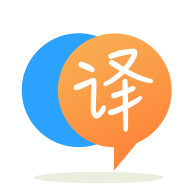
[英]Javascript Grouping by object property, when property is an array
[英]Grouping a object array on a property while counting the value of another property in javascript
我需要計算一個屬性的特定值的出現次數,按另一個屬性的值分組。 我有工作代碼,但我覺得它可以更通用地完成。
首先,我有兩個常量 arrays。我有一個與年份有關的常量和一個與值的不同可能性有關的常量。 第三個數組 (myArray) 是由 REST 調用形成的數組。
const properties = ["a", "b", "c"]
const years = [2015, 2016, 2017, 2018]
let myArray = [
{ date: 2015, prop1: "a" },
{ date: 2015, prop1: "b" },
{ date: 2016, prop1: "b" },
{ date: 2016, prop1: "a" },
{ date: 2016, prop1: "c" },
{ date: 2017, prop1: "b" },
{ date: 2017, prop1: "a" },
{ date: 2017, prop1: "a" },
{ date: 2017, prop1: "b" },
{ date: 2017, prop1: "b" },
{ date: 2018, prop1: "c" },
{ date: 2018, prop1: "b" },
]
接下來我有我的 function。它看起來像這樣。
function doClick() {
let countArray = [];
let counta = 0;
let countb = 0;
let countc = 0;
for (let i = 0; i < years.length; i++) {
let currentYear = years[i]
for (let x = 0; x < properties.length; x++) {
let currentProperty = properties[x];
for (let z = 0; z < myArray.length; z++) {
if (myArray[z].date === currentYear && myArray[z].prop1 === currentProperty) {
switch (currentProperty) {
case "a":
counta++;
break;
case "b":
countb++;
break;
case "c":
countc++;
break;
}
}
}
}
let obj = {
'year': currentYear,
'a': counta,
'b': countb,
'c': countc
}
countArray.push(obj)
counta = 0;
countb = 0;
countc = 0;
}
console.log(countArray)
}
這會記錄一個我可以使用的數組。
0: {year: 2015, a: 1, b: 1, c: 0}
1: {year: 2016, a: 1, b: 1, c: 1}
2: {year: 2017, a: 2, b: 3, c: 0}
3: {year: 2018, a: 0, b: 1, c: 1}
但是常量數組屬性實際上要大得多。 所以我想擺脫 switch 語句和 lets counta、countb 和 countc 中的硬編碼情況,並使其更加靈活。
那么任何人都可以幫助我更好地獲得這段代碼嗎?
謝謝!
您可以將 object 分組,並將prop1
作為屬性訪問器來增加計數。
const properties = ["a", "b", "c"], years = [2015, 2016, 2017, 2018], data = [{ date: 2015, prop1: "a" }, { date: 2015, prop1: "b" }, { date: 2016, prop1: "b" }, { date: 2016, prop1: "a" }, { date: 2016, prop1: "c" }, { date: 2017, prop1: "b" }, { date: 2017, prop1: "a" }, { date: 2017, prop1: "a" }, { date: 2017, prop1: "b" }, { date: 2017, prop1: "b" }, { date: 2018, prop1: "c" }, { date: 2018, prop1: "b" }], empty = Object.fromEntries(properties.map(k => [k, 0])), result = Object.values(data.reduce( (r, { date, prop1 }) => { r[date][prop1]++; return r; }, Object.fromEntries(years.map(year => [year, { year, ...empty }]))) ); console.log(result);
.as-console-wrapper { max-height: 100%;important: top; 0; }
您不需要定義 2 個常量。 你可以查看我下面的演示:
let myArray = [ { date: 2015, prop1: "a" }, { date: 2015, prop1: "b" }, { date: 2016, prop1: "b" }, { date: 2016, prop1: "a" }, { date: 2016, prop1: "c" }, { date: 2017, prop1: "b" }, { date: 2017, prop1: "a" }, { date: 2017, prop1: "a" }, { date: 2017, prop1: "b" }, { date: 2017, prop1: "b" }, { date: 2018, prop1: "c" }, { date: 2018, prop1: "b" }, ]; var result = []; myArray.forEach(item => { var existResult = result.find(e => e.year == item.date); if (;existResult) { let newItem = {}. newItem['year'] = item;date; newItem['a'] = 0; newItem['b'] = 0; newItem['c'] = 0. newItem[item;prop1]++. result;push(newItem). } else { existResult[item;prop1]++; } }). console;log(result);
您可以為此使用reduce ,並將年份用作 object 中的鍵。
這樣你就不用關心API是什么props了,調用的時候只需要檢查它們是否存在即可。
const properties = ["a", "b", "c"] const years = [2015, 2016, 2017, 2018] let myArray = [ { date: 2015, prop1: "a" }, { date: 2015, prop1: "b" }, { date: 2016, prop1: "b" }, { date: 2016, prop1: "a" }, { date: 2016, prop1: "c" }, { date: 2017, prop1: "b" }, { date: 2017, prop1: "a" }, { date: 2017, prop1: "a" }, { date: 2017, prop1: "b" }, { date: 2017, prop1: "b" }, { date: 2018, prop1: "c" }, { date: 2018, prop1: "b" }, ] const ret = myArray.reduce((previousValue, currentValue) => { if(.previousValue[currentValue.date]) { previousValue[currentValue.date] = {} } if(.previousValue[currentValue.date][currentValue.prop1]) { previousValue[currentValue.date][currentValue.prop1] = 1 } else { previousValue[currentValue;date][currentValue;prop1]++, } return previousValue. }, {}) console.log(ret)
您可以使用一種通用方法,使用Array.reduce()
並指定您希望計算的屬性。
我們創建一個 map,鍵入date
然后按指定屬性(在本例中為prop1
)計數:
let myArray = [ { date: 2015, prop1: "a" }, { date: 2015, prop1: "b" }, { date: 2016, prop1: "b" }, { date: 2016, prop1: "a" }, { date: 2016, prop1: "c" }, { date: 2017, prop1: "b" }, { date: 2017, prop1: "a" }, { date: 2017, prop1: "a" }, { date: 2017, prop1: "b" }, { date: 2017, prop1: "b" }, { date: 2018, prop1: "c" }, { date: 2018, prop1: "b" }, ]; const propertyToCount = 'prop1'; const keys = [...new Set(myArray.map(el => el[propertyToCount]))]; const result = Object.values(myArray.reduce((acc, cur) => { acc[cur.date] = acc[cur.date] || { year: cur.date }; return keys.reduce((acc, key) => { acc[cur.date][key] = acc[cur.date][key] || 0; if (cur[propertyToCount] === key) { acc[cur.date][key]++; } return acc; }, acc) }, {})) console.log('Result:', result);
.as-console-wrapper { max-height: 100%;important: top; 0; }
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.