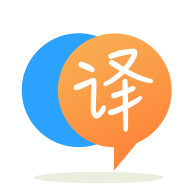
[英]How to combine Material-table with Material-UI Dialog? (ReactJS)
[英]How check the state of individual checkbox in reactjs (material-table)
我有一個表,其中包含從數據庫中獲取的學生列表。 當我單擊每個學生行時,將顯示一個模式彈出窗口,其中包含另一個 4 行和 3 列的表。 模式中的每一列將包含 4 個復選框。 當我單擊一個復選框時,我想將該行的狀態列從“待定”更改為“已付款”,並將徽章屬性類型從“危險”更改為“成功”。 問題是當我單擊一個復選框時,它會將所有列的狀態從“待定”更改為“已付款”,而不是僅僅更改單行。
這是我的代碼
import {
Table,
TableBody,
TableCell,
Badge,
TableFooter,
TableRow,
TableHeader,
TableContainer,
Input,
Modal,
ModalHeader,
ModalBody,
ModalFooter,
Button,
} from "@windmill/react-ui";
import MaterialTable from "material-table";
import AddBox from "@material-ui/icons/AddBox";
import ArrowDownward from "@material-ui/icons/ArrowDownward";
import Check from "@material-ui/icons/Check";
import ChevronLeft from "@material-ui/icons/ChevronLeft";
import ChevronRight from "@material-ui/icons/ChevronRight";
import Clear from "@material-ui/icons/Clear";
import DeleteOutline from "@material-ui/icons/DeleteOutline";
import Edit from "@material-ui/icons/Edit";
import FilterList from "@material-ui/icons/FilterList";
import FirstPage from "@material-ui/icons/FirstPage";
import LastPage from "@material-ui/icons/LastPage";
import Remove from "@material-ui/icons/Remove";
import SaveAlt from "@material-ui/icons/SaveAlt";
import Search from "@material-ui/icons/Search";
import ViewColumn from "@material-ui/icons/ViewColumn";
import React, { forwardRef, useState } from "react";
const Admin = () => {
const [isModalOpen, setIsModalOpen] = useState(false);
const [id, setId] = useState("");
function openModal(ids) {
setId(ids.studentId);
setIsModalOpen(true);
}
function closeModal() {
setIsModalOpen(false);
}
const tableIcons = {
Add: forwardRef((props, ref) => <AddBox {...props} ref={ref} />),
Check: forwardRef((props, ref) => <Check {...props} ref={ref} />),
Clear: forwardRef((props, ref) => <Clear {...props} ref={ref} />),
Delete: forwardRef((props, ref) => <DeleteOutline {...props} ref={ref} />),
DetailPanel: forwardRef((props, ref) => (
<ChevronRight {...props} ref={ref} />
)),
Edit: forwardRef((props, ref) => <Edit {...props} ref={ref} />),
Export: forwardRef((props, ref) => <SaveAlt {...props} ref={ref} />),
Filter: forwardRef((props, ref) => <FilterList {...props} ref={ref} />),
FirstPage: forwardRef((props, ref) => <FirstPage {...props} ref={ref} />),
LastPage: forwardRef((props, ref) => <LastPage {...props} ref={ref} />),
NextPage: forwardRef((props, ref) => <ChevronRight {...props} ref={ref} />),
PreviousPage: forwardRef((props, ref) => (
<ChevronLeft {...props} ref={ref} />
)),
ResetSearch: forwardRef((props, ref) => <Clear {...props} ref={ref} />),
Search: forwardRef((props, ref) => <Search {...props} ref={ref} />),
SortArrow: forwardRef((props, ref) => (
<ArrowDownward {...props} ref={ref} />
)),
ThirdStateCheck: forwardRef((props, ref) => (
<Remove {...props} ref={ref} />
)),
ViewColumn: forwardRef((props, ref) => <ViewColumn {...props} ref={ref} />),
};
const [status, setStatus] = useState("pending");
const [badge, setBadge] = useState("danger");
const handleClick = (e) => {
//Do something if checkbox is clicked
if (e.target.checked) {
setStatus("paid");
setBadge("success");
} else {
setStatus("pending");
setBadge("danger");
}
};
// Table to be rendered in the modal
function renderTable() {
return (
<TableContainer>
<Table>
<TableHeader>
<TableRow>
<TableCell>Dues</TableCell>
<TableCell>Amount</TableCell>
<TableCell>Status</TableCell>
</TableRow>
</TableHeader>
<TableBody>
<TableRow>
<TableCell>
<div className='flex items-center text-sm'>
<span className='font-semibold ml-2'>Level 100</span>
</div>
</TableCell>
<TableCell>
<span className='text-sm'>100</span>
</TableCell>
<TableCell>
<Badge type={badge}>{status}</Badge>
<Input
type='checkbox'
className='ml-6'
onClick={(e) => handleClick(e)}
/>
</TableCell>
</TableRow>
<TableRow>
<TableCell>
<div className='flex items-center text-sm'>
<span className='font-semibold ml-2'>Level 200</span>
</div>
</TableCell>
<TableCell>
<span className='text-sm'>100</span>
</TableCell>
<TableCell>
<Badge type={badge}>{status}</Badge>
<Input
type='checkbox'
className='ml-6'
onClick={(e) => handleClick(e)}
/>
</TableCell>
</TableRow>
<TableRow>
<TableCell>
<div className='flex items-center text-sm'>
<span className='font-semibold ml-2'>Level 300</span>
</div>
</TableCell>
<TableCell>
<span className='text-sm'>100</span>
</TableCell>
<TableCell>
<Badge type={badge}>{status}</Badge>
<Input
type='checkbox'
className='ml-6'
onClick={(e) => handleClick(e)}
/>
</TableCell>
</TableRow>
<TableRow>
<TableCell>
<div className='flex items-center text-sm'>
<span className='font-semibold ml-2'>Level 400</span>
</div>
</TableCell>
<TableCell>
<span className='text-sm'>100</span>
</TableCell>
<TableCell>
<Badge type={badge}>{status}</Badge>
<Input
type='checkbox'
className='ml-6'
onClick={(e) => handleClick(e)}
/>
</TableCell>
</TableRow>
</TableBody>
</Table>
<TableFooter></TableFooter>
</TableContainer>
);
}
return (
<React.Fragment>
{/* Modal popup */}
<Modal isOpen={isModalOpen} onClose={closeModal}>
<ModalHeader>{id}</ModalHeader>
<ModalBody>{renderTable()}</ModalBody>
<ModalFooter>
<Button
className='w-full sm:w-auto'
layout='outline'
onClick={closeModal}>
Cancel
</Button>
<Button className='w-full sm:w-auto'>Accept</Button>
</ModalFooter>
</Modal>
<MaterialTable
icons={tableIcons}
columns={[
{ title: "Student ID", field: "studentId" },
{ title: "Level", field: "level" },
{ title: "Programme", field: "programme" },
]}
data={[
{
studentId: "UG0222021",
level: "200",
programme: "BCOM",
},
{
studentId: "UG0199821",
level: "200",
programme: "BCOM",
},
]}
title='Students Information'
onRowClick={(event, rowData) => openModal(rowData)}
/>
</React.Fragment>
);
};
export default Admin;
這僅僅是因為您將一個 state 用於所有狀態徽章。 您應該使用不同的狀態來管理每個徽章。 您可以將name
屬性添加到每個輸入,這樣您就可以在handleClick
function 中查看哪個輸入正在更改,然后更新相關的 state。 您可以添加以下代碼:
/* states */
const[statusOfLevel100, setStatusOfLevel100] = useState('pending')
const[statusOfLevel200, setStatusOfLevel200] = useState('pending')
const[statusOfLevel300, setStatusOfLevel300] = useState('pending')
const[statusOfLevel400, setStatusOfLevel400] = useState('pending')
const[badgeOfLevel100, setBadgeOfLevel100] = useState('danger')
const[badgeOfLevel200, setBadgeOfLevel200] = useState('danger')
const[badgeOfLevel300, setBadgeOfLevel300] = useState('danger')
const[badgeOfLevel400, setBadgeOfLevel400] = useState('danger')
...
/* handleClick function */
const handleClick = (e) => {
switch (e.target.name) {
case 'inputLevel100':
if(e.target.checked) {
setStatusOfLevel100('paid')
setBadgeOfLevel100('success')
} else {
setStatusOfLevel100('pending')
setBadgeOfLevel100('danger')
}
break;
case 'inputLevel200':
if(e.target.checked) {
setStatusOfLevel200('paid')
setBadgeOfLevel200('success')
} else {
setStatusOfLevel200('pending')
setBadgeOfLevel200('danger')
}
break;
case 'inputLevel300':
if(e.target.checked) {
setStatusOfLevel300('paid')
setBadgeOfLevel300('success')
} else {
setStatusOfLevel300('pending')
setBadgeOfLevel300('danger')
}
break;
case 'inputLevel400':
if(e.target.checked) {
setStatusOfLevel400('paid')
setBadgeOfLevel400('success')
} else {
setStatusOfLevel400('pending')
setBadgeOfLevel400('danger')
}
break;
}
這是一種更通用的方法,我冒昧地使用 typescript 因為它更容易顯示正在發生的事情。
const initialStatuses = {
inputLevel100: "pending",
inputLevel200: "pending",
inputLevel300: "pending",
inputLevel400: "pending",
}
const initialBadges = {
inputLevel100: "danger",
inputLevel200: "danger",
inputLevel300: "danger",
inputLevel400: "danger",
}
const [status, setStatus] = React.useState<{ [key: string]: string }>(initialBadges);
const [badge, setBadge] = React.useState<{ [key: string]: string }>(initialStatuses);
const handleClick = (e: { target: { checked: boolean; name: string} }) => {
const name = e.target.name;
if (e.target.checked) {
setBadge({
...badge,
[name]: "success",
});
setStatus({
...status,
[name]: "paid",
});
}
};
你可以通過簡單地說得到狀態或徽章的價值
status["Level200"] // Should return either "pending" or "paid"
或者
badge["Level200"] // Should return either "danger" or "success"
這應該非常高效(請參閱JavaScript object 中的密鑰查找性能了解更多信息)
但是,更重要的是,您現在有了一種添加更多狀態和徽章的通用方法,而不必擔心為每個新添加的狀態或徽章添加useState
另外,這種方法的另一個額外優勢是,您可以(比如說)從遠程 api 獲取狀態和徽章列表,或者(比如說)有一個statuses.js
文件,其中包含一個 statuses 列表和一個badges.js
文件客戶端,您可以然后 map 過來。 然后,這使您的代碼更容易擴展,並且刪除/添加新狀態和徽章變得微不足道
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.