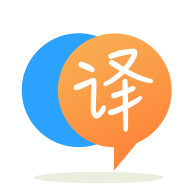
[英]Write a C program to arrange the numbers in an array as a series of odd and even numbers
[英]Want a C program to write into a file, read it and store even and odd numbers in a separate file
我試圖制作以下代碼。
#include<stdio.h>
#include<math.h>
#include<stdlib.h>
void main()
{
FILE *fp1,*fp2,*fp3;
int n,i,size;
printf("Enter no.of digits: ");
scanf("%d",&size);
fp1=fopen("NUMBER.txt","w");
printf("Enter the numbers: \n");
for(i=0;i<size;i++)
{
fflush(stdin);
scanf(" %d",&n);
fputc(n,fp1);
}
fclose(fp1);
fp1=fopen("NUMBER.txt","r");
fp2=fopen("EVEN.txt","w");
fp3=fopen("ODD.txt","w");
while((n=fgetc(fp1))!=EOF)
{
if(n%2==0)
fputc(n,fp2);
else
fputc(n,fp3);
}
fclose(fp1);
fclose(fp2);
fclose(fp3);
fp1=fopen("NUMBER.txt","r");
fp2=fopen("EVEN.txt","r");
fp3=fopen("ODD.txt","r");
printf("The content of number file are: ");
while((n=fgetc(fp1))!=EOF)
printf(" %d",n);
printf("\nThe content of even file are: ");
while((n=fgetc(fp2))!=EOF)
printf(" %d",n);
printf("\nThe content of odd file are: ");
while((n=fgetc(fp3))!=EOF)
printf(" %d",n);
fclose(fp1);
fclose(fp2);
fclose(fp3);
}
我面臨的問題是文件的內容是十六進制或二進制。 我希望它可以用文本編輯器而不是十六進制編輯器來閱讀。 我面臨的另一個問題是 scanf() 不接受 3 位數字。 output 如下所示。
輸入位數:5 輸入數字:123 34 456 67 789 數字文件內容為:123 34 200 67 21 偶數文件內容為:34 200 奇數文件內容為:123 67 21
我嘗試編寫以下代碼:
#include <stdio.h>
#include <stdlib.h>
int main()
{
FILE *fp1,*fp2,*fp3;
int size,n,a,b;
printf("Enter the size: ");
scanf("%d",&size);
fp1=fopen("numbers1.txt","w");
fp2=fopen("even1.txt","w");
fp3=fopen("odd1.txt","w");
printf("Enter the integers: ");
for(int i=0;i<size;i++)
{
scanf(" %d",&n);
//putc(n,fp1); reads as 12, 234 etc but digits in file is not visible
fprintf(fp1," %d\n",n);//reads 12, 234 as 12234 but digits in file are visible
}
fclose(fp1);
fp1=fopen("numbers1.txt","r");
n=getc(fp1);
while(n!=EOF)
{
if(n%2==0)
putc(n,fp2);
else if(n%2==1)
putc(n,fp3);
n=getc(fp1);
}
fclose(fp1);
fclose(fp2);
fclose(fp3);
return 0;
}
在上面的代碼中,文件中的內容是文本,但是奇偶文件是逐字符讀取的。 奇數、偶數和數字文件的內容都給出了打擊。
奇數檔案:133577
偶數文件:2 4 46 6 8
號碼文件:123 34 456 67 78
請幫我
在給出數據之前要求指定數據點的數量幾乎總是一個壞主意。 通常,這樣做的唯一原因是簡化代碼,這樣您就不需要增長數據結構。 但是,在這種情況下,您正在將所有數據寫入文件,因此您甚至不需要這樣做,因為該文件為您提供了存儲空間。
要編寫人類可讀的整數,您需要格式化數據。 最常見的方法是使用printf
。 有很多方法可以滿足您的要求,這只是一個想法:
#include <limits.h>
#include <stdio.h>
#include <stdlib.h>
struct named_file {
const char *name;
FILE *fp;
};
void xfopen(struct named_file *f, const char *mode);
void xfclose(struct named_file *f);
void display(const struct named_file *f);
int
main(void)
{
struct named_file f[3];
int n;
f[0].name = "NUMBER.txt";
f[1].name = "EVEN.txt";
f[2].name = "ODD.txt";
xfopen(f, "w");
while( scanf("%d", &n) == 1 ){
fprintf(f[0].fp, "%d\n", n);
}
xfclose(f);
xfopen(f + 0, "r");
xfopen(f + 1, "w");
xfopen(f + 2, "w");
while( fscanf(f[0].fp, "%d", &n) == 1 ){
FILE *out = n % 2 ? f[2].fp : f[1].fp;
fprintf(out, "%d\n", n);
}
for( int i = 0; i < 3; i += 1 ){
xfclose(f + i);
xfopen(f + i, "r");
display(f + i);
xfclose(f + i);
}
}
void
xfopen(struct named_file *f, const char *mode)
{
f->fp = fopen(f->name, mode);
if( f->fp == NULL ){
perror(f->name);
exit(EXIT_FAILURE);
}
}
void
xfclose(struct named_file *f)
{
if( fclose(f->fp) ){
perror(f->name);
exit(EXIT_FAILURE);
}
}
void
display(const struct named_file *f)
{
int n;
printf("The contents of %s: ", f->name);
while( fscanf(f->fp, "%d", &n) == 1 ){
printf(" %d",n);
}
putchar('\n');
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.