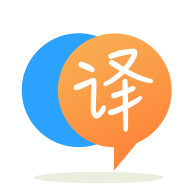
[英]Encountered a problemin my first node.js code andi can't understand what went wrong
[英]Can't understand what's went wrong
我寫了這段代碼作為工廠模式的練習,但出現錯誤,我不知道為什么。
代碼:
class ShopFactory {
constructor (item, type, name) {
let shelf;
if(type === "toiletries"){
shelf = new Toiletries(item, name);
}else if (type === "cosmetics") {
shelf = new Cosmetics(item, name);
}else if (type === "foods") {
shelf = new Foods(item, name);
}else if (type === "beautifications"){
shelf = new Beautifications(item, name);
}
shelf.type = type;
shelf.func = function () {
console.log(`${this.item}--${this.type}--${this.name} Cost: ${this.cost}`);
}
return shelf;
}
}
class Toiletries{
constructor (item, name){
this.item = item;
this.name = name;
this.cost = "$15";
}
}
class Cosmetics{
constructor (item, name){
this.item = item;
this.name = name;
this.cost = "$30";
}
}
class Foods{
constructor (item, name){
this.item = item;
this.name = name;
this.cost = "$20";
}
}
class Beautifications{
constructor (item, name){
this.item = item;
this.name = name;
this.cost = "$25";
}
}
var shelves = [];
shelves.push(new ShopFactory("grocery","foods","rice"));
shelves.push(new ShopFactory("cleaning","toiletries","toilet-cleaner"));
shelves.push(new ShopFactory("machine-tools","beautifications","screw-driver"));
shelves.push(new ShopFactory("powder","Cosmetics","body-powder"));
shelves.push(new ShopFactory("cleaning","beautifications","wand"));
shelves.push(new ShopFactory("grocery","foods","fish"));
console.log(shelves);
Error:
Uncaught TypeError: Cannot set property 'type' of undefined
at ShopFactory.createShelf (app2.js:18)
at app2.js:60
createShelf @ app2.js:18
(anonymous) @ app2.js:60
問題是:如果您傳遞給它一個無效type
,例如"Cosmetics"
,工廠會怎么做? 請記住,上限很重要。 您應該拋出一個適當的信息錯誤,否則將不會設置shelf
並且您正在嘗試執行(undefined).type = type;
:
class ShopFactory {
constructor(item, type, name) {
let shelf;
if (type === "toiletries") {
shelf = new Toiletries(item, name);
} else if (type === "cosmetics") {
shelf = new Cosmetics(item, name);
} else if (type === "foods") {
shelf = new Foods(item, name);
} else if (type === "beautifications") {
shelf = new Beautifications(item, name);
} else {
throw new Error(`Unknown type '${type}'`);
}
shelf.type = type;
shelf.func = function () {
console.log(`${this.item}--${this.type}--${this.name} Cost: ${this.cost}`);
}
return shelf;
}
}
架子應該是對象,因為您要在其上附加type
。
class ShopFactory {
constructor (item, type, name) {
let shelf = {};
if(type === "toiletries"){
shelf = new Toiletries(item, name);
}else if (type === "cosmetics") {
shelf = new Cosmetics(item, name);
}else if (type === "foods") {
shelf = new Foods(item, name);
}else if (type === "beautifications"){
shelf = new Beautifications(item, name);
}
shelf.type = type;
shelf.func = function () {
console.log(`${this.item}--${this.type}--${this.name} Cost: ${this.cost}`);
}
return shelf;
}
}
當您執行此行時
shelves.push(new ShopFactory("powder","Cosmetics","body-powder"));
您的構造函數的以下子句失敗(因為條件“化妝品”不匹配),因此變量shelf
仍然未定義。 然后您分配了shelf.type
但由於變量shelf
仍未定義(不是對象),您不能為其分配屬性。 這就是您收到錯誤的原因。
if(type === "toiletries"){
shelf = new Toiletries(item, name);
}else if (type === "cosmetics") {
shelf = new Cosmetics(item, name);
}else if (type === "foods") {
shelf = new Foods(item, name);
}else if (type === "beautifications"){
shelf = new Beautifications(item, name);
}
一種快速的解決方案是將變量shelf
聲明為一個對象,如let shelf = {};
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.