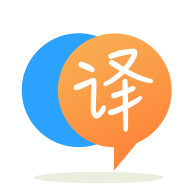
[英]How do you deallocate memory in java (using jna) from a C String returned from cgo?
[英]How to pass a Java string array []String to Go via cgo/JNA
我想通過 JNA 將 Java 中的 String[] 傳遞給我的 Go 函數。
我的 go 函數具有以下簽名:
func PredicateEval(keys, values []string, expression string) *C.char
我已將鏈接模式的 go 庫編譯為“c-shared”。 我在 Java 中有一個GoString定義為:
package predicates;
import com.ochafik.lang.jnaerator.runtime.NativeSize;
import com.sun.jna.Pointer;
import com.sun.jna.Structure;
import java.util.Arrays;
import java.util.List;
/**
* <i>native declaration : coverage_server/predicate_jvm_bridge/lib/libtest.h</i><br>
* This file was autogenerated by <a href="http://jnaerator.googlecode.com/">JNAerator</a>,<br>
* a tool written by <a href="http://ochafik.com/">Olivier Chafik</a> that <a href="http://code.google.com/p/jnaerator/wiki/CreditsAndLicense">uses a few opensource projects.</a>.<br>
* For help, please visit <a href="http://nativelibs4java.googlecode.com/">NativeLibs4Java</a> , <a href="http://rococoa.dev.java.net/">Rococoa</a>, or <a href="http://jna.dev.java.net/">JNA</a>.
*/
public class _GoString_ extends Structure {
/** C type : const char* */
public Pointer p;
public NativeSize n;
public _GoString_() {
super();
}
protected List<String> getFieldOrder() {
return Arrays.asList("p", "n");
}
/** @param p C type : const char* */
public _GoString_(Pointer p, NativeSize n) {
super();
this.p = p;
this.n = n;
}
public static class ByReference extends _GoString_ implements Structure.ByReference {
};
public static class ByValue extends _GoString_ implements Structure.ByValue {
};
}
我還有一個 Go 切片定義為:
package predicates;
import com.sun.jna.Pointer;
import com.sun.jna.Structure;
import java.util.Arrays;
import java.util.List;
/**
* <i>native declaration : coverage_server/predicate_jvm_bridge/lib/libtest.h</i><br>
* This file was autogenerated by <a href="http://jnaerator.googlecode.com/">JNAerator</a>,<br>
* a tool written by <a href="http://ochafik.com/">Olivier Chafik</a> that <a href="http://code.google.com/p/jnaerator/wiki/CreditsAndLicense">uses a few opensource projects.</a>.<br>
* For help, please visit <a href="http://nativelibs4java.googlecode.com/">NativeLibs4Java</a> , <a href="http://rococoa.dev.java.net/">Rococoa</a>, or <a href="http://jna.dev.java.net/">JNA</a>.
*/
public class GoSlice extends Structure {
/** C type : void* */
public Pointer data;
/** C type : GoInt */
public long len;
/** C type : GoInt */
public long cap;
public GoSlice() {
super();
}
protected List<String> getFieldOrder() {
return Arrays.asList("data", "len", "cap");
}
/**
* @param data C type : void*<br>
* @param len C type : GoInt<br>
* @param cap C type : GoInt
*/
public GoSlice(Pointer data, long len, long cap) {
super();
this.data = data;
this.len = len;
this.cap = cap;
}
public static class ByReference extends GoSlice implements Structure.ByReference {
};
public static class ByValue extends GoSlice implements Structure.ByValue {
};
}
這是我嘗試將 Java []String 轉換為 Go string[]。
static {
try {
Field field = sun.misc.Unsafe.class.getDeclaredField("theUnsafe");
field.setAccessible(true);
unsafe = (sun.misc.Unsafe) field.get(null);
Class<?> clazz = ByteBuffer.allocateDirect(0).getClass();
DIRECT_BYTE_BUFFER_ADDRESS_OFFSET = unsafe.objectFieldOffset(Buffer.class.getDeclaredField("address"));
DIRECT_BYTE_BUFFER_CLASS = clazz;
} catch (Exception e) {
throw new AssertionError(e);
}
}
private static long getAddress(ByteBuffer buffer) {
assert buffer.getClass() == DIRECT_BYTE_BUFFER_CLASS;
return unsafe.getLong(buffer, DIRECT_BYTE_BUFFER_ADDRESS_OFFSET);
}
public static _GoString_.ByValue JavaStringToGo(String jstr) {
try {
byte[] bytes = jstr.getBytes("utf-8");
//ByteBuffer bb = ByteBuffer.wrap(bytes);
ByteBuffer bb = ByteBuffer.allocateDirect(bytes.length);
bb.put(bytes);
Pointer p = new Pointer(getAddress(bb));
_GoString_.ByValue value = new _GoString_.ByValue();
value.n = new NativeSize(bytes.length);
value.p = p;
return value;
} catch (UnsupportedEncodingException e) {
throw new RuntimeException(e);
}
}
public static GoSlice.ByValue JavaStringArrayToGoStringSlice(String[] strings) {
_GoString_.ByValue[] goStrings = new _GoString_.ByValue[strings.length];
for (int i = 0; i < strings.length; i++) {
goStrings[i] = JavaStringToGo(strings[i]);
}
Memory arr = new Memory(strings.length * Native.getNativeSize(_GoString_.class));
for (int i = 0; i < strings.length; i++) {
System.out.println(Native.getNativeSize(_GoString_.class));
byte[] bytes = goStrings[0].getPointer().getByteArray(0, Native.getNativeSize(_GoString_.class));
arr.write(i*Native.getNativeSize(_GoString_.class), bytes, 0, bytes.length);
}
GoSlice.ByValue slice = new GoSlice.ByValue();
slice.data = arr;
slice.len = strings.length;
slice.cap = strings.length;
return slice;
}
一切都可以編譯,但是當我嘗試訪問 Go 端的切片元素時,出現了段錯誤:
unexpected fault address 0xb01dfacedebac1e
fatal error: fault
[signal SIGSEGV: segmentation violation code=0x1 addr=0xb01dfacedebac1e pc=0x10d7d3d6f]
goroutine 17 [running, locked to thread]:
您正在失去對實際字符串的內存分配的跟蹤(和控制)。
您對_GoString_
映射僅包括指針(4 或 8 字節)和NativeSize
(4 或 8 字節size_t
)的分配。 此映射假定Pointer
保持有效:
public class _GoString_ extends Structure {
/** C type : const char* */
public Pointer p;
public NativeSize n;
// constructors, etc.
}
但是,當您將值分配給p
您只會跟蹤指針的地址,而不是實際的內存分配(我已在代碼中添加了注釋):
public static _GoString_.ByValue JavaStringToGo(String jstr) {
try {
byte[] bytes = jstr.getBytes("utf-8");
//
// Here you allocate memory for the bytes
//
ByteBuffer bb = ByteBuffer.allocateDirect(bytes.length);
bb.put(bytes);
//
// Here you only keep track of the pointer to the bytes
//
Pointer p = new Pointer(getAddress(bb));
//
// You never reference bb again, it is no longer reachable
// and its allocation can be reclaimed by the system
//
_GoString_.ByValue value = new _GoString_.ByValue();
value.n = new NativeSize(bytes.length);
value.p = p;
return value;
} catch (UnsupportedEncodingException e) {
throw new RuntimeException(e);
}
}
作為直接字節緩沖區,內存位置和(解除)分配機制與普通對象和 GC 不同,但基本原則適用,一旦您失去對 Java 對象( ByteBuffer
)的引用,您將無法控制該本地對象何時內存將被釋放。 (當bb
被 GC 處理時,其內部字段包括在處理時將觸發釋放的引用。)
一種可能的解決方案是向您的_GoString_
類添加一個private
字段,該字段維護對ByteBuffer
的強引用並防止系統回收其內存(可能添加一個ByteBuffer
構造器)。
另一種解決方案是將 JNA 的Memory
類用於字符串,並將該Memory
對象(擴展Pointer
)直接存儲到p
字段。 我不確定您為什么為此應用程序選擇了直接字節緩沖區,因此這可能不適用於您的用例,但它肯定會簡化您的代碼。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.