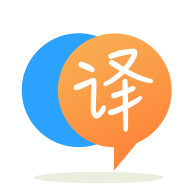
[英]how do you write a program for function named makeWordLengthDict which takes a LIST of words as its only parameter, and returns a dictionary in python
[英]how do I write a program that takes a list of strings as input and returns a dictionary, containing an index of the words to the matching strings
規則:在字典中,每個鍵將是一個單詞 k,而值將是單詞 k 出現的輸入字符串的索引列表。
單詞應僅被視為小寫。 即 Hello 和 hello 應該被同等對待。
可以假設數據集將只包含字符串列表。 無需檢查數據集中元素的類型。
數據集中的字符串數據將是干凈的。 無需擔心清潔,即去除標點符號或數字。
在下面的示例中,該函數確定給定數據集中單詞的索引是什么。 dataset 是包含字符串的列表。
reverse_index 函數應該創建並返回字典。
dataset = [
"Hello world",
"This is the WORLD",
"hello again"
]
res = reverse_index(dataset)
# This assertion checks if the result equals the expected dictinary
assert(res == {
'hello': [0, 2],
'world': [0, 1],
'this': [1],
'is': [1],
'the': [1],
'again':[2]
})
我不確定接下來要做什么,但這就是我開始的方式
dataset = [
"Hello world",
"This is the WORLD",
"hello again"
]
def reverse_index(dataset):
你可以試試這個方法
def reverse_index(data):
res = dict()
for i in range(len(data)):
for word in map(str.lower,data[i].split()):
if word not in res:
res[word] = [i,]
else:
res[word].append(i)
return res
輸出:
{
'hello': [0, 2],
'world': [0, 1],
'this': [1],
'is': [1],
'the': [1],
'again':[2]
}
您可以使用collections.defaultdict
作為基礎和一個小循環:
from collections import defaultdict
res = defaultdict(list)
for i,s in enumerate(dataset):
for w in set(map(str.lower, s.split())):
res[w].append(i)
dict(res)
輸出:
{'hello': [0, 2],
'world': [0, 1],
'is': [1],
'the': [1],
'this': [1],
'again': [2]}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.