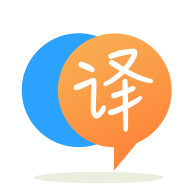
[英]How to get the specific token balance available at a give ETH address using web3.py
[英]Compute the LP Address of a token pair using web3.py
經過幾個小時的搜索,我設法運行了這段代碼,但不幸的是,這並沒有產生我想要的輸出,即在 (TOKEN/BNB LP) 中獲取 LP 池地址。
給定令牌地址: 0xe56842ed550ff2794f010738554db45e60730371
我想獲取 BIN/BNB 礦池地址: 0xe432afB7283A08Be24E9038C30CA6336A7cC8218 。
任何想法可能是什么問題?
from web3 import Web3
from eth_abi.packed import encode_abi_packed
from eth_abi import encode_abi
import eth_abi
"""
Contract: 0xe56842ed550ff2794f010738554db45e60730371
BIN/BNB Address: 0xe432afB7283A08Be24E9038C30CA6336A7cC8218
BIN/BNB LP URL: https://bscscan.com/token/0xe432afB7283A08Be24E9038C30CA6336A7cC8218#balances
"""
CONTRACTS = {"CONTRACT": "0xe56842ed550ff2794f010738554db45e60730371",}
PANCAKE_SWAP_FACTORY = "0xcA143Ce32Fe78f1f7019d7d551a6402fC5350c73"
PANCAKE_SWAP_ROUTER = "0x10ED43C718714eb63d5aA57B78B54704E256024E"
WBNB_ADDRESS = "0xbb4CdB9CBd36B01bD1cBaEBF2De08d9173bc095c"
hexadem_= '0x96e8ac4277198ff8b6f785478aa9a39f403cb768dd02cbee326c3e7da348845f'
factory = PANCAKE_SWAP_FACTORY
abiEncoded_1 = encode_abi_packed(['address', 'address'], (CONTRACTS['CONTRACT'], WBNB_ADDRESS))
salt_ = Web3.solidityKeccak(['bytes'], ['0x' +abiEncoded_1.hex()])
abiEncoded_2 = encode_abi_packed([ 'address', 'bytes32'], ( factory, salt_))
resPair = Web3.solidityKeccak(['bytes','bytes'], ['0xff' + abiEncoded_2.hex(), hexadem_])[12:]
# resPair is the address for the pancakeswap CONTRACT /WBNB pair
print("Token Contract: ", CONTRACTS)
print("BNB-LP Address: ", resPair.hex()) #-- expecting to get 0xe432afB7283A08Be24E9038C30CA6336A7cC8218
當前輸出:
BNB-LP Address: 0xde173b8a63b9641a531de0fbb1c5c9eee3b4bc0c
預期輸出:
Token Contract: 0xe56842ed550ff2794f010738554db45e60730371
BNB-LP Address: 0xe432afB7283A08Be24E9038C30CA6336A7cC8218 #-- correct LP Address
您需要按字母順序輸入兩個硬幣。
pair_traded = [token_a, token_b] #token_a, token_b are the address's
pair_traded.sort()
hexadem_1 = 0xff
abiEncoded_1 = encode_abi_packed(['address', 'address'], (token_list[0], token_list[1] ))
salt_ = w3.solidityKeccak(['bytes'], ['0x' +abiEncoded_1.hex()])
abiEncoded_2 = encode_abi_packed([ 'address', 'bytes32'], ( factory, salt_))
pair_address = w3.solidityKeccak(['bytes','bytes'], ['0xff' + abiEncoded_2.hex(), pair_code_hash])[12:]
對於 Pancake Swap,我認為 hexadem_ 應該是這樣的: hexadem_= '0x00fb7f630766e6a796048ea87d01acd3068e8ff67d078148a3fa3f4a84f69bd5' ## Pancake SWAP
您的代碼中的一個用於 UniSwap
hexadem_ = '0x00fb7f630766e6a796048ea87d01acd3068e8ff67d078148a3fa3f4a84f69bd5' # This is pancake right hex。
factory = PANCAKE_SWAP_FACTORY
abiEncoded_1 = encode_abi_packed(['address', 'address'], (CONTRACTS['CONTRACT'], WBNB_ADDRESS))
salt_ = Web3.solidityKeccak(['bytes'], ['0x' +abiEncoded_1.hex()])
abiEncoded_2 = encode_abi_packed([ 'address', 'bytes32'], ( factory, salt_))
resPair = Web3.solidityKeccak(['bytes','bytes'], ['0xff' + abiEncoded_2.hex(), hexadem_])[12:]
但是我輸出的值和你的不一樣,我的輸出值:
Token Contract: {'CONTRACT': '0xe56842ed550ff2794f010738554db45e60730371'}
BNB-LP Address: 0xdbb161367d9a2a852ebeef3cbfcbf2c43b85064b
我發現你需要確保兩個地址都是小寫或大寫。 這在排序時會有所不同。 這對我有用:
def compute_pool_address(self, token_address_a, token_address_b):
pair_traded = [token_address_a.lower(), token_address_b.lower()]
pair_traded.sort()
hexadem = '0x00fb7f630766e6a796048ea87d01acd3068e8ff67d078148a3fa3f4a84f69bd5'
abiEncoded_1 = encode_abi_packed(['address', 'address'], (pair_traded[0], pair_traded[1]))
salt_ = self.web3.solidityKeccak(['bytes'], ['0x' + abiEncoded_1.hex()])
abiEncoded_2 = encode_abi_packed(['address', 'bytes32'], (PANCAKE_FACTORY_ADDRESS, salt_))
return self.web3.toChecksumAddress(self.web3.solidityKeccak(['bytes', 'bytes'], ['0xff' + abiEncoded_2.hex(), hexadem])[12:])
(self.web3 是一個 web3.py Web3 實例)
在嘗試重新創建 uniswap 合約的功能時,我遇到了類似的問題。 當我在表達式中使用 eth_abi.abi.encode(python) 函數時,它已解決。
Solidity 編碼我想要的:
keccak256(
abi.encodePacked(
hex'ff',
factory,
keccak256(abi.encode(key.token0, key.token1, key.fee)),
POOL_INIT_CODE_HASH
)
)
產生相同結果的 Python 代碼:
encoded_internal_data = abi.encode(['address', 'address', 'uint24'], (token0, token1, fee))
key_hash = web3.solidityKeccak(['bytes'], [encoded_internal_data])
encoded_full_data = encode_abi_packed(
["bytes1", "address", "bytes", "bytes"],
(HexBytes('ff'), UNISWAPV3_FACTORY_ADDRESS, key_hash, HexBytes(UNISWAPV3_POOL_INIT_CODE_HASH))
)
pair_address = web3.solidityKeccak(['bytes'], [encoded_full_data])[12:].hex()
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.