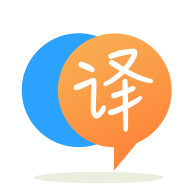
[英]Accessing nested object values inside an array when using map to iterate over the array of objects
[英]using map to iterate over an object that is inside of an array
這是我的代碼:
class SomeClass{
constructor(props){
super(props);
this.state = {
SomeElement:
[
{
AAAQQQ: "",
AAA: ""
},
],
errorMessage: ""
};
}
MyFunction = (someParam) =>{
//do something
}
}
我可以通過執行以下操作訪問 errorMessage:
this.state.errorMessage
但是對於訪問AAAQQQ,我說:
this.state.SomeElement.map((item, index) =>{
SomeFunction(item.AAAQQQ);
});
我的問題:
上述方法是否正確訪問對象數組中的元素?
item.AAAQQQ
部分是。 如果您不使用數組map
創建,則map
部分不是。 我已經寫了為什么在這里以及該怎么做,但基本上使用for-of
:
for (const item of this.state.SomeElement) {
SomeFunction(item.AAAQQQ);
}
或forEach
:
this.state.SomeElement.forEach(item => {
SomeFunction(item.AAAQQQ);
});
當您需要索引以及項目( .forEach((item, index) => { /*...*/ })
)時, forEach
非常方便。
我將列出訪問元素的各種方法。 在此之前,我想告訴您什么時候可以使用哪些javascript函數。
像 map 和 filter 這樣的數組函數將返回一個數組作為輸出。 因此,如果您想對每個數組元素執行一些操作並將最終結果存儲在數組中,那么我們可以使用 map 和 filter 方法。
當您想保存數組中所有元素的結果時可以使用映射,如果只想保存某些元素,則可以使用過濾器。
一個簡單的例子在這里
const arr = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10];
const allSquare = arr.map(element => (element * element));
const evenElements = arr.filter(element => {
if(arr%2 == 0) {
return true;
}
return false;
});
// more concise way to write
const evenElements = arr.filter(element => !(element%2));
基本上在過濾器中,每當您返回 true 時,元素將被保存,否則將被跳過。
因此,這里要注意的一點是,只要您想要另一個數組作為輸出,就可以使用映射和過濾器。 否則,您還有其他迭代方式,例如 for、forEach。
for基本上是我們在所有編程語言中使用的普通 for 循環, forEach接受回調函數,因此您可以在那里執行操作。
在您的情況下,您不想為 someFunctions 存儲任何輸出,因此您可以使用 forEach,這是訪問元素的最佳方式。
如果您只有一個對象,那么您可以直接使用圓括號索引訪問方法。 this.state.someElement[0].AAAQQQ
否則,如果您有對象數組,那么 forEach 是最好的方法,因為您不必像這樣手動編寫
for(let i = 0; i<this.state.someElement.length; i++) {
// do something
}
你可以直接這樣做
this.state.someElement.forEach((ele, index) => {
// do something
})
如果你有這樣的對象
const people = {
person1: { name: 'John', age: 10 },
person2: { name: 'Ajay', age: 12 },
person3: { name: 'Rocky', age: 15 }
}
然后你可以像這樣訪問元素。
const peopleKeys = Object.keys(people);
const names = peopleKeys.map((key) => {
return people[key].name;
})
console.log(names) // ['John', 'Ajay', 'Rocky']
請注意,這里我們想要每個元素的輸出,因此我們使用了 map。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.