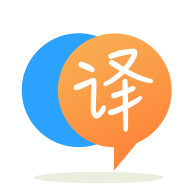
[英]React- Unhandled Rejection (TypeError): Cannot read property 'city' of undefined
[英]React- Unhandled Rejection (TypeError): Cannot read property 'data' of undefined
我正在注冊以創建個人資料。 輸入值后,當我單擊提交時出現此錯誤
配置文件.js
import axios from "axios";
import { setAlert } from "./alert";
import { GET_PROFILE, PROFILE_ERROR } from "./types";
export const getCurrentProfile = () => async (dispatch) => {
try {
const res = await axios.get("/api/profile/me");
dispatch({
type: GET_PROFILE,
payload: res.data,
});
} catch (error) {
dispatch({
type: PROFILE_ERROR,
payload: {
msg: error.response.statusText,
status: error.response.status,
},
});
}
};
//Create or Update profile
export const createProfile =
(formData, history, edit = false) =>
async (dispatch) => {
try {
const config = {
headers: {
"Content=Type": "application/json",
},
};
const res = await axios.post("/api/profile", formData, config);
dispatch({
type: GET_PROFILE,
payload: res.data,
});
dispatch(setAlert(edit ? "Profile Updated" : "Profile Created"));
if (!edit) {
history.push("/dashboard");
}
} catch (error) {
const errors = error.response.data.errors;
if (errors) {
errors.forEach((error) => dispatch(setAlert(error.msg, "danger")));
}
dispatch({
type: PROFILE_ERROR,
payload: {
msg: error.response.statusText,
status: error.response.status,
},
});
}
};
創建配置文件.js
import React, { Fragment, useState } from "react";
import { Link, withRouter } from "react-router-dom";
import { createProfile } from "../../actions/profile";
import { connect } from "react-redux";
import PropTypes from "prop-types";
const CreateProfile = ({ createProfile, history }) => {
const [formData, setFormData] = useState({
status: "",
company: "",
bio: "",
website: "",
location: "",
skills: "",
github_username: "",
twitter: "",
facebook: "",
linkedin: "",
});
const [displaySocialInputs, toggleSocialInputs] = useState(false);
const {
status,
company,
bio,
website,
location,
skills,
github_username,
twitter,
facebook,
linkedin,
} = formData;
const onChange = (e) =>
setFormData({ ...formData, [e.target.name]: e.target.value });
const onSubmit = (e) => {
e.preventDefault();
createProfile(formData, history);
};
return (
<Fragment>
<h1 className='large text-primary'>Create Your Profile</h1>
<p className='lead'>
<i className='fas fa-user'></i> Let's get some information to make your
profile stand out
</p>
<small>* = required field</small>
<form className='form' onSubmit={(e) => onSubmit(e)}>
<div className='form-group'>
<select name='status' value={status} onChange={(e) => onChange(e)}>
<option value='0'>* Select Professional Status</option>
<option value='Developer'>Developer</option>
<option value='Junior Developer'>Junior Developer</option>
<option value='Senior Developer'>Senior Developer</option>
<option value='Manager'>Manager</option>
<option value='Student or Learning'>Student or Learning</option>
<option value='Instructor'>Instructor or Teacher</option>
<option value='Intern'>Intern</option>
<option value='Other'>Other</option>
</select>
<small className='form-text'>
Give us an idea of where you are at in your career
</small>
</div>
<div className='form-group'>
<input
type='text'
placeholder='Company'
name='company'
value={company}
onChange={(e) => onChange(e)}
/>
<small className='form-text'>
Could be your own company or one you work for
</small>
</div>
<div className='form-group'>
<input
type='text'
placeholder='Website'
name='website'
value={website}
onChange={(e) => onChange(e)}
/>
<small className='form-text'>
Could be your own or a company website
</small>
</div>
<div className='form-group'>
<input
type='text'
placeholder='Location'
name='location'
value={location}
onChange={(e) => onChange(e)}
/>
<small className='form-text'>
City & state suggested (eg. Boston, MA)
</small>
</div>
<div className='form-group'>
<input
type='text'
placeholder='* Skills'
name='skills'
value={skills}
onChange={(e) => onChange(e)}
/>
<small className='form-text'>
Please use comma separated values (eg. HTML,CSS,JavaScript,PHP)
</small>
</div>
<div className='form-group'>
<input
type='text'
placeholder='Github Username'
name='githubusername'
value={github_username}
onChange={(e) => onChange(e)}
/>
<small className='form-text'>
If you want your latest repos and a Github link, include your
username
</small>
</div>
<div className='form-group'>
<textarea
placeholder='A short bio of yourself'
name='bio'
value={bio}
onChange={(e) => onChange(e)}
></textarea>
<small className='form-text'>Tell us a little about yourself</small>
</div>
<div className='my-2'>
<button
onClick={() => toggleSocialInputs(!displaySocialInputs)}
type='button'
className='btn btn-light'
>
Add Social Network Links
</button>
<span>Optional</span>
</div>
{displaySocialInputs && (
<Fragment>
<div className='form-group social-input'>
<i className='fab fa-twitter fa-2x'></i>
<input
type='text'
placeholder='Twitter URL'
name='twitter'
value={twitter}
onChange={(e) => onChange(e)}
/>
</div>
<div className='form-group social-input'>
<i className='fab fa-facebook fa-2x'></i>
<input
type='text'
placeholder='Facebook URL'
name='facebook'
value={facebook}
onChange={(e) => onChange(e)}
/>
</div>
<div className='form-group social-input'>
<i className='fab fa-linkedin fa-2x'></i>
<input
type='text'
placeholder='Linkedin URL'
name='linkedin'
value={linkedin}
onChange={(e) => onChange(e)}
/>
</div>
</Fragment>
)}
<input type='submit' className='btn btn-primary my-1' />
<a className='btn btn-light my-1' href='dashboard.html'>
Go Back
</a>
</form>
</Fragment>
);
};
CreateProfile.propTypes = {
createProfile: PropTypes.func.isRequired,
};
export default connect(null, { createProfile })(withRouter(CreateProfile));
在此之前的一切工作正常。我能夠登錄,檢查用戶是否有個人資料,但是這個錯誤:未處理的拒絕(類型錯誤):無法讀取未定義的屬性“數據”我已經檢查了很多來源,但我找不到問題所在。
我的項目沙盒鏈接
我已經解決了這個問題。
所以我遇到的第一個問題是我在創建配置文件時無法輸入 Github 用戶名。
這是因為在我的 CreateProfile.js 中,我的狀態為 github_username,但輸入名稱為 name="githubusername" 並且我正在使用名稱作為狀態鍵來更新狀態,因此該值永遠不會改變。
所以更改名稱以匹配
name="github_username"
之后我可以輸入一個Github用戶名並嘗試提交表單,但它仍然沒有完全解決問題。
該錯誤實際上來自我在 actions/profile.js createProfile 函數中的配置對象,因為我有..
"Content=Type": "application/json",
哪個應該是
內容類型不是內容=類型
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.