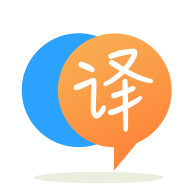
[英]What wrong am I doing in the below code? When I run this program I am getting error
[英]I am getting an Attribute error message when I want to make my sprite character shoot bullets. What am I doing wrong?
我能夠加載我的 pygame 窗口,但是當我按下空格按鈕讓我的精靈角色射擊時,它會退出 pygame 窗口並給我一個屬性錯誤。 我究竟做錯了什么? 我該如何解決這個問題??? 我已經嘗試過 from bullet import Bullet 但這不起作用。 請幫我修復這個錯誤,這樣我就可以繼續編碼......非常感謝你的幫助。
autopilot.py 代碼:
import pygame
import car
import debris
pygame.init()
#screen settings
WIDTH = 1000
HEIGHT = 400
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("AutoPilot")
screen.fill((255, 255, 255))
#fps
FPS = 120
clock = pygame.time.Clock()
#load images
bg = pygame.image.load('background/street.png').convert_alpha() # background
#define game variables
######################CAR/DEBRIS##########################
player = car.Player(1, 5)
debris = debris.Debris(1, 5)
##########################################################
#groups
bullet_group = pygame.sprite.Group()
debris_group = pygame.sprite.Group()
debris_group.add(debris)
#game runs here
run = True
while run:
#draw street
screen.blit(bg, [0, 0])
#update groups
bullet_group.update()
bullet_group.draw(screen)
#draw debris
debris.draw()
#draw car
player.draw()
player.move()
for event in pygame.event.get():
if event.type == pygame.QUIT:
run = False
#check if key is down
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_ESCAPE:
run = False
if event.key == pygame.K_a:
player.movingLeft = True
if event.key == pygame.K_d:
player.movingRight = True
if event.key == pygame.K_SPACE:
player.shoot()
#check if key is up
if event.type == pygame.KEYUP:
if event.key == pygame.K_a:
player.movingLeft = False
if event.key == pygame.K_d:
player.movingRight = False
#update the display
pygame.display.update()
pygame.display.flip()
clock.tick(FPS)
pygame.quit()
car.py 代碼:
import pygame
#screen settings
WIDTH = 1000
HEIGHT = 400
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("AutoPilot")
screen.fill((255, 255, 255))
#load bullets
bullets = pygame.image.load('car/bullet.png').convert_alpha()
#groups
bullet_group = pygame.sprite.Group()
#player class
class Player(pygame.sprite.Sprite):
def __init__(self, scale, speed):
pygame.sprite.Sprite.__init__(self)
self.speed = speed
#self.x = x
#self.y = y
self.moving = True
self.frame = 0
self.flip = False
self.direction = 0
#load car
self.images = []
img = pygame.image.load('car/car.png').convert_alpha()
img = pygame.transform.scale(img, (int(img.get_width()) * scale, (int(img.get_height()) * scale)))
self.images.append(img)
self.image = self.images[0]
self.rect = self.image.get_rect()
self.update_time = pygame.time.get_ticks()
self.movingLeft = False
self.movingRight = False
self.rect.x = 465
self.rect.y = 325
#draw car to screen
def draw(self):
screen.blit(self.image, (self.rect.centerx, self.rect.centery))
#move car
def move(self):
#reset the movement variables
dx = 0
dy = 0
#moving variables
if self.movingLeft and self.rect.x > 33:
dx -= self.speed
self.flip = True
self.direction = -1
if self.movingRight and self.rect.x < 900:
dx += self.speed
self.flip = False
self.direction = 1
#update rectangle position
self.rect.x += dx
self.rect.y += dy
#shoot
def shoot(self):
bullet = bullets.Bullet(self.rect.centerx + 18, self.rect.y + 30, self.direction)
bullet_group.add(bullet)
子彈.py 代碼:
import pygame
from autopilot import debris, bullet_group
#screen settings
WIDTH = 1000
HEIGHT = 400
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("AutoPilot")
screen.fill((255, 255, 255))
#load bullets
bullets = pygame.image.load('car/bullet.png').convert_alpha()
#bullet class
class Bullet(pygame.sprite.Sprite):
def __init__(self, x, y, direction):
pygame.sprite.Sprite.__init__(self)
self.speed = 5
self.image = bullets
self.rect = self.image.get_rect()
self.rect.center = (x,y)
self.direction = direction
def update(self):
self.rect.centery -= self.speed
#check if bullet has gone off screen
if self.rect.top < 1:
self.kill()
#check collision with cement block
if pygame.sprite.spritecollide(debris, bullet_group, False):
if debris.alive:
debris.health -= 25
碎片.py代碼:
import pygame
#screen settings
WIDTH = 1000
HEIGHT = 400
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("AutoPilot")
screen.fill((255, 255, 255))
#debris class
class Debris(pygame.sprite.Sprite):
def __init__(self, scale, speed):
pygame.sprite.Sprite.__init__(self)
self.x = 400
self.y = HEIGHT / 2 - 200
self.speed = speed
self.vy = 0
self.on_ground = True
self.move = True
self.health = 100
self.max_health = self.health
self.alive = True
#load debris
self.images = []
img = pygame.image.load('debris/cement.png').convert_alpha()
img = pygame.transform.scale(img, (int(img.get_width()) * scale, (int(img.get_height()) * scale)))
self.images.append(img)
self.image = self.images[0]
self.rect = self.image.get_rect()
#draw debris to screen
def draw(self):
screen.blit(self.image,(self.x,self.y))
我的錯誤:
您想創建Bullet
類的一個實例。 因此,您必須導入Bullet
:
from bullet import Bullet
最后,您可以使用Bullet
類:
class Player(pygame.sprite.Sprite):
# [...]
def shoot(self):
bullet = Bullet(self.rect.centerx + 18, self.rect.y + 30, self.direction)
bullet_group.add(bullet)
如果只想導入bullet.py文件,則必須重命名bullet
變量。 否則會在變量bullet
和模塊bullet
之間出現名稱沖突:
import bullet
class Player(pygame.sprite.Sprite):
# [...]
def shoot(self):
bullet_object = bullet.Bullet(self.rect.centerx + 18, self.rect.y + 30, self.direction)
bullet_group.add(bullet_object)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.