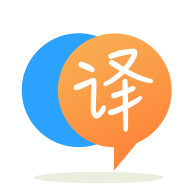
[英]My toString method using a loop shows an output with a missing element, how do I fix it?
[英]How can I fix my output in my toString method?
我正在為我的數據結構類開發一個項目,該項目要求我編寫一個類來實現一個整數鏈表。
- 為節點使用內部類。
- 包括以下方法。
- 編寫一個測試器,使您能夠以任何順序使用您想要的任何數據測試所有方法。
我必須創建一個名為“public String toString()”的 toString 方法。 此方法旨在“打印列表,但格式為 [1, 2, 3],逗號和括號位於正確的位置。” 我在下面有這個方法的代碼。 但是,當我使用 toString 方法時,會出現一些問題。 例如,如果我有一個列表 [17, 14, 8, 11, 19, 6, 15, 11, 5, 5]。 我的輸出是 [17, 14, 8, 11, 19, 6, 15, 11, 5, 5, ]。 在最后一個元素之后,我得到一個額外的逗號和一個額外的空格。 我該如何解決?
import java.util.Random;
import java.util.Scanner;
public class LinkedListOfInts {
Node head;
Node tail;
private class Node {
int value;
Node nextNode;
public Node(int value, Node nextNode) {
this.value = value;
this.nextNode = nextNode;
}
}
public LinkedListOfInts(LinkedListOfInts other) {
Node tail = null;
for (Node n = other.head; n != null; n = n.nextNode) {
if (tail == null)
this.head = tail = new Node(n.value, null);
else {
tail.nextNode = new Node(n.value, null);
tail = tail.nextNode;
}
}
}
public LinkedListOfInts(int[] other) {
Node[] nodes = new Node[other.length];
for (int index = 0; index < other.length; index++) {
nodes[index] = new Node(other[index], null);
if (index > 0) {
nodes[index - 1].nextNode = nodes[index];
}
}
head = nodes[0];
}
public LinkedListOfInts(int N, int low, int high) {
Random random = new Random();
for (int i = 0; i < N; i++)
this.addToFront(random.nextInt(high - low) + low);
}
public void addToFront(int x) {
head = new Node(x, head);
}
public String toString() {
String result = "";
for (Node ptr = head; ptr != null; ptr = ptr.nextNode)
result += ptr.value + ", ";
return "[" + result + "]";
}
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
LinkedListOfInts list = new LinkedListOfInts(10, 1, 20);
boolean done = false;
while (!done) {
System.out.println("1. toString");
switch (input.nextInt()) {
case 1:
System.out.println("toString");
System.out.println(list.toString());
break;
}
}
}
}
使用StringJoiner
,例如...
public String toString() {
StringJoiner sj = new StringJoiner(", ", "[", "]");;
for (Node ptr = head; ptr != null; ptr = ptr.nextNode) {
sj.add(Integer.toString(ptr.value));
}
return sj.toString();
}
然后使用類似...
LinkedListOfInts list = new LinkedListOfInts(new int[]{17, 14, 8, 11, 19, 6, 15, 11, 5, 5});
System.out.println(list.toString());
會打印...
[17, 14, 8, 11, 19, 6, 15, 11, 5, 5]
現在,如果您不能使用StringJoiner
🙄,請不要附加,
而是在它StringJoiner
加上前綴,例如...
public String toString() {
String result = "";
for (Node ptr = head; ptr != null; ptr = ptr.nextNode) {
if (!result.isEmpty()) {
result += ", ";
}
result += ptr.value;
}
return "[" + result + "]";
}
我們可以討論使用StringBuilder
的重要性以及所有這些,但您明白了
跟蹤循環外的節點並通過檢查下一個節點是否為空來迭代到倒數第二個節點。 然后在 for 循環之后追加最后一個節點的值。
IE
public String toString() {
String result = "";
Node ptr;
for (ptr = head; ptr.nextNode != null; ptr = ptr.nextNode) {
result += ptr.value + ", ";
result += ptr.value + "]";
result = "[" + result;
return result;
嘗試這個。
public String toString() {
return Stream.iterate(head, Objects::nonNull, Node::nextNode)
.map(n -> String.valueOf(n.value))
.collect(Collectors.joining(", ", "[", "]"));
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.