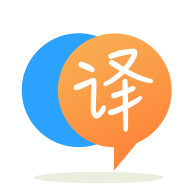
[英]TypeError: Cannot read properties of undefined (reading 'noticeNo') React.js
[英]TypeError: Cannot read properties of undefined (reading 'params') in React js
我想通過傳遞員工 ID 來查看員工詳細信息。 這是我的員工列表頁面。
import React, { Component } from 'react'
import EmployeeService from '../employeeServices'
import { useNavigate } from 'react-router-dom'
class EmployeeList extends Component {
constructor(props) {
super(props)
this.state = {
employees: []
}
this.addEmployee = this.addEmployee.bind(this);
this.editEmployee = this.editEmployee.bind(this);
this.deleteEmployee = this.deleteEmployee.bind(this);
}
deleteEmployee(id){
EmployeeService.deleteEmployee(id).then( res => {
this.setState({employees: this.state.employees.filter(employee => employee.id !== id)});
});
}
viewEmployee(id){
this.props.navigate(`/viewEmployee/${id}`);
}
editEmployee(id){
this.props.navigate(`/addEmployee/${id}`);
}
componentDidMount(){
EmployeeService.getEmployees().then((res) => {
this.setState({ employees: res.data});
console.log(res.data);
});
}
addEmployee(){
this.props.navigate('/addEmployee/_add');
}
render() {
return (
<div>
<h2 className="text-center">Employees List</h2>
<div className = "row">
<button className="btn btn-primary" onClick={this.addEmployee}> Add Employee</button>
</div>
<br></br>
<div className = "row">
<table className = "table table-striped table-bordered">
<thead>
<tr>
<th> Employee First Name</th>
<th> Employee Last Name</th>
<th> Employee Email Id</th>
<th> Actions</th>
</tr>
</thead>
<tbody>
{
this.state.employees.map(
employee =>
<tr key = {employee._id}>
<td> {employee.firstName} </td>
<td> {employee.lastName}</td>
<td> {employee.emailId}</td>
<td>
<button onClick={ () => this.editEmployee(employee._id)} className="btn btn-info">Update </button>
<button style={{marginLeft: "10px"}} onClick={ () => this.deleteEmployee(employee.id)} className="btn btn-danger">Delete </button>
<button style={{marginLeft: "10px"}} onClick={ () => this.viewEmployee(employee._id)} className="btn btn-info">View </button>
</td>
</tr>
)
}
</tbody>
</table>
</div>
</div>
)
}
}
function WithNavigate(props){
const navigate = useNavigate();
return <EmployeeList {...props} navigate={navigate}/>
}
export default WithNavigate;
在下面您可以看到我的員工詳細信息查看頁面。
import React, { Component } from 'react'
import EmployeeService from '../employeeServices'
class ViewEmployees extends Component {
constructor(props) {
super(props)
this.state = {
id: this.props.match.params.id,
employee: {}
}
}
componentDidMount(){
EmployeeService.getEmployeeById(this.state.id).then( res => {
this.setState({employee: res.data});
console.log(this.state.id);
})
}
render() {
return (
<div>
<br></br>
<div className = "card col-md-6 offset-md-3">
<h3 className = "text-center"> View Employee Details</h3>
<div className = "card-body">
<div className = "row">
<label> Employee First Name: </label>
<div> { this.state.employee.firstName }</div>
</div>
<div className = "row">
<label> Employee Last Name: </label>
<div> { this.state.employee.lastName }</div>
</div>
<div className = "row">
<label> Employee Email ID: </label>
<div> { this.state.employee.emailId }</div>
</div>
</div>
</div>
</div>
)
}
}
export default ViewEmployees;
我把<Route path = "/viewEmployee/:id" element={<ViewEmployees/>}></Route>
放在我的 App.js 中。
當我單擊employeeList 頁面上的查看按鈕時,它顯示諸如TypeError 之類的錯誤:無法讀取未定義的屬性(讀取“參數”)
我該如何克服呢?
嘗試使用useParams
掛鈎檢索參數:
import { useParams } from 'react';
...
constructor(props) {
super(props)
this.state = {
id: -1,
employee: {}
}
}
componentDidMount(){
const { id } = useParams();
this.setState({ id })
EmployeeService.getEmployeeById(this.state.id).then( res => {
this.setState({employee: res.data});
console.log(this.state.id);
})
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.