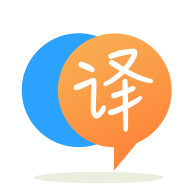
[英]How to use std::thread of C++ 11 under Cygwin GCC 4.7.2
[英]c++ : std::visit not compilable under gcc
這是我的代碼,它對 clang 編譯得很好,但在 gcc 時失敗了
#include <iostream>
#include <string>
#include <regex>
#include <variant>
struct Id {
void SetValue(const std::string& item)
{
value = item;
}
std::string value;
};
struct Number {
void SetValue(const std::string& item)
{
value = std::stoi(item);
}
int value;
};
using TokenBase
= std::variant<Number, Id>;
struct Token : TokenBase {
using TokenBase::TokenBase;
template <typename T>
[[nodiscard]] bool Is() const {
return std::holds_alternative<T>(*this);
}
template <typename T>
[[nodiscard]] const T& As() const {
return std::get<T>(*this);
}
template <typename T>
[[nodiscard]] const T* TryAs() const {
return std::get_if<T>(this);
}
};
struct LexerTokenExtractor {
const std::string& item_;
void operator()(Number& item) const {
item.SetValue(item_);
}
void operator()(Id& item) const {
item.SetValue(item_);
}
};
int main()
{
const std::string string_token("x");
Token id_token = Id();
std::visit(LexerTokenExtractor{string_token}, id_token);
std::cout << "ok" << std::endl;
}
這是日志:
required from here
/usr/include/c++/7/variant:97:29: error: incomplete type ‘std::variant_size<Token>’ used in nested name specifier
inline constexpr size_t variant_size_v = variant_size<_Variant>::value;
/usr/include/c++/7/variant: In instantiation of ‘constexpr const auto std::__detail::__variant::__gen_vtable<void, LexerTokenExtractor&&, Token&>::_S_vtable’:
/usr/include/c++/7/variant:711:29: required from ‘struct std::__detail::__variant::__gen_vtable<void, LexerTokenExtractor&&, Token&>’
/usr/include/c++/7/variant:1255:23: required from ‘constexpr decltype(auto) std::visit(_Visitor&&, _Variants&& ...) [with _Visitor = LexerTokenExtractor; _Variants = {Token&}]’
1673947047/source.cpp:65:57: required from here
/usr/include/c++/7/variant:711:49: error: ‘_S_apply’ was not declared in this scope
static constexpr auto _S_vtable = _S_apply();
請給我任何關於這里可能出錯的想法
正如評論中提到的,這是當前版本的 GCC 中的一個已知錯誤: https : //gcc.gnu.org/bugzilla/show_bug.cgi? id =90943
一個簡單的解決方法是強制std::visit()
直接對變體而不是子類進行操作。
std::visit(LexerTokenExtractor{string_token}, static_cast<TokenBase&>(id_token));
在 Godbolt 上查看: https ://gcc.godbolt.org/z/vMGfahq3z
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.