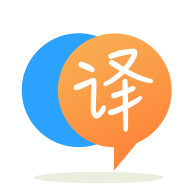
[英]How to get rid of wait time when a key is kept pressed in handling keyboard events in GLUT?
[英]How to check if key not pressed in GLUT?
我在 OpenGL 中制作游戲,現在,我的相機中有一個錯誤:
shift+l
或shift+k
(移動相機),相機將移動,直到我按下另一個鍵。 要測試我的相機,請在if
語句中的playerCameraFunc()
function 之后的 display function 中添加createKillerBlock(x, y)
(例如createKillerBlock(20, 20)
)。
這是我的代碼:
#include <stdbool.h>
#include <GL/glut.h>
#define WINDOW_X 1920
#define WINDOW_Y 1080
#define DEFAULT_PLAYER_SPAWN_X 3.0
#define DEFAULT_PLAYER_SPAWN_Y 3.0
#define DEFAULT_CAMERA_X 0.0
#define DEFAULT_CAMERA_Y 0.0
#define UNKILLABLE_LOCATION 1.01
bool isGameStart = false;
int shiftLPresses = 0;
int shiftKPresses = 0;
int shiftXPresses = 0;
int shiftCPresses = 0;
char pressedKey1, pressedKey2;
float blockSize = 10;
float playerX = DEFAULT_PLAYER_SPAWN_X;
float playerY = DEFAULT_PLAYER_SPAWN_Y;
float playerR = 255;
float playerG = 255;
float playerB = 255;
float playerMoveSpeed = 0.7;
float cameraX = DEFAULT_CAMERA_X;
float cameraY = DEFAULT_CAMERA_Y;
float cameraMoveSpeed = 7.1;
float killerBlockX = UNKILLABLE_LOCATION;
float killerBlockY = UNKILLABLE_LOCATION;
float killerBlockR = 255;
float killerBlockG = 0;
float killerBlockB = 0;
void gameMenu()
{
if (isGameStart == false)
{
glBegin(GL_LINES);
glColor3f(1.0, 1.0, 1.0);
glVertex2f(-0.2, -0.2);
glVertex2f(0.2, -0.2);
glVertex2f(0.2, -0.2);
glVertex2f(0.2, 0.2);
glVertex2f(0.2, 0.2);
glVertex2f(-0.2, 0.2);
glVertex2f(-0.2, 0.2);
glVertex2f(-0.2, -0.2);
glEnd();
glBegin(GL_POLYGON);
glVertex2f(-0.1, -0.1);
glVertex2f(0.1, 0.0);
glVertex2f(-0.1, 0.1);
glEnd();
}
}
void startGame()
{
isGameStart = true;
}
void spawnPlayer()
{
glBegin(GL_POLYGON);
glColor3f(playerR / 255, playerG / 255, playerB / 255);
glVertex2f(-1.0 + (playerX * (blockSize / WINDOW_X)), -1.0 + (playerY * (blockSize / WINDOW_Y)));
glVertex2f(-1.0 + (playerX * (blockSize / WINDOW_X) + (blockSize / WINDOW_X)), -1.0 + (playerY * (blockSize / WINDOW_Y)));
glVertex2f(-1.0 + (playerX * (blockSize / WINDOW_X) + (blockSize / WINDOW_X)), -1.0 + (playerY * (blockSize / WINDOW_Y) + (blockSize / WINDOW_Y)));
glVertex2f(-1.0 + (playerX * (blockSize / WINDOW_X)), -1.0 + (playerY * (blockSize / WINDOW_Y) + (blockSize / WINDOW_Y)));
glEnd();
}
void playerCameraFunc()
{
glMatrixMode(GL_PROJECTION);
glLoadIdentity();
glTranslatef(cameraX * (blockSize / WINDOW_X), cameraY * (blockSize / WINDOW_Y), 0);
}
void killPlayer()
{
isGameStart = false;
pressedKey1 = '\0';
pressedKey2 = '\0';
playerX = DEFAULT_PLAYER_SPAWN_X;
playerY = DEFAULT_PLAYER_SPAWN_Y;
cameraX = DEFAULT_CAMERA_X;
cameraY = DEFAULT_CAMERA_Y;
glutPostRedisplay();
}
void playerKillFunc()
{
if (playerX >= killerBlockX - 1.0 & playerY >= killerBlockY - 1.0 & playerX < killerBlockX + 1.0 & playerY <= killerBlockY + 1.0)
{
if (killerBlockX != UNKILLABLE_LOCATION & killerBlockY != UNKILLABLE_LOCATION)
{
killPlayer();
}
}
}
void createKillerBlock(int x, int y)
{
killerBlockX = x;
killerBlockY = y;
glBegin(GL_POLYGON);
glColor3f(killerBlockR / 255, killerBlockG / 255, killerBlockB / 255);
glVertex2f(-1.0 + (x * (blockSize / WINDOW_X)), -1.0 + (y * (blockSize / WINDOW_Y)));
glVertex2f(-1.0 + (x * (blockSize / WINDOW_X) + (blockSize / WINDOW_X)), -1.0 + (y * (blockSize / WINDOW_Y)));
glVertex2f(-1.0 + (x * (blockSize / WINDOW_X) + (blockSize / WINDOW_X)), -1.0 + (y * (blockSize / WINDOW_Y) + (blockSize / WINDOW_Y)));
glVertex2f(-1.0 + (x * (blockSize / WINDOW_X)), -1.0 + (y * (blockSize / WINDOW_Y) + (blockSize / WINDOW_Y)));
glEnd();
playerKillFunc();
}
void timer1()
{
glutTimerFunc(1000 / 60, timer1, 0);
switch (pressedKey1)
{
case 'w':
if (isGameStart == true)
{
playerY += playerMoveSpeed / (60 * (shiftXPresses + 1) / (shiftCPresses + 1));
}
break;
case 'a':
if (isGameStart == true)
{
playerX -= playerMoveSpeed / (60 * (shiftXPresses + 1) / (shiftCPresses + 1));;
}
break;
case 's':
if (isGameStart == true)
{
playerY -= playerMoveSpeed / (60 * (shiftXPresses + 1) / (shiftCPresses + 1));
}
break;
case 'd':
if (isGameStart == true)
{
playerX += playerMoveSpeed / (60 * (shiftXPresses + 1) / (shiftCPresses + 1));
}
break;
}
glutPostRedisplay();
}
void timer2()
{
glutTimerFunc(1000 / 60, timer2, 0);
switch (pressedKey2)
{
case 76:
if (isGameStart == true)
{
cameraX -= cameraMoveSpeed / (60 * (shiftLPresses + shiftKPresses + 1));
playerX += cameraMoveSpeed / (60 * (shiftLPresses + shiftKPresses + 1));
}
break;
case 75:
if (isGameStart == true)
{
cameraX += cameraMoveSpeed / (60 * (shiftKPresses + shiftLPresses + 1));
playerX -= cameraMoveSpeed / (60 * (shiftKPresses + shiftLPresses + 1));
}
break;
}
glutPostRedisplay();
}
void display()
{
glClear(GL_COLOR_BUFFER_BIT);
gameMenu();
if (isGameStart == true)
{
spawnPlayer();
playerCameraFunc();
}
glFlush();
}
void keyboard(unsigned char key, int x, int y)
{
switch (key)
{
case 'w':
if (isGameStart == true)
{
pressedKey1 = 'w';
pressedKey2 = '\0';
timer1();
}
break;
case 'a':
if (isGameStart == true)
{
pressedKey1 = 'a';
pressedKey2 = '\0';
timer1();
}
break;
case 's':
if (isGameStart == true)
{
pressedKey1 = 's';
pressedKey2 = '\0';
timer1();
}
break;
case 'd':
if (isGameStart == true)
{
pressedKey1 = 'd';
pressedKey2 = '\0';
timer1();
}
break;
case 76:
if (isGameStart == true)
{
shiftLPresses += 1;
pressedKey2 = 76;
timer2();
}
break;
case 75:
if (isGameStart == true)
{
shiftKPresses += 1;
pressedKey2 = 75;
timer2();
}
break;
case 88:
if (isGameStart == true)
{
shiftXPresses += 1;
pressedKey2 = '\0';
timer1();
}
break;
case 67:
if (isGameStart == true)
{
shiftCPresses += 1;
pressedKey2 = '\0';
timer1();
}
break;
case 13:
if (isGameStart == false)
{
startGame();
timer1();
}
break;
default:
pressedKey2 = '\0';
}
glutPostRedisplay();
}
int main(int argc, char **argv)
{
glutInit(&argc, argv);
glutInitDisplayMode(GLUT_SINGLE);
glutCreateWindow("OpenAttack1");
glutFullScreen();
glutDisplayFunc(display);
glutKeyboardFunc(keyboard);
glutSetCursor(GLUT_CURSOR_NONE);
glutMainLoop();
}
編輯
如果按下shift+l
或shift+k
鍵,我想移動相機,但如果未按下 shift+ shift+l
或shift+k
+k,則停止移動相機。
升級到像FreeGLUT這樣的 GLUT 4+ 實現並注冊一個glutKeyboardUpFunc()
/ glutSpecialUpFunc()
回調以在釋放鍵時獲得通知:
添加了幾個新的回調,並且幾個特定於 Silicon Graphics 硬件的回調尚未實現。 大多數或所有新回調都列在 GLUT 版本 4“glut.h”header 文件中,但未將其納入文檔。 新回調包括常規和特殊按鍵釋放回調、操縱桿回調、菜單 state 回調(帶有一個參數,不同於具有三個參數的菜單狀態回調)、一個 window 狀態回調(也帶有一個參數)和一個window position 回調。 不支持的回調是兩個 Tablet 回調。 如果用戶需要不支持的回調,他應該聯系 freeglut 開發團隊。
用法示例。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.