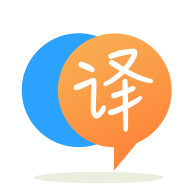
[英]How to pass multiple query parameters in @Query in Spring Data JPA?
[英]How to have filtering of multiple parameters passed as RequestParams in Spring Data Jpa?
假設我有一個 Employee 類,其中包含多個字段,如姓名、年齡、薪水、職位、加入日期、性別等等。 現在,我如何使用許多這樣的參數應用過濾? 在這種情況下可能有多種組合。 (例如,如果我想要 6 個過濾器,那么總共可以有 6 個!= 720 種可能的組合!!!)
僅適用於 2,3 個參數,如年齡、薪水、姓名; 那么我可以寫多個 if 案例:
if(age!=null && name==null && salary==null)
{
findByAge
}
if(age==null && name!=null && salary==null)
{
findByName
}
if(age!=null && name!=null && salary==null)
{
findByAgeAndName
}
等等,像這些在 Spring Data JPA 的幫助下。 但是如何處理更多的參數,因為每個 RequestParams 的組合都會增加?
您正在尋找的是多條件查詢。 您可以使用非常簡單的示例 API
在你的情況下,代碼看起來像這樣:
您將使用構建器模式創建一個實體。 Lombok 可以幫助您完成這一步:
@Data
@Builder
@NoArgsConstructor
@AllArgsConstructor
@Entity
@Table(name = "employee")
public class Employee {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(name = "name")
private String name;
@Column(name = "age")
private int age;
// your other fields
}
然后你可以調用你的方法:
Employee employee = Employee
.builder()
.name(nameValue)
.age(ageValue)
.build();
return repository.findAll(Example.of(employee));
你會 在這里找到一個完整的例子。
你不應該寫很多條件和/或調用函數。 許多條件很難結合起來。
在這種情況下,我經常編寫查詢,如果參數不為空或指定,則該查詢將附加條件。
這是我的方法:
String query = "select * from table where 1=1";
Map<String,Object> params = new HashMap<>();
if(filter1!=null){
query += " and field1 = :filter1";
params.put("filter1",filter1);
}
if(filter2!=null){
query += " and field2 = :filter2";
params.put("filter2",filter2);
}
dao.execute(query,params);
條件 null 不是必需的,您可以對其進行調整。 如果你不想在你的函數中有很多參數。 您可以創建對象/上下文/地圖來保存所有過濾器。
PS:我使用 String 來提高可讀性,你應該使用 StringBuilder。
對於 spring-jpa:ExampleMatcher 可以做同樣的事情。
https://docs.spring.io/spring-data/jpa/docs/current/reference/html/#query-by-example.matchers
一種解決方案是使用單一方法,並通過管理查詢中的空值使參數“可選”:
@Query("""
select a from YourEntity a where
(?1 is null or a.name = ?1)
and (?2 is null or a.age= ?2)
and (?3 is null or a.salary = ?3)
and (?4 is null or a.description = ?4)
and (?5 is null or a.joiningDate = ?5)
and (?6 is null or a.gender = ?6)
""")
List<YourEntity> findFiltered(String name, Integer age, Float salary, String designation, LocalDateTime joiningDate, String gender)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.