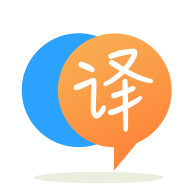
[英]how can i test browser back-button behaviour using jest and (react-) testing-library?
[英]How can I assert which value has been passed to a <li> key attribute in react with jest and testing-library/react
我正在使用 react+ts 和 jest+testing-library 進行測試。 我想對以下代碼進行單元測試,並希望確保將person.id設置為li元素的key屬性。 最初我以為我可以通過運行expect(listItems[1]).toHaveAttribute('key', '2');
來斷言它expect(listItems[1]).toHaveAttribute('key', '2');
但似乎 key 屬性已從最終的 HTML 中刪除,這似乎是我正在測試的內容。 如何確保將 person.id 傳遞給列表項的 key 屬性?
import React from 'react';
import Api from '../control/api';
const PersonList = () => {
const persons = Api.getPersons();
return (
<ul>
{persons.map((person) => {
return (
<li key={person.id}>
{`${person.id}: ${person.name.familyName}, ${person.name.givenName}`}
</li>
);
})}
</ul>
);
};
export default PersonList;
考試:
import React from 'react';
import { render, screen } from '@testing-library/react';
import PersonList from '../../components/PersonList';
import { getPersons } from '../../control/api';
jest.mock('../../control/api');
const mockGetPersons = getPersons as jest.MockedFunction<typeof getPersons>;
describe('The PersonList component', () => {
it('should output empty list if there are no persons returned by the api', () => {
mockGetPersons.mockReturnValue([]);
render(<PersonList />);
const lists = screen.getAllByRole('list');
expect(lists).toHaveLength(1);
});
it('should render one person returned by api', () => {
mockGetPersons.mockReturnValue([
{
id: '1',
name: {
familyName: 'Duck',
givenName: 'Donald',
},
},
]);
render(<PersonList />);
const listItems = screen.getAllByRole('listitem');
expect(listItems).toHaveLength(1);
expect(listItems[0]).toHaveTextContent('1: Duck, Donald');
});
it('should render several persons returned by api', () => {
mockGetPersons.mockReturnValue([
{
id: '1',
name: {
familyName: 'Duck',
givenName: 'Donald',
},
},
{
id: '2',
name: {
familyName: 'Duck',
givenName: 'Dagobert',
},
},
{
id: '3',
name: {
familyName: 'Mouse',
givenName: 'Mickey',
},
},
]);
render(<PersonList />);
const listItems = screen.getAllByRole('listitem');
expect(listItems).toHaveLength(3);
expect(listItems[0]).toHaveTextContent('1: Duck, Donald');
// expect(listItems[1]).toHaveAttribute('key', '2');
expect(listItems[2]).toHaveTextContent('3: Mouse, Mickey');
});
});
key 屬性由 react 在內部使用,它被設置為什么並不重要,只要當前列表中的每個項目的值都是唯一的。 因此,代碼的用戶(最終用戶或開發人員)都不會使用/查看/知道那里設置的值,這意味着我們不應該編寫測試來斷言 key 屬性。
有關react 文檔中關鍵屬性的更多信息
關於測試實施細節的博客文章
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.