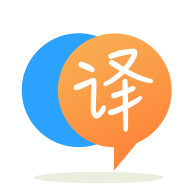
[英]How can I return a value while checking if a Java 8 Optional is present?
[英]How do I return the value of the node before an item is present?
我正在為我的數據結構類開發一個項目,該項目要求我編寫一個類來實現一個整數鏈表。
- 為節點使用內部類。
- 包括以下方法。
- 編寫一個測試器,使您能夠以任何順序使用您想要的任何數據測試所有方法。
我必須創建一個名為“私有節點 getPreviousNode”的方法。 此方法旨在“返回剛好位於項目之前的節點的值,如果不存在則返回 null”。 我在下面有這個方法的代碼。 但是,當我測試這個方法時,我得到了錯誤的輸出。 它應該返回項目之前的節點的值,如果不存在則返回 null。 因此,例如,如果我有一個像“9 10 2 5 16 18 17 1 2 19”這樣的列表,並且我想獲取 16 的前一個節點,則該方法應返回 5。但相反,它返回“LinkedListOfInts$Node@5b464ce8”有人知道我做錯了什么嗎? 以及如何解決?
import java.util.Random;
import java.util.Scanner;
public class LinkedListOfIntsTest {
Node head;
private class Node {
int value;
Node nextNode;
public Node(int value, Node nextNode) {
this.value = value;
this.nextNode = nextNode;
}
}
public LinkedListOfIntsTest(LinkedListOfIntsTest other) {
Node tail = null;
for (Node n = other.head; n != null; n = n.nextNode) {
if (tail == null)
this.head = tail = new Node(n.value, null);
else {
tail.nextNode = new Node(n.value, null);
tail = tail.nextNode;
}
}
}
public LinkedListOfIntsTest(int[] other) {
Node[] nodes = new Node[other.length];
for (int index = 0; index < other.length; index++) {
nodes[index] = new Node(other[index], null);
if (index > 0) {
nodes[index - 1].nextNode = nodes[index];
}
}
head = nodes[0];
}
public LinkedListOfIntsTest(int N, int low, int high) {
Random random = new Random();
for (int i = 0; i < N; i++)
this.addToFront(random.nextInt(high - low) + low);
}
public void addToFront(int x) {
head = new Node(x, head);
}
private Node getPreviousNode(int item) {
Node previous = null;
for (Node ptr = head; ptr != null; ptr = ptr.nextNode) {
if (ptr.value == item)
return previous;
previous = ptr;
}
return null;
}
public String toString() {
String result = " ";
for (Node ptr = head; ptr != null; ptr = ptr.nextNode)
result += ptr.value + " ";
return result;
}
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
LinkedListOfIntsTest list = new LinkedListOfIntsTest(10, 1, 20);
LinkedListOfIntsTest copy = new LinkedListOfIntsTest(list);
boolean done = false;
while (!done) {
System.out.println("1. Get Previous Node");
System.out.println("2. toString");
switch (input.nextInt()) {
case 1:
System.out.println("Get Previous Node");
System.out.println(list.getPreviousNode(input.nextInt()));
break;
case 2:
System.out.println("toString");
System.out.println(list.toString());
break;
}
}
}
}
此方法旨在“返回剛好位於項目之前的節點的值,如果不存在則返回 null”。 我在下面有這個方法的代碼
由於該方法必須返回節點的值,因此不應返回節點實例本身,而是返回其value
成員。
由於您的節點值是int
s,選擇它作為函數的返回類型似乎是合適的,但由於您還必須預見一個null
返回值,返回類型應該是Integer
。
最后,該函數還有一個問題:當用一個根本沒有出現在列表中的值調用search
時,它返回列表的尾節點。 同樣在這種情況下,它應該返回null
。
這是一個可能的更正:
private Integer getPreviousNode(int item) {
if (head != null)
for (Node previous = head; previous.nextNode != null; previous = previous.nextNode)
if (previous.nextNode.value == item)
return previous.value;
return null;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.