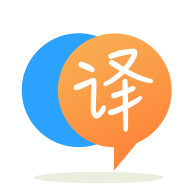
[英]How can I use the following code in an onclick event for a button other than a function()?
[英]How can I use a button onchange event to run the selected button's onclick function?
選擇HTML表單中的單選按鈕時,我希望它將運行我鏈接到它的onclick函數。 我之前嘗試使用 onclick 而不是 onchange ,但它不適用於單選按鈕。 有沒有人有任何建議/提示我可以用來解決這個問題?
function select(id, job, salary, someBool) { console.log(id, job, salary, someBool); }
<form class="choice"> <input type="radio" id="librarian" name="profession" value="lib" onclick="select('#professionSelect', 'Librarian', 4850, true);"> <label for="librarian">Librarian: $4,850/month</label><br> <input type="radio" id="mechanic" name="profession" value="mech" onclick="select('#professionSelect', 'Motorcycle Mechanic', 2800, true)"> <label for="mechanic">Motorcycle Mechanic: $2,800/month</label><br> <input type="radio" id="actor" name="profession" value="act" onclick="select('#professionSelect', 'Actor', 3100, true)"> <label for="actor">Actor: $3,100/month</label><br> <input type="radio" id="teacher" name="profession" value="tea" onclick="select('#professionSelect', 'Teacher', 4500, true)"> <label for="teacher">Teacher: $4,500/month</label><br> <input type="radio" id="doctor" name="profession" value="doc" onclick="select('#professionSelect', 'Doctor', 13000, true)"> <label for="doctor">Doctor: $13,000/month</label><br> <input type="radio" id="fire" name="profession" value="fi" onclick="select('#professionSelect', 'Firefighter', 3700, true)"> <label for="fire">Firefighter: $3,700/month</label><br> <input type="radio" id="engineer" name="profession" value="engi" onclick="select('#professionSelect', 'Computer Engineer', 9400, true)"> <label for="engineer">Computer Engineer: $9,400/month</label><br> </form>
這樣的東西會起作用嗎?
$(".choice").change(function() {
$(this).onclick();
});
您需要使用 name 屬性在每個單獨的 radio 元素上使用change
事件。 以下是如何執行此操作的基本示例:
$('input:radio[name=profession]').change(function(el) { select(this.getAttribute("id"), this.getAttribute("salary")); }); function select(profession, salary) { console.log(profession + ", " + salary) }
<script src="https://cdnjs.cloudflare.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script> <form class="choice"> <input type="radio" id="librarian" name="profession" value="lib" salary="4850"> <label for="librarian">Librarian: $4,850/month</label><br> <input type="radio" id="mechanic" name="profession" value="mech" salary="2800"> <label for="mechanic">Motorcycle Mechanic: $2,800/month</label><br> <input type="radio" id="actor" name="profession" value="act" salary="3100"> <label for="actor">Actor: $3,100/month</label><br> <input type="radio" id="teacher" name="profession" value="tea" salary="4500"> <label for="teacher">Teacher: $4,500/month</label><br> <input type="radio" id="doctor" name="profession" value="doc" salary="13000"> <label for="doctor">Doctor: $13,000/month</label><br> <input type="radio" id="fire" name="profession" value="fi" salary="3700"> <label for="fire">Firefighter: $3,700/month</label><br> <input type="radio" id="engineer" name="profession" value="engi" salary="9400"> <label for="engineer">Computer Engineer: $9,400/month</label><br> </form>
select
似乎是您不能在 HTML 內聯事件偵聽器中使用的詞。 使用任何其他有效的非保留關鍵字。
此外, onclick
和onchange
屬性都應該在這里工作。 這是一個使用它們的示例:
function boo(name,salary){ console.log(name,salary); } function body(name,salary){ console.log(name,salary); } function select(name,salary){ console.log(name,salary); }
<form class="choice"> <input type="radio" id="librarian" name="profession" value="lib" onchange="body('lib',4850)"> <label for="librarian">Librarian: $4,850/month</label><br> <input type="radio" id="mechanic" name="profession" value="mech" onchange="select('mechanic',2800)"> <label for="mechanic">Motorcycle Mechanic: $2,800/month</label><br> <input type="radio" id="actor" name="profession" value="act" onchange="boo('actor',3100)"> <label for="actor">Actor: $3,100/month</label><br> <input type="radio" id="teacher" name="profession" value="tea" onchange="boo('teacher',4500)"> <label for="teacher">Teacher: $4,500/month</label><br> <input type="radio" id="doctor" name="profession" value="doc" onclick="boo('doctor',13000)"> <label for="doctor">Doctor: $13,000/month</label><br> </form>
在上面的例子中,我同時使用了select
和body
,它們都不是 JS 中的關鍵字。 但不能在內聯事件處理程序中工作。
您可以直接在 JS 中使用它們,如下所示:
let select = function(){ console.log(event.target.value); } let body = function(){ console.log(event.target.value); } document.querySelector('#librarian').onchange = select; document.querySelector('#librarian2').onchange = body;
<input type="radio" id="librarian" name="profession" value="lib" /> <label for="librarian">Librarian: $4,850/month</label> <input type="radio" id="librarian2" name="profession" value="lib2" /> <label for="librarian2">Librarian2: $4,850/month</label>
也許,為什么文檔建議不要使用內聯事件處理程序。
將 JS 和 HTML 分開。
我剛剛發現您基本上是在嘗試從 HTML 本身傳遞數據。
因此,最好的方法是使用data-
attribute 屬性來執行此操作並在其上設置您的值並在 Javascript 中處理其余部分,而不是自己編寫單擊事件。
const professions = document.querySelectorAll("input[name='profession']"); function select(id, job, salary, someBool) { console.log(id, job, salary, someBool); } professions.forEach((profession)=>{ profession.addEventListener('click',(e)=>{ const professionData = profession.getAttribute('data-profession'); const dataArray = professionData.split(","); select(profession.id,dataArray[1],dataArray[2],dataArray[3]); }) });
<form class="choice"> <input type="radio" id="librarian" name="profession" value="lib" data-profession="'#professionSelect', 'Librarian', 4850, true"> <label for="librarian">Librarian: $4,850/month</label><br> <input type="radio" id="mechanic" name="profession" value="mech" data-profession="'#professionSelect', 'Motorcycle Mechanic', 2800, true"> <label for="mechanic">Motorcycle Mechanic: $2,800/month</label><br> <input type="radio" id="actor" name="profession" value="act" data-profession = "'#professionSelect', 'Actor', 3100, true"> <label for="actor">Actor: $3,100/month</label><br> </form>
試試這個代碼。 要了解有關數據屬性的更多信息,您可以使用此 w3school 資源。
一種方法:
const myForm = document.forms['choice'] , data = { lib : { id: '#professionSelect', job: 'Librarian', salary: 4850, someBool: true } , mech : { id: '#professionSelect', job: 'Motorcycle Mechanic', salary: 2800, someBool: true } , act : { id: '#professionSelect', job: 'Actor', salary: 3100, someBool: true } , tea : { id: '#professionSelect', job: 'Teacher', salary: 500, someBool: true } , doc : { id: '#professionSelect', job: 'Doctor', salary: 13000, someBool: true } , fi : { id: '#professionSelect', job: 'Firefighter', salary: 3700, someBool: true } , engi : { id: '#professionSelect', job: 'Computer Engineer', salary: 9400, someBool: true } } myForm.oninput = e => { console.clear() console.log( data[myForm.profession.value] ) }
label { display : block; float : left; clear : left; margin : .3em 1em; }
<form name="choice" > <label> <input type="radio" name="profession" value="lib" > Librarian: $4,850/month </label> <label> <input type="radio" name="profession" value="mech" > Motorcycle Mechanic: $2,800/month </label> <label> <input type="radio" name="profession" value="act" > Actor: $3,100/month </label> <label> <input type="radio" name="profession" value="tea" > Teacher: $4,500/month </label> <label> <input type="radio" name="profession" value="doc" > Doctor: $13,000/month </label> <label> <input type="radio" name="profession" value="fi" > Firefighter: $3,700/month </label> <label> <input type="radio" name="profession" value="engi" > Computer Engineer: $9,400/month </label> </form>
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.