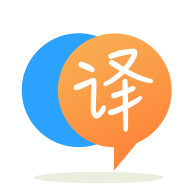
[英]Python 3 TypeError: unsupported operand type(s) for ** or pow(): 'str' and 'int'
[英]TypeError: unsupported operand type(s) for ** or pow(): 'int' and 'set'
問題:不使用任何字符串方法,嘗試打印以下內容: 123...n 請注意,“...”表示其間的連續值。
示例 n=5 打印 12345。
我的解決方案
n = int(input())
sum=0
i=n
while i>0:
sum=sum + i*(10**{n-i})
i -= 1
print(sum)
首先:如果 n=0, {ni}
將評估為{-1}
,因為{x}
是在 python 中表達集合的方式
第二:您要求打印數字字符串的方法,但沒有字符串操作(因此所有連接都應該在兩個整數之間進行)。 這里我假設接受的輸入只能是正數
例如:
在學習解決此類“挑戰”問題時,最好進行測試驅動:編寫一個可以正常工作的簡單程序,然后與生成的預期結果進行比較/斷言
這是生成 output(使用字符串操作)的正確但不可接受的方法:
inp = int(input())
expected = ""
for i in range(1, inp+1):
expected = expected + str(i)
print(expected)
然后嘗試逐步解決它:僅假設單個數字輸入。 在這里,我們的想法是,為了將一個數字放在另一個數字旁邊,我們需要將第一個數字乘以 10,然后將下一個數字乘以 1。因此,將其乘以 10 的冪的解決方案已經在正確的軌道上
現在我們可以寫:
inp = int(input())
result = 0
for i in range(1, inp+1):
power_of_ten = 10**(inp-i)
print("pot", power_of_ten)
result = result + (i*power_of_ten)
print("r", result)
print(result)
output:
5
pot 10000
r 10000
pot 1000
r 12000
pot 100
r 12300
pot 10
r 12340
pot 1
r 12345
12345
此時,我們可以嘗試斷言我們的 output 是否與我們生成的 output 相同(使用字符串操作的那個):
inp = int(input())
result = 0
for i in range(1, inp+1):
power_of_ten = 10**(inp-i)
result = result + (i*power_of_ten)
print(result)
expected = ""
for i in range(1, inp+1):
expected = expected + str(i)
print(expected)
assert(result == int(expected))
print("assertion passed")
output:
5
12345
12345
assertion passed
但如果我們使用兩位輸入,output 將不再正確:
12
123456790122
123456789101112
Traceback (most recent call last):
File "/tmp/c.py", line 14, in <module>
assert(result == int(expected))
AssertionError
所以,如果我們必須在輸入 12 時 output 123456789101112 ,那么我們需要一個數學 function (不是字符串函數)來計算數字中的位數:
這樣的 function 稱為對數 function:例如:
math.floor(math.log(i, 10)) + 1
首先我們嘗試對輸入以 10 為底,然后我們將結果取底(這樣結果就不是小數/小數); 然后我們加 1
這是包含它的代碼:請注意,為簡單起見,我們正在向后循環(例如:12,11,10,9..1)
import math
inp = int(input())
result = 0
pad = 0
for i in range(inp, 0, -1):
result = result + i*10**pad
pad = pad + math.floor(math.log(i, 10)) + 1
print(result)
expected = ""
for i in range(1, inp+1):
expected = expected + str(i)
print(expected)
assert(result == int(expected))
print("assertion passed")
在這里我添加了一個變量pad
,它將包含下一次迭代要添加的 pad 的數量,例如:input=5
當輸入=12
output:
$ python3 /tmp/a.py
5
12345
12345
assertion passed
$ python3 /tmp/a.py
12
123456789101112
123456789101112
assertion passed
所以最終的代碼是:
import math
inp = int(input())
result = 0
pad = 0
for i in range(inp, 0, -1):
result = result + i*10**pad
pad = pad + math.floor(math.log(i, 10)) + 1
print(result)
n = int(input())
s, m = 0, 1
for i in range(n, 0, -1):
s += i*m
m *= 10
print(s)
chepner 的評論和Kristian 的回答解決了您收到該錯誤的原因。
我想知道,你甚至需要做任何算術嗎? 這提供了一個字符串作為print
的參數,但實際上並不使用任何字符串方法(例如join
)。 有人可能會爭辯說,這遵循了“不使用任何字符串方法......”的字母(如果不是精神的話)。
n = int(input("Enter a number: "))
# Generate a list of the numbers from 1 through n.
numbers = list(range(1, n + 1))
# Print out all the numbers, without spaces between them.
print(*numbers, sep='')
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.