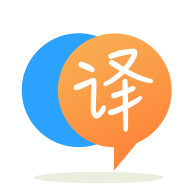
[英]How do I use JavaScript to send an email with the Rails 3 Action Mailer?
[英]How to use mailer in Rails 6.1?
我目前正在嘗試創建一個聯系我們表單,用戶可以在其中向我的個人電子郵件地址發送任何類型的報告。 為了這個例子,我們稱之為my-email-address@email.com
。
目前我不太關心用戶的電子郵件。 假設我將使用以下信息。
from:
"my-email-address@email.com"
to:
"my-email-address@email.com"
subject:
"a subject name"
第 1 步:我使用 AJAX POST 請求在views/home/contact_us.html.erb
創建了我的表單:
<form id="sendEmailForm">
<div class="form-group mb-3">
<input type="email" class="form-control" id="exampleFormControlInput1" placeholder="Enter your email">
</div>
<div class="form-group mb-3">
<input type="text" class="form-control" id="exampleFormControlInput2" placeholder="Enter a subject (Optional)">
</div>
<div class="form-group mb-3">
<textarea class="form-control" placeholder="Please write your name, company-name, and what you would like to achieve." id="exampleFormControlTextarea3" rows="5"></textarea>
</div>
<button type="submit" class="btn btn-primary mb-2">Send Email</button>
</form>
<script type="text/javascript">
$('#sendEmailForm').on('submit', function(e) {
e.preventDefault();
e.stopPropagation();
let final_json_data = {
email: document.getElementById("exampleFormControlInput1").value,
subject: document.getElementById("exampleFormControlInput2").value,
content: document.getElementById("exampleFormControlTextarea3").value
};
jQuery.ajax({
url: '/home/send_email_to_server',
type: "POST",
data: {emailDetails: final_json_data},
success: function(result) {
alert("ajax request OK!");
},
fail: function(result) {
alert("ajax has failed")
}
});
});
</script>
第 2步:我的家庭控制器和 routes.rb:
class HomeController < ApplicationController
def contact_us
puts "GETTING THE PAGE !!!!!!!!!!!!!!!!!!!"
end
def send_email_to_server
@emailDetails = params[:emailDetails]
puts ">>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>"
puts " Store email details on server"
puts @emailDetails['email']
puts @emailDetails['subject']
puts @emailDetails['content']
puts ">>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>"
ContactUsMailer.notify_server_via_email(@emailDetails['email'], @emailDetails['subject']).deliver
puts ">>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>"
end
end
Rails.application.routes.draw do
get 'home/contact_us'
post 'home/send_email_to_server'
end
第 3步:修改application_mailer.rb
以使用默認的from-email
:
class ApplicationMailer < ActionMailer::Base
default from: "my-email-address@email.com"
layout 'mailer'
end
第 4 contact_us_mailer.rb
:修改contact_us_mailer.rb
以使用捕獲的參數處理請求:
class ContactUsMailer < ApplicationMailer
def notify_server_via_email(toEmail, aSubject)
puts ">>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>"
puts " Trying to send an email . . . "
@email = toEmail
@subject = aSubject
puts @email
puts @subject
puts ">>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>"
mail(
to: @email,
subject: @subject
)
end
end
第 4 notify_server_via_email.html.erb
:然后在views/contact_us_mailer
我創建了一個名為notify_server_via_email.html.erb
的新文件並添加了以下內容:
<h1> hello world </h1>
所以這是按順序發生的:
/home/send_email_to_server
mail()
函數但是我收到以下錯誤:
Started POST "/home/send_email_to_server" for ::1 at 2021-07-03 18:01:00 +0300
Processing by HomeController#send_email_to_server as */*
Parameters: {"emailDetails"=>{"email"=>"my-email-address@email.com", "subject"=>"some new subject", "content"=>"a text example"}}
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
Store email details on server
my-email-address@email.com
some new subject
a text example
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
Trying to send an email . . .
my-email-address@email.com
some new subject
>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>>
Rendering layout layouts/mailer.html.erb
Rendering contact_us_mailer/notify_server_via_email.html.erb within layouts/mailer
Rendered contact_us_mailer/notify_server_via_email.html.erb within layouts/mailer (Duration: 0.5ms | Allocations: 70)
Rendered layout layouts/mailer.html.erb (Duration: 1.5ms | Allocations: 241)
ContactUsMailer#notify_server_via_email: processed outbound mail in 14.0ms
Delivered mail 60e07bace69f9_27544024-497@DESKTOP-MQJ3IGG.mail (30045.8ms)
Date: Sat, 03 Jul 2021 18:01:00 +0300
From: my-email-address@email.com
To: my-email-address@email.com
Message-ID: <60e07bace69f9_27544024-497@DESKTOP-MQJ3IGG.mail>
Subject: some new subject
Mime-Version: 1.0
Content-Type: text/html;
charset=UTF-8
Content-Transfer-Encoding: 7bit
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<style>
/* Email styles need to be inline */
</style>
</head>
<body>
<h1> hello world </h1>
</body>
</html>
Completed 500 Internal Server Error in 30095ms (ActiveRecord: 0.0ms | Allocations: 11373)
EOFError (end of file reached):
app/controllers/home_controller.rb:35:in `send_email_to_server'
我不知道是什么導致了 500 內部服務器錯誤。 我目前正在開發方面工作,我知道我不應該這樣做,但這只是為了測試目的,我的目標不是永遠保持這個配置。 另外,我遇到了這個StackOverflow 問題,它與我的問題相似,但沒有明確的答案,因為這是大學 wifi 阻止 smtp 請求工作。 我正在嘗試使用家庭 wifi。
另外作為參考這里是我的development.rb
命令action_mailer
:
config.action_mailer.perform_deliveries = true
config.action_mailer.raise_delivery_errors = true
config.action_mailer.delivery_method = :smtp
config.action_mailer.smtp_settings = {
:address => 'localhost',
:port => 3000
}
config.action_mailer.default_url_options = {host: 'localhost:3000'}
config.action_mailer.perform_caching = false
錯誤由庫net/protocol.rb#227引發。 如果您打開瀏覽器開發人員工具,您可以在子選項卡響應下看到它在選項卡網絡下狀態為 500 的請求。
錯誤原因:庫無法連接到您的 smtp 服務器,根據您的development.rb
配置config.action_mailer.smtp_settings
位於localhost:3000
。 在端口 3000 位於您的 Web 服務器,smtp 通常位於端口 25、587、2525(如果它正在運行)。
如果您希望在開發環境中向my-email-address@email.com
發送郵件,您需要在本地計算機上運行 smtp 服務器並正確配置config.action_mailer.smtp_settings
。
如果您想查看電子郵件,您可以查看控制台或日志。 或者使用 gem letter_opener,或者使用ActionMailer::Preview
。 請參閱StackOverflow 上的答案。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.