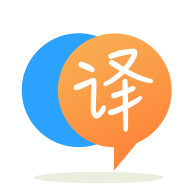
[英]Write test to check local setState call with jest and react-testing-library
[英]How can I write unit test for api call with token using React, Jest and React-testing-library?
這是我想測試的功能,它需要一個令牌和一個描述作為道具。 通常在 React 代碼中,我可以從 useContext 獲取令牌。
export const updateUserProfileAbout = async (
token,
description
) => {
const dataUpdateTemplateDescriptionRes = await patchData(`me/`, token, {
about:description,
});
const dataUpdateTemplateDescriptionJson = await dataUpdateTemplateDescriptionRes.json();
return dataUpdateTemplateDescriptionJson;
};
這是我的自定義 patchData 函數:
const patchData = async (urn, token, data = "") => {
const headers = {
"Content-Type": "application/json",
Authorization: `Bearer ${token.access}`,
};
const body = data ? JSON.stringify(data) : null;
let response;
if (body) {
response = await fetch(`${host}/api/${urn}`, {
method: "PATCH",
headers,
body,
});
} else {
response = await fetch(`${host}/api/${urn}`, {
method: "PATCH",
headers,
});
}
if (!response.ok) throw new Error(response.status);
return response;
};
經過一天的研究,雖然我可能錯了,但我認為在前端單元測試時我不必關心令牌或授權。 我只需要 jest.fn() 來模擬 function 和 jest.spyOn(global, "fetch") 來跟蹤獲取 API。
你是對的。 你不需要令牌。 模擬獲取所需要做的全部如下:
jest.spyOn(global, 'fetch').mockImplementationOnce(
jest.fn(() => Promise.resolve()) as jest.Mock);
如果要從 json 響應中檢索特定對象,可以使用:
jest.spyOn(global, 'fetch').mockImplementationOnce(
jest.fn(() => Promise.resolve({ ok: true, json: () => Promise.resolve({ myObject }) })) as jest.Mock);
您也可以拒絕它以觸發錯誤捕獲:
jest.spyOn(global, 'fetch').mockImplementationOnce(
jest.fn(() => Promise.reject()) as jest.Mock);
如果您想多次返回某些內容,請將mockImplementationOnce
更改為您需要的任何內容(可能mockImplementation
,以便在您每次調用它時返回它)。
如果您還希望調用 fetch 只需添加一個常量:
const myFetch = jest.spyOn(global, 'fetch').mockImplementationOnce(
jest.fn(() => Promise.reject()) as jest.Mock);
然后你可以通過以下方式期待它: expect(myFetch).toBecalledTimes(1);
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.