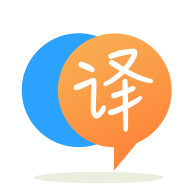
[英]How to reboot the cisco switch with Netmiko SSH (Python)?
[英]How to do ssh on multiple cisco devices using netmiko in python
我正在嘗試為多個設備運行下面的腳本,它僅適用於根據以下腳本的最后一個設備。
請您驗證以下腳本,因為我需要使用 for 循環語句執行兩個設備輸出。
from netmiko import ConnectHandler
from getpass import getpass
password= getpass()
RTR_01 = {
'device_type': 'cisco_ios',
'host': '10.10.10.10',
'username': 'admin',
'password': password,
}
RTR_02 = { 'device_type': 'cisco_ios',
'host': '10.10.10.11',
'username': 'admin',
'password': password, }
device_list = [RTR_01,RTR_02]
for device in device_list: print('Connecting to the device :' + device ['host'])
net_connect = ConnectHandler(**device)
output = net_connect.send_command('show ip interface brief')
print(output)
output = net_connect.send_command('show version')
print(output)
我現在如何 100% 正確工作。 我曾嘗試過使用適當的縮進的net_connect.disconnect()
,但它不起作用,因為一旦退出循環的縮進,它就會自動退出。 問題雖然很愚蠢,但我仍然連接到實際設備並收到一個我忽略的錯誤,抱怨無法創建/var/home/myusername/.ssh
我需要做的就是在循環的最后發出以下命令:
net_connect.write_channel('exit\\n')
諸如愚蠢的錯誤和浪費了這么多時間,但教訓是寶貴的! 也許它可以幫助這里的其他人。
你需要這些行在 for 循環中縮進
net_connect = ConnectHandler(**device)
output = net_connect.send_command('show ip interface brief')
print(output)
output = net_connect.send_command('show version')
print(output)
您的代碼應如下所示:
from getpass import getpass
from netmiko import ConnectHandler
password = getpass()
RTR_01 = {
"device_type": "cisco_ios",
"host": "10.10.10.10",
"username": "admin",
"password": password,
}
RTR_02 = {
"device_type": "cisco_ios",
"host": "10.10.10.11",
"username": "admin",
"password": password,
}
device_list = [RTR_01, RTR_02]
for device in device_list:
print("Connecting to the device :" + device["host"])
net_connect = ConnectHandler(**device)
output = net_connect.send_command("show ip interface brief")
print(output)
output = net_connect.send_command("show version")
print(output)
net_connect.disconnect() # to clear the vty line when done
這是你的代碼的更好版本,它做同樣的事情:
from getpass import getpass
from netmiko import ConnectHandler
password = getpass()
ipaddrs = ["10.10.10.10", "10.10.10.11"]
# A list comprehension
devices = [
{
"device_type": "cisco_ios",
"host": ip,
"username": "admin",
"password": password,
}
for ip in ipaddrs
]
for device in devices:
print(f'Connecting to the device: {device["host"]}')
with ConnectHandler(**device) as net_connect: # Using Context Manager
intf_brief = net_connect.send_command(
"show ip interface brief"
) # Inside the connection
facts = net_connect.send_command("show version") # Inside the connection
# Notice here I didn't call the `net_connect.disconnect()`
# because the `with` statement automatically disconnects the session.
# On this indentation level (4 spaces), the connection is terminated
print(intf_brief)
print(facts)
輸出(
intf_brief
和facts
)在連接外打印,因為不再需要會話來打印任何收集的值。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.