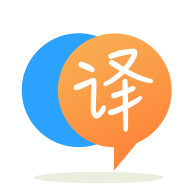
[英]Javascript - How to make scrollbar only visible when you move your mouse inside respective div
[英]How to make a JavaScript canvas element move with your mouse?
我已經知道如何使用 jQuery 使標題移動,而且我還可以在 JavaScript 畫布上繪制內容。 我現在想知道如何結合這兩個原則來使我用鼠標繪制的蜜蜂移動? 我已經在 Sublime Text 3 上試用了我的版本(我使用的是 Windows 7 Professional 硬件和軟件,所以順便說一下,當我嘗試下載 Sublime Text 4 時收到一條錯誤消息)。 這是我的JavaScript/HTML/CSS
:
var canvas = document.getElementById("canvas"); var ctx = canvas.getContext("2d"); var x = 100; var y = 100; var circle = function (x, y, radius, fillCircle) { ctx.beginPath(); ctx.arc(x, y, radius, 0, Math.PI * 2, false); if (fillCircle) { ctx.fill(); } else { ctx.stroke(); } }; var drawBee = function (x, y) { ctx.lineWidth = 2; ctx.strokeStyle = "Black"; ctx.fillStyle = "Gold"; circle(x, y, 8, true); circle(x, y, 8, false); circle(x - 5, y - 11, 5, false); circle(x + 5, y - 11, 5, false); }; drawBee(); var update = $("html").mousemove(function (event) { $(drawBee()).offset({ left: event.pageX, top: event.pageY }); }); setInterval(function () { ctx.clearRect(0, 0, 500, 500); drawBee(x, y); ctx.strokeRect(0, 0, 500, 500); }, 30);
<canvas id="canvas" width="500" height="500"></canvas> <script src="https://code.jquery.com/jquery-2.1.0.js"></script>
請給我一個新的代碼添加到<script>
標簽中(用於將 JavaScript 添加到 HTML 文檔中),並告訴我我的錯誤,以供將來參考。
提前致謝!
如果您需要跟隨鼠標位置在畫布內移動,請使用$("#canvas").mousemove()
和clientX
/ clientY
坐標(相對於畫布)
var canvas = document.getElementById("canvas"); var ctx = canvas.getContext("2d"); var x = 100; var y = 100; var circle = function (x, y, radius, fillCircle) { ctx.beginPath(); ctx.arc(x, y, radius, 0, Math.PI * 2, false); if (fillCircle) { ctx.fill(); } else { ctx.stroke(); } }; var drawBee = function (x, y) { ctx.lineWidth = 2; ctx.strokeStyle = "Black"; ctx.fillStyle = "Gold"; circle(x, y, 8, true); circle(x, y, 8, false); circle(x - 5, y - 11, 5, false); circle(x + 5, y - 11, 5, false); }; drawBee(); var update = $("#canvas").mousemove(function (event) { x=event.clientX; y=event.clientY; }); setInterval(function () { ctx.clearRect(0, 0, 500, 500); drawBee(x, y); ctx.strokeRect(0, 0, 500, 500); }, 30);
<canvas id="canvas" width="500" height="500"></canvas> <script src="https://code.jquery.com/jquery-2.1.0.js"></script>
我個人喜歡使用全局鼠標對象來存儲坐標。 這使我可以在需要的地方輕松插入鼠標坐標。
此外,如果您希望對象以鼠標為中心,則需要在更新鼠標位置時考慮畫布 x 和 y 坐標。 如您所見,我從 event.clientX 和 .clientY 中減去它們
var canvas = document.getElementById("canvas"); var ctx = canvas.getContext("2d"); var mouse = {x: null, y: null}; var circle = function (x, y, radius, fillCircle) { ctx.beginPath(); ctx.arc(x, y, radius, 0, Math.PI * 2, false); if (fillCircle) { ctx.fill(); } else { ctx.stroke(); } }; var drawBee = function () { ctx.lineWidth = 2; ctx.strokeStyle = "Black"; ctx.fillStyle = "Gold"; circle(mouse.x, mouse.y, 8, true); circle(mouse.x, mouse.y, 8, false); circle(mouse.x - 5, mouse.y - 11, 5, false); circle(mouse.x + 5, mouse.y - 11, 5, false); }; drawBee(); $("#canvas").mousemove(function (event) { mouse.x = event.clientX - canvas.getBoundingClientRect().x; mouse.y = event.clientY - canvas.getBoundingClientRect().y; }); setInterval(function () { ctx.clearRect(0, 0, 500, 500); drawBee(); ctx.strokeRect(0, 0, 500, 500); }, 30);
<canvas id="canvas" width="500" height="500"></canvas> <script src="https://code.jquery.com/jquery-2.1.0.js"></script>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.