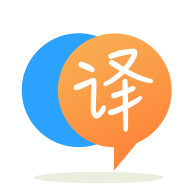
[英]Using map with reduce in javascript to filter objects in an array
[英]Using Javascript Map and Reduce to manipulate Array of Objects
我已經這樣做了:
import React from 'react';
const Header =(props)=>{
const courseRecord = props.course.map(line => line)
return(
<>
<h2> {courseRecord[0].name }</h2>
<h2> {courseRecord[1].name }</h2>
</>
)
}
const Part =(props) =>{
return(
<>
<p>{props.partName} {props.noOfEx}</p>
</>
)
}
const Content =(props) =>{
return(
<div>
{props.course
.map(kourse => kourse['parts']
.map(parti =><Part key={parti['id']} partName = {parti['name']} noOfEx = {parti['exercises']}/> )
)}
</div>
)
}
const Total =(props) =>{
// const numbers = props.course.parts;
const numbers = props.course.map(kourse => kourse['parts']);
var exTotal = numbers.reduce((totalExercises,currentValue) =>{
console.log("totalExercises " , totalExercises , " current value " ,currentValue, " Exercises ", currentValue[0][1])
return totalExercises * currentValue.exercises
},0 );
return(
<>
<p><b>Total of {exTotal} exercises</b></p>
</>
)}
const Course = (props) =>{
let records =props.course.length;
return(
<>
<h1>Web Development Curriculumn</h1>
<Header course={props.course} />
<Content course ={props.course}/>
<Total course ={props.course}/>
</>
)
}
const App = () => {
const course =[
{
id: 1,
name:'Half Stack application development',
parts: [
{
name: 'Fundamentals of React',
exercises: 10,
id: 1
},
{
name: 'Using props to pass data',
exercises: 7,
id: 2
},
{
name: 'State of a component',
exercises: 14,
id: 3
},
{
name: 'Destructuring',
exercises: 14,
id: 4
}
]
},
{
name :'Node JS',
id: 2,
parts:[
{
name: 'Routing',
exercises: 3,
id: 1
},
{
name: 'middlewares',
exercises: 7,
id: 2
}
]
}
]
return <Course course={course} />
}
export default App;
我希望以這種方式格式化輸出:
Web Development curriculum
Half Stack Application Development
Fundamentals of React 10
Using props to pass Data 7
State of Component 14
Destructuring 14
Total of 45 exercises
Node Js
Routing 3
Middlewares 7
total of 10 exercises
我得到這個:
Web Development Curriculumn
Half Stack application development
Node JS
Fundamentals of React 10
Using props to pass data 7
State of a component 14
Destructuring 14
Routing 3
middlewares 7
Total of NaN exercises
我該如何解決?
當你使用數組時,請使用復數,所以數組的課程應該是courses
而不是course
,只是循環似乎不正確,除此之外你的代碼看起來很好。 請檢查下面的示例代碼。
import React from 'react';
const Header = ({ course }) => {
return (
<>
<h2> {course.name}</h2>
</>
);
};
const Part = (props) => {
return (
<>
<p>
{props.partName} {props.noOfEx}
</p>
</>
);
};
const Content = ({ course }) => {
return (
<div>
{course['parts'].map((parti) => (
<Part
key={parti['id']}
partName={parti['name']}
noOfEx={parti['exercises']}
/>
))}
</div>
);
};
const Total = ({ course }) => {
// const numbers = props.course.parts;
var exTotal = course['parts'].reduce((totalExercises, currentValue) => {
console.log(
'totalExercises ',
totalExercises,
' current value ',
currentValue,
' Exercises ',
currentValue
);
totalExercises = totalExercises + currentValue.exercises;
return totalExercises;
}, 0);
return (
<>
<p>
<b>Total of {exTotal} exercises</b>
</p>
</>
);
};
const Course = ({ courses }) => {
return (
<>
<h1>Web Development Curriculumn</h1>
{courses && courses.length
? courses.map((course, index) => (
<div key={index}>
<Header course={course} />
<Content course={course} />
<Total course={course} />
</div>
))
: null}
</>
);
};
const App = () => {
const courses = [
{
id: 1,
name: 'Half Stack application development',
parts: [
{
name: 'Fundamentals of React',
exercises: 10,
id: 1,
},
{
name: 'Using props to pass data',
exercises: 7,
id: 2,
},
{
name: 'State of a component',
exercises: 14,
id: 3,
},
{
name: 'Destructuring',
exercises: 14,
id: 4,
},
],
},
{
name: 'Node JS',
id: 2,
parts: [
{
name: 'Routing',
exercises: 3,
id: 1,
},
{
name: 'middlewares',
exercises: 7,
id: 2,
},
],
},
];
return <Course courses={courses} />;
};
export default App;
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.