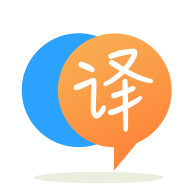
[英]Python - how to match specific words / digits from multiple lines in a text file and store them in separate lists
[英]How to extract from text digits and calculate them in a specific order?
我正在從要分析的網站上抓取數據。 在關於工作經驗的部分中,我提取了指定某人在公司工作多長時間的文本 - 該信息如下所示:
雇佣期 \\n2 年 2 月
分析以月表示的就業時間會更容易。 現在我想知道如何從文本中提取這些信息並正確計算。 給定示例的計算應為:
2 x 12 + 2
我嘗試這樣做:
def text_format(text: str):
digits = []
text = text.replace('\\n', ' ')
text = text.replace('.', '')
text = text.split()
for word in text:
if word.isalpha():
pass
else:
word = int(word)
digits.append(word)
total = digits[0] * 12 + digits[1]
return total
在這種特殊情況下,上面的功能運行良好,但我可能有其他情況,例如
聘期\\n3年
或者
雇佣期 11 周一
我不知道如何處理所有可能的情況。
>>> str_1 = "Employment period \n2years 2 mon."
>>> str_2 = "Employment period \n3years"
>>> str_3 = "Employment period 11 mon."
>>> def func(x):
... return (
... eval(x.strip("Employment period ")
... .strip()
... .replace("years", "* 12 +")
... .replace("mon.", "")
... .strip()
... .rstrip("+")
... ))
>>> func(str_1)
26
>>> func(str_2)
36
>>> func(str_3)
11
您可以使用正則表達式來解決這個問題並涵蓋所有可能的場景。 例如,像下面這樣的應該使這項任務更容易:
Employment period(?:[ \\n]+(\d+)[ ]*years?)?(?:[ \\n]+(\d+)[ ]*mon\.)?
您也可以在Regex Demo上嘗試一下。
這是一個 Python 示例,它貫穿了提到的特定用例,以及我添加的一些其他邊緣情況:
import re
pattern = re.compile(r'Employment period(?:[ \\n]+(\d+)[ ]*years?)?(?:[ \\n]+(\d+)[ ]*mon\.)?')
string = r"""\
Employment period \n2years 2 mon.
Employment period \n3years
Employment period 11 mon.
Employment period 010 years
Employment period 1 year
Employment period
testing\
"""
for x in pattern.finditer(string):
print('Found a match:', x.group(0))
years, months = x.groups()
if years or months:
total_months = int(years or 0) * 12 + int(months or 0)
print(f'Total months: {total_months}')
輸出:
Found a match: Employment period \n2years 2 mon.
Total months: 26
Found a match: Employment period \n3years
Total months: 36
Found a match: Employment period 11 mon.
Total months: 11
Found a match: Employment period 010 years
Total months: 120
Found a match: Employment period 1 year
Total months: 12
Found a match: Employment period
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.