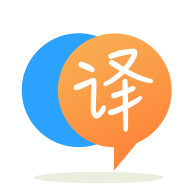
[英]How to get an image of a scanned QR Code with ZXING in Java? #667
[英]How to convert image to qr code using java?
如果我們搜索圖像到二維碼,有很多網站在線將圖像轉換為二維碼。
但是我怎么能在 java 中做到這一點?
我正在使用以下代碼將文本轉換為二維碼:-
import java.io.File;
import java.io.IOException;
import java.util.HashMap;
import java.util.Map;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.MultiFormatWriter;
import com.google.zxing.NotFoundException;
import com.google.zxing.WriterException;
import com.google.zxing.client.j2se.MatrixToImageWriter;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
public class MyQr {
public static void createQR(String data, String path,
String charset, Map hashMap,
int height, int width)
throws WriterException, IOException
{
BitMatrix matrix = new MultiFormatWriter().encode(
new String(data.getBytes(charset), charset),
BarcodeFormat.QR_CODE, width, height);
MatrixToImageWriter.writeToFile(
matrix,
path.substring(path.lastIndexOf('.') + 1),
new File(path));
}
public static void main(String[] args)
throws WriterException, IOException,
NotFoundException
{
String data = "TEXT IN QR CODE";
String path = "image.png";
String charset = "UTF-8";
Map<EncodeHintType, ErrorCorrectionLevel> hashMap
= new HashMap<EncodeHintType,
ErrorCorrectionLevel>();
hashMap.put(EncodeHintType.ERROR_CORRECTION,
ErrorCorrectionLevel.L);
createQR(data, path, charset, hashMap, 200, 200);
}
}
但是如何在二維碼中編碼圖像呢?
我假設您的意思是要向 QR 碼添加圖像(例如徽標)。
這通常是通過在二維碼頂部(通常在中心)覆蓋一個小圖像來完成的。 當一小部分被遮擋時,二維碼仍然可讀,尤其是在中心。
可以使用MatrixToImageWriter.toBufferedImage(matrix)
獲取包含二維碼圖像的 BufferedImage,然后使用 Java 圖像方法疊加圖像。 例如,參見java 中的疊加圖像
圖片太大而無法嵌入二維碼(小圖標除外)。 因此,圖像被放置在可公開訪問的服務器上,圖像的 URL 嵌入在二維碼中。
因此,您需要訪問可以實現此目的的服務器。 這可能是您將要實施的大部分內容。
否則,它是直截了當的:
import java.io.IOException;
import java.net.MalformedURLException;
import java.net.URL;
import java.nio.file.Path;
import java.util.HashMap;
import java.util.Map;
import com.google.zxing.BarcodeFormat;
import com.google.zxing.EncodeHintType;
import com.google.zxing.MultiFormatWriter;
import com.google.zxing.WriterException;
import com.google.zxing.client.j2se.MatrixToImageWriter;
import com.google.zxing.common.BitMatrix;
import com.google.zxing.qrcode.decoder.ErrorCorrectionLevel;
public class MyQr {
/**
* Uploads the image to a server and returns to image URL.
* @param imagePath path to the image
* @return image URL
*/
public static URL uploadImage(String imagePath)
{
// implement a solution to put an image on a public server
try {
return new URL("https://yourserver/some_path/image.jpg");
} catch (MalformedURLException e) {
throw new RuntimeException(e);
}
}
public static void createQR(String payloadImagePath, String qrCodeImagePath,
Map<EncodeHintType, ?> hints, int height, int width)
throws WriterException, IOException
{
URL imageURL = uploadImage(payloadImagePath);
BitMatrix matrix = new MultiFormatWriter().encode(
imageURL.toString(), BarcodeFormat.QR_CODE, width, height, hints);
String imageFormat = qrCodeImagePath.substring(qrCodeImagePath.lastIndexOf('.') + 1);
MatrixToImageWriter.writeToPath(matrix, imageFormat, Path.of(qrCodeImagePath));
}
public static void main(String[] args)
throws WriterException, IOException
{
String payloadImagePath = "embed_this.jpg";
String qrCodeImagePath = "qrcode.png";
Map<EncodeHintType, ErrorCorrectionLevel> hints = new HashMap<>();
hints.put(EncodeHintType.ERROR_CORRECTION, ErrorCorrectionLevel.L);
createQR(payloadImagePath, qrCodeImagePath, hints, 200, 200);
}
}
順便說一句:下面的代碼(來自您的代碼示例)是無意義的。 充其量,它會創建字符串的副本,這是不必要的。 在最壞的情況下,它會破壞charset
不支持的所有字符。 我不認為你打算任何一個。
new String(data.getBytes(charset), charset)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.