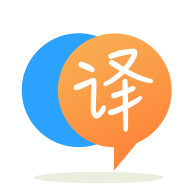
[英]Facebook Messenger Instant Games cannot fetch from or post to leaderboards
[英]How to use Facebook Instant Games Leaderboards and In-App Ads with LibGDX
我想讓我的 LibGDX 游戲作為 Facebook 即時游戲運行。 到目前為止,我已經成功地設置了游戲客戶端,如快速入門指南所示:
<!DOCTYPE html>
<html>
<head>
<title>My LibGDX Game</title>
<meta http-equiv="content-type" content="text/html; charset=UTF-8" />
<meta
id="gameViewport"
name="viewport"
content="width=device-width initial-scale=1"
/>
<meta name="apple-mobile-web-app-capable" content="yes" />
<meta name="full-screen" content="yes" />
<meta name="screen-orientation" content="portrait" />
</head>
<body>
<div align="center" id="embed-html"></div>
<script src="https://connect.facebook.net/en_US/fbinstant.6.3.js"></script>
<script src="main.js"></script>
<script type="text/javascript" src="html/html.nocache.js"></script>
</body>
<script>
function handleMouseDown(evt) {
evt.preventDefault();
evt.stopPropagation();
window.focus();
}
function handleMouseUp(evt) {
evt.preventDefault();
evt.stopPropagation();
}
document
.getElementById("embed-html")
.addEventListener("mousedown", handleMouseDown, false);
document
.getElementById("embed-html")
.addEventListener("mouseup", handleMouseUp, false);
</script>
</html>
在與 GWT 生成的 JS 代碼分開的main.js
文件中,我可以使用 Facebook Instant games SDK 獲取用戶名和 ID,如下所示:
FBInstant.initializeAsync().then(function () {
var progress = 0;
var interval = setInterval(function () {
progress += 3;
FBInstant.setLoadingProgress(progress);
if (progress >= 99) {
clearInterval(interval);
FBInstant.startGameAsync().then(function () {
console.log("shit stsrted");
var playerId = FBInstant.player.getID(); // Get Facebook ID
var playerName = FBInstant.player.getName(); // Get Facebook Name
console.log(playerId);
console.log(playerName);
});
}
}, 100);
console.log("loaded");
});
現在我需要在 GameOver 屏幕上顯示一個廣告和排行榜,但是如果代碼是在 JavaScript 中,我如何添加排行榜和應用內廣告? 我試圖深入研究 GWT 編譯的 JS 代碼以某種方式找到 GameOver 函數,但該代碼不可讀。 有沒有辦法在 Java 代碼中做到這一點,也許注入 JavaScript 並讓 GWT 用 Java 編譯它?
GWT 項目支持從 Java 調用原生 JS,以前通過 JSNI 允許你編寫 JavaScript 作為 Java 世界中方法的注釋,但現在有一個更好的解決方案 - JSInterop - http://www.gwtproject.org/doc/latest /DevGuideCodingBasicsJsInterop.html
我在自己學習時遇到了你的問題,這個博客很好地解釋了我的用例: https : //lofidewanto.medium.com/learn-once-use-everywhere-javascript-in-java-for-web-瀏覽器-ec1b9657232c
這就是我所做的:Libgdx 是多平台的,我想保留將我的游戲移植到移動平台的能力,所以我創建了一個 Java 界面來處理廣告(插頁式廣告和獎勵視頻)的加載和顯示:
public interface AdRequestHandler {
void loadInterstitial();
void showInterstitial();
void loadRewardedVideo();
void showRewardedVideo();
}
我的 GWT/HTML 僅針對 Facebook 即時游戲,因此我提供了上述接口的實現:
public class FacebookAdRequestHandler implements AdRequestHandler {
private FacebookAdRequestService facebookAdRequestService = new FacebookAdRequestService();
@Override
public void loadInterstitial() {
facebookAdRequestService .loadInterstitialImpl();
}
@Override
public void showInterstitial() {
facebookAdRequestHandlerNative.showInterstitialImpl();
}
@Override
public void loadRewardedVideo() {
facebookAdRequestService.loadRewardedVideoImpl();
}
@Override
public void showRewardedVideo() {
facebookAdRequestService.showRewardedVideoImpl();
}
}
該實現簡單地調用了一個執行所有原生 JS 調用的 Service 類:
@JsType(namespace = JsPackage.GLOBAL, name = "FacebookAdRequestHandler", isNative = true)
public class FacebookAdRequestService {
public native void loadInterstitialImpl();
public native void showInterstitialImpl();
public native void loadRewardedVideoImpl();
public native void showRewardedVideoImpl();
}
現在對於 JS 部分,我將您的 main.js 文件放在html/webapp
下的項目中
FBInstant.initializeAsync().then(function () {
var progress = 0;
var interval = setInterval(function () {
progress += 3;
FBInstant.setLoadingProgress(progress);
if (progress >= 99) {
clearInterval(interval);
FBInstant.startGameAsync().then(function () {
//var playerId = FBInstant.player.getID(); // Get Facebook ID
//var playerName = FBInstant.player.getName(); // Get Facebook Name
});
}
}, 100);
});
FacebookAdRequestHandler = function () {
var preloadedInterstitial = null;
var preloadedRewardedVideo = null;
}
FacebookAdRequestHandler.prototype.loadInterstitialImpl = function () {
FBInstant.getInterstitialAdAsync(
'123123123123_123123123123' // Your Ad Placement Id
).then(function(interstitial) {
// Load the Ad asynchronously
preloadedInterstitial = interstitial;
return preloadedInterstitial.loadAsync();
}).then(function() {
console.log('Interstitial preloaded');
}).catch(function(err){
console.error('Interstitial failed to preload: ' + err.message);
});
}
FacebookAdRequestHandler.prototype.showInterstitialImpl = function () {
preloadedInterstitial.showAsync()
.then(function() {
// Perform post-ad success operation
console.log('Interstitial ad finished successfully');
})
.catch(function(e) {
console.error(e.message);
});
}
FacebookAdRequestHandler.prototype.loadRewardedVideoImpl = function () {
FBInstant.getRewardedVideoAsync(
'456456456456_456456456456' // Your Ad Placement Id
).then(function(rewarded) {
// Load the Ad asynchronously
preloadedRewardedVideo = rewarded;
return preloadedRewardedVideo.loadAsync();
}).then(function() {
console.log('Rewarded video preloaded');
}).catch(function(err){
console.error('Rewarded video failed to preload: ' + err.message);
});
}
FacebookAdRequestHandler.prototype.showRewardedVideoImpl = function () {
preloadedRewardedVideo.showAsync()
.then(function() {
// Perform post-ad success operation
console.log('Rewarded video watched successfully');
})
.catch(function(e) {
console.error(e.message);
});
}
我已經直接從 FB 的小游戲文檔 - 小游戲的應用內廣告中獲取了用於加載和顯示插頁式廣告的代碼,而且我實際上已經以您的示例來解決初始化和繞過進度條報告的問題。
到目前為止,我已經對其插頁式廣告進行了測試,並且可以正確顯示廣告。 需要做的是確保只在加載時顯示廣告(也許只是在showInterstitialImpl
做一個空檢查。現在這只是一個片面的解決方案,它不會向 Java 部分報告任何顯示的內容,你可以也可以使用 @JsFunction 注釋來創建一些回調
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.