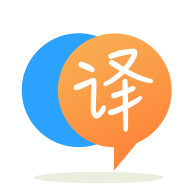
[英]Why is my maximum depth solution for binary tree returning one less than it is supposed to?
[英]maximum depth of a binary tree using BFS- why is the depth doubled what it is supposed to be?
我正在編寫迭代解決方案來查找二叉樹的最大深度 - 使用Breadth First Search (BFS)
:
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
from collections import deque
class Solution:
def maxDepth(self, root: TreeNode) -> int:
if not root:
return 0
queue = deque()
queue.append(root)
depth = 1
level_length = len(queue)
while queue:
for i in range(level_length):
node = queue.popleft()
if node.left:
queue.append(node.left)
if node.right:
queue.append(node.right)
depth +=1
return depth
對於輸入root = [3,9,20,null,null,15,7]
,我得到的是output=6
而不是 3,這顯然是正確的答案。
我已經在紙上多次瀏覽了我的代碼,但似乎看不出它的不足之處 - 有什么想法嗎?
level_length = len(queue)
在遍歷開始前只計算一次,所以它總是 1。這意味着你的內部for
循環永遠不會運行超過一次並且實際上不存在,導致depth += 1
在每個節點上運行。
將長度計算移到while
以便for
循環在增加depth
之前為每個級別運行正確的次數。
Leetcode 需要depth = 0
而不是depth = 1
,但您可能會在修復主要錯誤后弄清楚這一點。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.