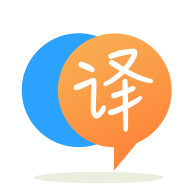
[英]Nodejs crypto.pbkdf2 result is different from CryptoJS.PBKDF2
[英]Nodejs mysql .query() with Crypto.PBKDF2() difference?
const key = CryptoJS.PBKDF2(passphrase, salt, { hasher: CryptoJS.algo.SHA512, keySize: 512/32, iterations: 1000});
const sql = `
SELECT
p.PRIVILEGE_LEVEL_NAME, user.USER_ID, user.USER_NAME ,user.PERMITTED_FUNCTIONS
FROM
user_priviledge_table p
INNER JOIN
user_account_table user on user.PRIVILEGE_LEVEL = p.PRIVILEGE_LEVEL
WHERE
user.user_name='${req.body.user_name}' and user.password='${key}' LIMIT 1
`;
const [payload] = await db.query(sql);
這將等於相同的結果:
const key = CryptoJS.PBKDF2(passphrase, salt, { hasher: CryptoJS.algo.SHA512, keySize: 512/32, iterations: 1000});
const sql = `
SELECT
p.PRIVILEGE_LEVEL_NAME, user.USER_ID, user.USER_NAME ,user.PERMITTED_FUNCTIONS
FROM
user_priviledge_table p
INNER JOIN
user_account_table user on user.PRIVILEGE_LEVEL = p.PRIVILEGE_LEVEL
WHERE
user.user_name=? and user.password=? LIMIT 1
`;
const [payload] = await db.query(sql, [req.body.user_name, key.toString(CryptoJS.enc.Hex)]);
我想知道db.query(sql)
如何將 WordArray object 十六進制轉換為 String 以及這兩種方法之間的確切區別。
哪個更好用?
query()
方法的結構如下:
Connection.prototype.query = function query(sql, values, cb) {
var query = Connection.createQuery(sql, values, cb);
query._connection = this;
if (!(typeof sql === 'object' && 'typeCast' in sql)) {
query.typeCast = this.config.typeCast;
}
if (query.sql) {
query.sql = this.format(query.sql, query.values);
}
if (query._callback) {
query._callback = wrapCallbackInDomain(this, query._callback);
}
this._implyConnect();
return this._protocol._enqueue(query);
};
createQuery()
Connection.createQuery = function createQuery(sql, values, callback) {
if (sql instanceof Query) {
return sql;
}
var cb = callback;
var options = {};
if (typeof sql === 'function') {
cb = sql;
} else if (typeof sql === 'object') {
options = Object.create(sql);
if (typeof values === 'function') {
cb = values;
} else if (values !== undefined) {
Object.defineProperty(options, 'values', { value: values });
}
} else {
options.sql = sql;
if (typeof values === 'function') {
cb = values;
} else if (values !== undefined) {
options.values = values;
}
}
if (cb !== undefined) {
cb = wrapCallbackInDomain(null, cb);
if (cb === undefined) {
throw new TypeError('argument callback must be a function when provided');
}
}
return new Query(options, cb);
};
檢查文件
另一方面,我認為您描述的兩種方法在技術上是相同的,不同之處在於傳遞值的方式,通常在文檔中我們可以找到 3 種調用query()
的方法。
.query() 最簡單的形式是.query(sqlString, callback)
,其中SQL字符串是第一個參數,第二個是回調:
connection.query('SELECT * FROM `books` WHERE `author` = "David"', function (error, results, fields) {
// error will be an Error if one occurred during the query
// results will contain the results of the query
// fields will contain information about the returned results fields (if any)
});
第二種形式.query(sqlString, values, callback)
在使用占位符值時出現(請參閱 escaping 查詢值):
connection.query('SELECT * FROM `books` WHERE `author` = ?', ['David'], function (error, results, fields) {
// error will be an Error if one occurred during the query
// results will contain the results of the query
// fields will contain information about the returned results fields (if any)
});
第三種形式.query(options, callback)
在查詢中使用各種高級選項時出現,例如 escaping 查詢值、使用重疊列名、超時和類型轉換的連接。
connection.query({
sql: 'SELECT * FROM `books` WHERE `author` = ?',
timeout: 40000, // 40s
values: ['David']
}, function (error, results, fields) {
// error will be an Error if one occurred during the query
// results will contain the results of the query
// fields will contain information about the returned results fields (if any)
});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.