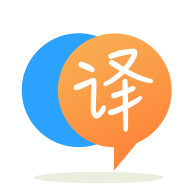
[英]Spring Boot - There was an unexpected error (type=Internal Server Error, status=500)
[英]Spring Boot Whitelabel Error page (type=Internal Server Error, status=500)
我正在嘗試遵循 spring 啟動的在線教程,我的所有 java 文件似乎都可以。 他們來了:
用戶.java文件
package net.javaguides.springboot.model;
import javax.persistence.Column;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.GenerationType;
import javax.persistence.Id;
import javax.persistence.Table;
@Entity
@Table(name = "users")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private long id;
@Column(name = "first_name")
private String firstName;
@Column(name = "last_name")
private String lastName;
private String email;
public User(String firstName, String lastName, String email) {
super();
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
}
public long getId() {
return id;
}
public void setId(long id) {
this.id = id;
}
public String getFirstName() {
return firstName;
}
public void setFirstName(String firstName) {
this.firstName = firstName;
}
public String getLastName() {
return lastName;
}
public void setLastName(String lastName) {
this.lastName = lastName;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}}
UserRepository.java 文件
package net.javaguides.springboot.repository;
import org.springframework.data.jpa.repository.JpaRepository;
import org.springframework.stereotype.Repository;
import net.javaguides.springboot.model.User;
@Repository
public interface UserRepository extends JpaRepository<User, Long>{
}
UserController.java 文件
package net.javaguides.springboot.controller;
import java.util.List;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import net.javaguides.springboot.model.User;
import net.javaguides.springboot.repository.UserRepository;
@RestController
@RequestMapping("api/")
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping("users")
public List<User> getUsers() {
return this.userRepository.findAll();
}}
最后一個是名為 Thinghiem1Application.java 的文件
package net.javaguides.springboot;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.CommandLineRunner;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import net.javaguides.springboot.model.User;
import net.javaguides.springboot.repository.UserRepository;
@SpringBootApplication
public class Thinghiem1Application implements CommandLineRunner{
public static void main(String[] args) {
SpringApplication.run(Thinghiem1Application.class, args);
}
@Autowired
private UserRepository userRepository;
@Override
public void run(String... args) throws Exception {
// TODO Auto-generated method stub
this.userRepository.save(new User("Duc", "Nguyen", "ramesh@gmail.com"));
this.userRepository.save(new User("Long", "Hoang", "tom@gmail.com"));
this.userRepository.save(new User("Tony", "Lan", "dir@gmail.com"));
}}
對不起我的錯誤,這是我的東西hiem1/pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0
https://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.5.2</version>
<relativePath/> <!-- lookup parent from repository -->
</parent>
<groupId>net.javaguides</groupId>
<artifactId>thinghiem1</artifactId>
<version>0.0.1-SNAPSHOT</version>
<name>thinghiem1</name>
<description>Demo project for Spring Boot</description>
<properties>
<java.version>11</java.version>
</properties>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
</project>
如您所見,沒有錯誤。
But when I ran the Thinghiem1Application.java file as Spring Boot App, it said to me that there was an error like this 2021-07-15 20:28:27.457 ERROR 11092 --- [nio-8080-exec-1] oac.c.C.[.[.[/].[dispatcherServlet]: Servlet.service() for servlet [dispatcherServlet] in context with path [] threw exception [Request processing failed; nested exception is org.springframework.orm.jpa.JpaSystemException: No default constructor for entity: : net.javaguides.springboot.model.User; nested exception is org.hibernate.InstantiationException: No default constructor for entity: : net.javaguides.springboot.model.User] with root cause
2021-07-15 20:28:27.457 ERROR 11092 --- [nio-8080-exec-1] oac.c.C.[.[.[/].[dispatcherServlet]: Servlet.service() for servlet [dispatcherServlet] in context with path [] threw exception [Request processing failed; nested exception is org.springframework.orm.jpa.JpaSystemException: No default constructor for entity: : net.javaguides.springboot.model.User; nested exception is org.hibernate.InstantiationException: No default constructor for entity: : net.javaguides.springboot.model.User] with root cause
並且頁面無法加載,它對我說Whitelabel Error Page This application has no explicit mapping for /error, so you are seeing this as a fallback.
我對這個問題感到非常困惑並試圖解決它但仍然無法解決它,你能給我一些想法嗎? 先感謝您。
您需要有一個默認構造函數,例如:
public User() {
}
Hibernate 使用默認構造函數來創建實體對象。 如果默認構造函數在任何實體中都不可用,則 InstantiationException: 出現意外錯誤 (type=Internal Server Error, status=500)。 將從休眠中拋出。
在您的用戶實體類中添加默認構造函數
您只需要向 User 類添加一個空構造函數:
公共用戶(){}
所有持久類都必須有一個默認構造函數,以便 Hibernate 可以使用 Constructor.newInstance() 實例化它們。 建議您在 Hibernate 中使用至少具有包可見性的默認構造函數來生成運行時代理。 所以你必須添加一個默認構造函數。
public User() {
}
Whitelabel 錯誤頁面 此應用程序沒有針對 /error 的顯式映射,因此您將其視為后備。
Tue Sep 27 17:27:29 WIB 2022 出現意外錯誤(類型=內部服務器錯誤,狀態=500)。 沒有可用的消息
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.