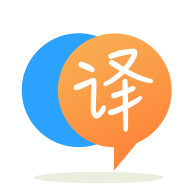
[英]Error: Constructor does not have return type and it says that variables x and y are undeclared
[英]Is it possible to have a constructor with 1 input parameter of undeclared type?
這是我想做的事情:
Class 有兩個對象( string myStr
和int myInt
)
構造函數接受1 個參數(數據類型不固定)。
If
參數是字符串: myStr = parameter; myInt = parameter.length();
myStr = parameter; myInt = parameter.length();
If
參數是 int: myInt = parameter; myStr = "0000...0"
myInt = parameter; myStr = "0000...0"
(長度為“參數”的字符串,由 for 循環設置)
Else
: cout << "Error";
是否可以做我在第 2 行中描述的事情?
我已經設法僅使用字符串和轉換數據類型(在為myStr
的長度施加人為下限時能夠做到這一點)做一些變通方法,但這會導致我進一步的其他問題。
是否可以有一個帶有 1 個未聲明類型的輸入參數的構造函數?
從技術上講,您可以使用可變參數,允許未指定數量的未指定類型的 arguments。 但我建議不要使用它。
必須聲明所有其他參數的類型。
如果參數是字符串: myStr = parameter; myInt = parameter.length();
如果參數是 int: myInt = parameter; myStr = "0000...0"(長度為“參數”的字符串,由 for 循環設置)
您可以使用兩個構造函數而不是一個構造函數輕松實現這一點。 一個接受字符串,另一個接受 integer。
這是討論的想法的示例,重載構造函數和模板化構造函數(主要用於教育目的)。 我想你明白為什么在這種情況下重載被認為更實用:)
#include <iostream>
#include <string>
#include <type_traits>
//-------------------------------------------------------------------------------------------------
// class wit overloaded constructorss
class my_class_t
{
public:
explicit my_class_t(const std::size_t& size) :
m_size{ size }
{
}
explicit my_class_t(const std::string& string) :
m_string{ string },
m_size{ string.size() }
{
}
// any other type of parameter to the constructor will just not compile
std::size_t size() const
{
return m_size;
}
private:
std::string m_string;
std::size_t m_size;
};
//-------------------------------------------------------------------------------------------------
// class with templated constructor
// it is a bit more complicated :)
class my_class_template_constructor_t
{
public:
// this is the syntax for a function that can accept a parameter of any type
template<typename type_t>
explicit my_class_template_constructor_t(const type_t& value)
{
// select code based on some compile time logic.
// e.g. if type_t can be converted to the type of m_size then select the first
// bit to compile (that's what if constexpr is for)
if constexpr (std::is_convertible_v<type_t,decltype(m_size)>)
{
m_size = static_cast<int>(value);
}
else
// if a std::string can be constructed from type_t
if constexpr (std::is_constructible_v<std::string, type_t>)
{
m_string = value;
m_size = m_string.size();
}
else
// give a compile time error (not a runtime one)
{
static_assert(false, "unsupported type for type_t");
}
}
std::size_t size() const
{
return m_size;
}
private:
std::size_t m_size;
std::string m_string;
};
//-------------------------------------------------------------------------------------------------
int main()
{
my_class_t c1{ 42 };
my_class_t c2{ "Hello world!" };
std::cout << c1.size() << "\n";
std::cout << c2.size() << "\n";
my_class_template_constructor_t ct1{ 42 };
my_class_template_constructor_t ct2{ "Happy new year!" };
std::cout << ct1.size() << "\n";
std::cout << ct2.size() << "\n";
return 0;
}
問題不在於使用單個參數,而是您需要稍后找出參數的數據類型。
一個常用的技巧是使用通用指針或通用 object class 參考或指針。
MyClass::MyClass ( void* P );
MyClass::MyClass ( void& SomeBaseClass );
由於您正在處理更簡單的數據,您可能想嘗試一種 OO 方法,例如將值嵌套在對象中,然后檢查數據的類型:
class BaseClass
{
// ...
} ;
class IntegerClass: BaseClass
{
int Value;
} ;
class StringClass: BaseClass
{
String Value;
} ;
您的 class 可以收到“BaseClass” object 參考:
class MyClass
{
private int MyInt;
private String MyString;
MyClass ( BaseClass& P )
{
if ( P is IntegerClass )
{
//
}
else if ( P is StringClass )
{
//
}
}
} ;
int main (...)
{
IntegerClass *i = new IntegerClass(5);
StringClass *s = new StringClass ("Hello");
MyClass *O1 = new MyClass(i);
MyClass *O2 = new MyClass(s);
// Display values of O1 and O2 here
free O2;
free O1;
free s;
free i;
return 0;
}
就我的兩個比特幣...
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.