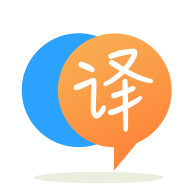
[英]how do i send a print('somethin') in node red using python function node?
[英]How do i print 2 consecutive red or green candles using the def function and conditional statements in python
我希望 python 條件語句只執行/打印“請出售”,如果連續有 2 個連續的紅色蠟燭,然后是連續的 2 個連續的綠色蠟燭
對於 ELIF,如果連續有 2 個連續的 GREEN CANDLES,然后是連續的 2 個連續的 RED CANDLES。
我的以下代碼僅在 1 根紅色蠟燭和 1 根綠色蠟燭上打印和執行。 請讓我知道如何改進代碼以給出我預期的結果
def data_color(open,close):
color = []
if open > close:
color.append("RED")
elif open < close:
color.append("GREEN")
else:
color.append("DOJI")
return color
while True:
time_iq = API.get_server_timestamp()
if int(dt.fromtimestamp(time_iq).second) == 59 or 1 > 0:
data_candle = API.get_candles(pair,timeframe,10,time_iq)
colors = data_color(data_candle[-2]["open"], data_candle[-2]["close"])
if colors[0] == "RED":
print(" PLEASE SELL")
elif colors[0] == "GREEN":
print("PLEASE BUY")
for i in range (1,len(colors)):
if colors[i-1] and colors[i] == "RED":
print(" PLEASE SELL")
elif colors[i-1] and colors[i] == "GREEN":
print("PLEASE BUY")
else:
pass
你應該試試這個
有很多方法可以解決這個問題,但這里有兩種方法可以滿足您的需求:
這取決於您想如何評估和找到您正在尋找的模式。 從您的問題來看,目前尚不清楚您擁有的數據是否來自 4 個條目的預格式化“數據包”,您在其中尋找“2 綠 2 紅”或“2 紅 2 綠”的識別模式,因此我將假設第一個示例不是這種情況。 在第二個例子中,我將說明如何處理事情,如果這個假設是真實的。
示例 1:迭代顏色列表並在最后 4 個條目中找到您要查找的圖案,包括當前迭代。
def evaluate_by_iteration(_colors):
# -- skip the first three entries
for i in range(4, len(_colors)):
# -- get the preceding three elements and the current one.
# -- note the "i+1" here, this is how list slicing works
first, second, third, fourth = _colors[i-3: i+1]
if (first == second == 'GREEN') and (third == fourth == 'RED'):
print('[iterator] PLEASE SELL')
elif (first == second == 'RED') and (third == fourth == 'GREEN'):
print('[iterator] PLEASE BUY')
示例 2:將列表分成大小為 4 的數據包,並檢查每個數據包是否符合模式。 僅當數據預計以這種方式分塊時才這樣做! (同樣,從你的問題中不清楚)
from itertools import izip_longest
def evaluate_by_chunks(_colors):
chunked_colors = izip_longest(*[iter(_colors)] * 4, fillvalue=None)
for chunk in list(chunked_colors):
first, second, third, fourth = chunk
if (first == second == 'GREEN') and (third == fourth == 'RED'):
print('[chunker] PLEASE SELL')
if (first == second == 'RED') and (third == fourth == 'GREEN'):
print('[chunker] PLEASE BUY')
為什么要使用第二種方法而不是第一種方法?
這取決於您想如何評估數據。 取以下數據集:
colors = ['RED', 'GREEN', 'RED', 'RED', 'GREEN', 'GREEN', 'RED', 'RED']
print('-------------')
print('EVALUATE BY ITERATION')
evaluate_by_iteration(colors)
print(' ')
print('-------------')
print('EVALUATE BY CHUNKS')
evaluate_by_chunks(colors)
print(' ')
這將打印:
-------------
EVALUATE BY ITERATION
[iterator] PLEASE BUY
[iterator] PLEASE SELL
-------------
EVALUATE BY CHUNKS
[chunker] PLEASE SELL
請注意“通過迭代評估”如何有兩個匹配項,因為我們每次只將搜索索引增加一個。
現在,根據您的需要,其中之一可能是正確的; 使用基於迭代器的方法,您將找到您要查找的模式的每個實例,包括重疊的模式,例如,您有一個“GREEN GREEN RED RED GREEN GREEN”模式。
使用基於塊的方法,您可以確保永遠不會評估重疊的模式,但前提是假設您的數據很好地組織在大小為 4 的數據包中。
有一種最終方法采用迭代器方法,但確保不會對任何項進行兩次評估; 在這個例子中,我們重寫了基於迭代的評估器方法,但不是回顧,而是向前看。 這允許我們在找到模式匹配時手動增加“索引”變量,確保我們在匹配時跳過模式。
這允許數據相對非結構化,同時確保您不會評估重疊模式。
def evaluate_by_iteration_looking_forward(_colors):
# -- skip the first three entries
counter = 0
for i in range(0, len(_colors) - 3):
if counter > len(_colors) - 3:
break
first, second, third, fourth = _colors[counter: counter + 4]
if (first == second == 'GREEN') and (third == fourth == 'RED'):
print('[iterator] PLEASE SELL')
counter += 4
continue
elif (first == second == 'RED') and (third == fourth == 'GREEN'):
print('[iterator] PLEASE BUY')
counter += 4
continue
counter += 1
為了測試這一點,我們運行以下代碼:
print('-------------')
print('EVALUATE BY ITERATION')
evaluate_by_iteration(colors)
print(' ')
print('-------------')
print('EVALUATE BY CHUNKS')
evaluate_by_chunks(colors)
print(' ')
print('-------------')
print('EVALUATE BY ITERATION LOOKING FORWARD')
evaluate_by_iteration_looking_forward(colors)
哪個打印:
-------------
EVALUATE BY ITERATION
[iterator] PLEASE BUY
[iterator] PLEASE SELL
-------------
EVALUATE BY CHUNKS
[chunker] PLEASE SELL
-------------
EVALUATE BY ITERATION LOOKING FORWARD
[iterator] PLEASE BUY
如您所見,我們的前瞻性評估器只匹配第一個模式,然后跳過 head,確保它不會與之前已經評估過的元素第二次匹配。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.