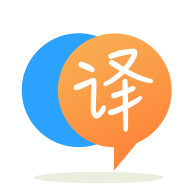
[英]Check if any value in any 2 arrays with different dimension is same in php
[英]PHP check if certain value in different arrays are the same for all
我有幾個這樣的數組
$arrayOne = array (
"name" => 'john',
"position" => 'instructor',
"hired" => '2010',
"department" => 'math',
);
$arrayTwo = array (
"name" => 'smith',
"position" => 'instructor',
"hired" => '2010',
"department" => 'math',
);
$arrayThree = array (
"name" => 'dave',
"position" => 'instructor',
"hired" => '2009',
"department" => 'math',
);
如何檢查這些陣列是否都具有相同的雇用日期?
一種方法是比較每個人:
if($arrayOne['hired'] === $arrayTwo['hired']) & if($arrayOne['hired'] === $arrayThree['hired']) & ...
但是有沒有更干凈的方法來做到這一點?
你最初的問題和隨后的評論是相似的,但他們試圖用數據做不同的事情,結果不同。 最初被問到的是:
我如何檢查這些數組是否都具有相同的雇用日期
這可以通過以下方式完成:
var_dump(count(array_unique(array_column([$arrayOne, $arrayTwo, $arrayThree], 'hired'))));
// or
$combined = [$arrayOne, $arrayTwo, $arrayThree];
$hiredValues = array_column($combined, 'hired');
$hiredValuesUnique = array_unique($hiredValues);
$length = count($hiredValuesUnique);
var_dump($length);
如果count
為1
,則它們相同,否則它們不同。
但是,您的后續評論是
我怎么知道哪些是相同的
為此,我將創建一個由該值鍵控的新數組,並在源數組上foreach
,有效地將相似的數組分組以便您進一步操作。
$final = [];
foreach([$arrayOne, $arrayTwo, $arrayThree] as $array){
if(!array_key_exists($array['hired'], $final)){
$final[$array['hired']] = [];
}
$final[$array['hired']][] = $array;
}
var_dump($final);
其中產生:
array(2) {
[2010]=>
array(2) {
[0]=>
array(4) {
["name"]=>
string(4) "john"
["position"]=>
string(10) "instructor"
["hired"]=>
string(4) "2010"
["department"]=>
string(4) "math"
}
[1]=>
array(4) {
["name"]=>
string(5) "smith"
["position"]=>
string(10) "instructor"
["hired"]=>
string(4) "2010"
["department"]=>
string(4) "math"
}
}
[2009]=>
array(1) {
[0]=>
array(4) {
["name"]=>
string(4) "dave"
["position"]=>
string(10) "instructor"
["hired"]=>
string(4) "2009"
["department"]=>
string(4) "math"
}
}
}
我寫了下面的代碼:
//Your data
$arrayOne = array (
"name" => 'john',
"position" => 'instructor',
"hired" => '2010',
"department" => 'math',
);
$arrayTwo = array (
"name" => 'smith',
"position" => 'instructor',
"hired" => '2010',
"department" => 'math',
);
$arrayThree = array (
"name" => 'dave',
"position" => 'instructor',
"hired" => '2009',
"department" => 'math',
);
function hiredIsTheSameEverywhere(...$arrays) : bool
{
return count(array_count_values(array_column($arrays, "hired"))) === 1;
}
function whereHiredIsTheSame(...$arrays) : array
{
$return = [];
$count = array_count_values(array_column($arrays, "hired"));
foreach($arrays as $array) {
if($count[$array['hired']] > 1) {
$return[$array['hired']][] = $array;
}
}
return $return;
}
//The output
var_dump(hiredIsTheSameEverywhere($arrayOne, $arrayTwo, $arrayThree));
var_dump(whereHiredIsTheSame($arrayOne, $arrayTwo, $arrayThree));
輸出:
bool(false)
array(1) {
[2010]=>
array(2) {
[0]=>
array(4) {
["name"]=>
string(4) "john"
["position"]=>
string(10) "instructor"
["hired"]=>
string(4) "2010"
["department"]=>
string(4) "math"
}
[1]=>
array(4) {
["name"]=>
string(5) "smith"
["position"]=>
string(10) "instructor"
["hired"]=>
string(4) "2010"
["department"]=>
string(4) "math"
}
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.