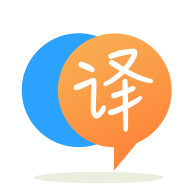
[英]How do I split string value with Regex expression and return an array and object?
[英]how to do array split with regex?
我有一個字符串,我需要將它轉換為一個對象數組
const str = "addias (brand|type) sneakers(product) for men(o)"
預期產出
let output = [
{
key:"addias",
value:["brand","type"]
},
{
key:"sneakers",
value:["product"]
},
{
key:"for men",
value:[]
}
]
我試過的代碼
function gerateSchema(val) {
let split = val.split(" ")
let maps = split.map((i) => {
let obj = i.split("(")
let key = obj[0].replaceAll(/\s/g, "")
let cleanValue = obj[1].replace(/[{()}]/g, "")
let stripedValues = cleanValue.split("|")
return {
key: key,
value: stripedValues,
}
})
return maps
}
let out = gerateSchema(str)
但是當有一些帶有空格的單詞例如for men
時,這會中斷
如何使用正則表達式進行拆分
一種方法是首先執行正則表達式查找所有以查找原始字符串中的所有鍵/值組合。 然后,迭代該結果並使用單詞鍵和數組值構建一個哈希圖。
var str = "addias (brand|type) sneakers(product) for men(o)"; var matches = str.match(/\\w+(?: \\w+)*\\s*\\(.*?\\)/g, str); var array = []; for (var i=0; i < matches.length; ++i) { var parts = matches[i].split(/\\s*(?=\\()/); var map = {}; map["key"] = parts[0]; map["value"] = parts[1].replace(/^\\(|\\)$/g, "").split(/\\|/); array.push(map); } console.log(array);
第一個正則表達式匹配每個鍵/值字符串:
\w+ match a word
(?: \w+)* followed by a space, and another word, the quantity zero or more times
\s* optional whitespace
\( (
.*? pipe separated value string
\) )
然后,我們在\\s*(?=\\()
上分割每個術語,這是緊接在(...|...)
術語之前的空格。最后,我們在管道|
上分割值字符串以生成值的集合。
另一種方法可能是這樣。
const str = "addias (brand|type) sneakers(product) for men(o)" const array = str.split(')').filter(i => i.length).map(i => { const item = i.split('('); return { key: item[0].trim(), value: item[1].split('|') } }) console.log(array)
在regex101.com 的幫助下,推導出以下正則表達式和以下代碼。
([^\\(]+)\\(([^\\)]*)\\)
分解為
([^\\(]+)
- 捕獲 1 個或多個字符直到第一個(
作為組 1
\\(
- 吞下左括號
([^\\)]*)
- 將直到下一次出現)
所有內容都捕獲為第 2 組
\\)
- 吞下右括號
我開始[^|]+
- 解析第 2 組的文本,但實際上使用簡單的split
語句更簡單。
function generateSchema(str) { const regex = /([^\\(]+)\\(([^\\)]*)\\)/mg; // captures the 'word (word)' pattern let m; let output = []; let obj = {}; while ((m = regex.exec(str)) !== null) { // This is necessary to avoid infinite loops with zero-width matches if (m.index === regex.lastIndex) { regex.lastIndex++; } m.forEach((match, groupIndex) => { if (groupIndex === 1) { obj = {}; obj.key = match.trim(); } else if (groupIndex === 2) { obj.value = match.split('|').map(i=>i.trim()); output.push(obj); } }); } return output; } const str = "addidas (brand | type ) sneakers(product) for men(o)"; console.log(generateSchema(str));
使用exec method
迭代正則表達式找到的模式可能更簡單。
const str = 'addias(brand|type|size|color) sneakers(pro) for men(o)'; // The regex looks for an initial group of letters, // then matches the string inside the parentheses const regex = /([az]+)\\(([az\\|]+)\\)/g; let myArray; const arr = []; while ((myArray = regex.exec(str)) !== null) { // Destructure out the key and the delimited string const [_, key, ...rest] = myArray; // `split` on the string found in `rest` first element const values = rest[0].split('|'); // Finally push a new object into the output array // (removing "o" for whatever reason) arr.push({ key, value: values.filter(v => v !== 'o') }); } console.log(arr);
試試這個
const str = 'The quick brown fox jumps over the lazy dog.'; const words = str.split(' '); console.log(words[3]); // expected output: "fox" const chars = str.split(''); console.log(chars[8]); // expected output: "k" const strCopy = str.split(); console.log(strCopy); // expected output: Array ["The quick brown fox jumps over the lazy dog."]
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.