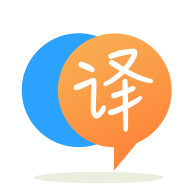
[英]How to use TypeScript with Vue.js and Single File Components?
[英]How to link a shared store with multiple single file components in Vue.js 3?
我有一個 Vue.js 3 應用程序。 此應用程序包含多個單文件組件。 我試圖弄清楚如何實現最簡單的全局狀態管理。 這些文檔對我來說很有意義。 但是,有限的文檔顯示了從多個組件的data
函數返回的reactive
對象。 這個例子假設reactive
對象和組件都在同一個文件中。 我遇到的挑戰是在多個文件中使用共享存儲。 目前我有:
這些文件如下所示:
應用程序
<template>
<div>
<div>How much money do you have?</div>
<input type="number" v-model="currentAmount" />
<br />
<div>How much money do you want?</div>
<input type="number" v-model="desiredAmount" />
</div>
</template>
<script>
import ComponentA from './component-a.vue';
import ComponentB from './component-b.vue';
export default {
components: {
ComponentA,
ComponentB
},
data() {
return {
currentAmount: 0,
desiredAmount: 0
}
},
}
</script>
組件-a.vue
<template>
<div>
<div>You have {{ remainingAmount }} to reach your goal.</div>
</div>
</template>
<script>
export default {
data() {
return {
remainingAmount: 0
}
},
}
</script>
組件-b.vue
<template>
<div>
<div>You are {{ progress }}% of the way there!</div>
<button @click="increaseGoal">increase goal by $10</button>
</div>
</template>
<script>
export default {
data() {
return {
progress: 0
}
},
methods: {
increaseGoal() {
// need to increase targetAmount by $10
store.targetAmount += 10;
}
}
}
</script>
商店.js
import { reactive} from 'vue';
const store = reactive({
startingAmount: 0,
targetAmount: 0
});
用戶界面呈現。 但是,很明顯,數據並沒有在組件之間傳播。 我可以清楚地看到 store 和三個 .vue 文件之間沒有鏈接。 沒有任何東西可以將商店與 UI 聯系起來。 我不知道如何讓store.js
與三個.vue文件,即使正確關聯。
您如何將共享存儲與多個單文件組件鏈接起來? 請注意:我不想用Vuex。
要在全局級別上獲取商店,您可以使用 vue use()
它,以便您能夠從 VueApp 中的任何組件調用this.$store
主文件
import { createApp } from 'vue';
import store from './store/index'; // OR the path to your
import router from './router/index';
import App from './App.vue';
createApp(App).use(router).use(store).mount('#app');
我創建了一個商店文件夾,因為我在多個模塊中使用了商店。 store 文件夾中是index.js
,其中包含基本存儲和所有模塊。
商店/index.js
import { createStore } from 'vuex';
const store = createStore({
modules: {},
state() {
return {};
},
mutations: {},
actions: {},
getters: {},
});
export default store;
通過這樣做,您可以從任何組件調用商店。
組件.vue
export default {
name: 'users-list',
created() {
console.log(this.$store);
},
}
不應直接更改商店狀態中的變量。
這家商店有3種程序。
行動- 做精心設計的東西
操作用於完成給定數據更改的繁重工作。 在這里,您可以從不同的商店模塊交叉饋送功能,進行 axios 調用等。 因此,Actions 是唯一可以訪問存儲本身的過程組。 第一個參數基本上是整個商店。 動作是通過調用dispatch('NameOfModule/NameOfAction', ValuetoPass)
觸發的。
突變- 心愛的 Setter 函數
Mutations 用於改變狀態,並且只有 Mutations 應該改變狀態以保持反應性和工作流干凈。 因此,Mutations 只會將它們自己的本地狀態作為第一個參數傳遞。 突變將由commit('NameOfMutation', ValueToPass)
觸發
Getters - 不言自明,不是嗎?
吸氣劑習慣於,是的,獲取狀態。
正如您所說,您不需要使用 Vuex 來創建全局狀態。 多虧了 composition-api 我們可以使用可組合物。
首先,創建目錄/composables並添加javascript文件(創建以use word開頭的文件是一個好習慣)useState.js:
import { reactive, toRefs } from "vue";
const state = reactive({
isMenuOpened: false
});
const toggleMenuState = () => {
state.isMenuOpened = !state.isMenuOpened;
};
export default {
...toRefs(state),
toggleMenuState
};
toRefs 將所有屬性轉換為具有 refs 屬性的普通對象
現在你可以在 vue 組件中使用可組合了:
<script>
import useState from "./composables/useState";
export default {
setup() {
const { isMenuOpened, toggleMenuState } = useState;
return {
isMenuOpened,
toggleMenuState,
};
},
};
</script>
演示: https : //codesandbox.io/s/happy-chandrasekhar-o05uv?file=/ src/ App.vue
關於組合 api 和可組合: https : //v3.vuejs.org/guide/composition-api-introduction.html
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.