temp)
ciphertext += newChar
if e_or_d == 'e':
print('Here is your encrypted text: ')
elif e_or_d == 'd':
print('Here is your decrypted text: ')
print(ciphertext)
注意:我認為對e_or_d
使用 boolean 值會更好。 還要保持變量名稱一致,例如僅使用駝峰式大小寫或僅使用_
。
有幾點需要注意:
ord(a)-ord(z)
范圍從 97-122,即使在改變點 1 之后,你也可能有意想不到的結果,例如, manipulate("a", 1, "d")
是`
和manipulate("z", 1, "e")
是{
,因為它們在操作后超出范圍。我想用戶只會輸入小寫字母,如果他們會輸入大寫字母或其他字符,則需要額外檢查,這里的代碼只改變了上面的2點:
def manipulate(text, key, e_or_d):
ciphertext = ''
if e_or_d == "e":
step = 1
action = "encrypted"
elif e_or_d == "d":
step = -1
action = "decrypted"
for i in text:
# * step means + key in encryption and - key in decryption
new_ord = ord(i) + (key * step)
# check if new_ord is inside the range of a-z
if 97 <= new_ord <= 122:
new_char = chr(new_ord)
else:
# if new_ord > 122, it will - 26, elif new_ord < 97, it will + 26
new_char = chr(new_ord - (26 * step))
ciphertext += new_char
print(f'Here is your {action} text: ')
print(ciphertext)
text = input('Enter text: ')
key = int(input('Enter encryption key: '))
e_or_d = input('Enter \'e\' for encryption or \'d\' for decryption: ')
manipulate(text, key, e_or_d)
Output:
Enter text: weasel
Enter encryption key: 2
Enter 'e' for encryption or 'd' for decryption: e
Here is your encrypted text:
ygcugn
Enter text: a
Enter encryption key: 1
Enter 'e' for encryption or 'd' for decryption: d
Here is your decrypted text:
z
Enter text: z
Enter encryption key: 1
Enter 'e' for encryption or 'd' for decryption: e
Here is your encrypted text:
a
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.