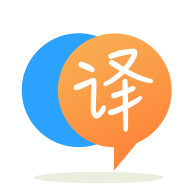
[英]Jest/Typescript: Mock class dependencies in Jest and Typescript
[英]Jest/Typescript: Mock class dependencies containing private members in jest and typescript
Context: I have class B which is dependent on class A. I want to test a method of class B which is internally calling a method of class A. Now, I want to unit test my method of class B by mocking class A.
注 1 : Class A 有一些私有成員
注2 : Class A無接口
這是我的代碼結構:
class Base {
someMethod() {
return "Hello ";
}
}
class A {
private _baseClassImpl: Base;
constructor(baseClassImpl: Base) {
this._baseClassImpl = baseClassImpl;
}
getSomething() {
return this._baseClassImpl.someMethod() + " Something";
}
}
class B {
constructor(objectOfClassA: A) {
this._objectOfClassA = objectOfClassA;
}
functionOfClassBToTest() {
const returnValueFromClassA = this._objectOfClassA.getSomething();
return returnValueFromClassA;
}
}
到目前為止我已經嘗試過:
從我之前的 SO 帖子中獲得建議后,我嘗試編寫如下測試:
const getSomethingMock = jest.fn().mockImplementation(() => {
return "Mock value";
});
const mockA = jest.Mocked<A> = {
getSomething: getSomethingMock
};
test("test functionOfClassBToTest", () => {
const classBToTest = new B(mockA);
expect(classBToTest.functionofClassBToTest.toStrictEqual("Hello Something");
});
上面的代碼給了我這個錯誤:
Type '{ getSomething: jest.Mock<any, any>; }' is not assignable is not assignable to type 'Mocked<A>'.
Type '{ getSomething: jest.Mock<any, any>; }' is missing the following properties from type 'A': _baseClassImpl
當我嘗試像這樣為 _baseClassImpl 提供模擬值時:
const baseClassMock = jest.Mocked<Base> = {
someMethod: jest.fn()
};
const getSomethingMock = jest.fn().mockImplementation(() => {
return "Mock value";
});
const mockA = jest.Mocked<A> = {
getSomething: getSomethingMock,
_baseClassImpl: baseClassMock
};
Typescript 給了我這個錯誤:
Type '{ getSomething: jest.Mock<any, any>; _baseClassImpl: jest.Mocked<Base>; }' is not assignable is not assignable to type 'Mocked<A>'.
Type '{ getSomething: jest.Mock<any, any>; _baseClassImpl: jest.Mocked<Base>; }' is not assignable is not assignable to type 'A'.
Property '_baseClassImpl' is private in type 'A' but not in type '{ getSomething: jest.Mock<any, any>; _baseClassImpl: jest.Mocked<Base>; }'
我已經在我之前的 SO 帖子中嘗試過答案,但它在 class 與私人成員的情況下不起作用。
一些注意事項:
注 1 : Class A 包含私有成員
注2 : Class A無接口
注 3 :我不想在我的測試 function 中初始化 class A 的 object。 我只想模擬 class。
通過這個github repo的一些嘗試和幫助,我能夠解決這個問題。
這是供參考的代碼:
測試 functionOfClassBToTest:
//Note: this can be also written as : const mockA = new (<any>A)() as jest.Mocked<A>;
const mockA = new (<new () => A>A)() as jest.Mocked<A>;
mockA.getSomething = jest.fn();
test("test functionOfClassBToTest", () => {
mockA.getSomething.mockReturnValueOnce("Hello Something");
const classBToTest = new B(mockA);
expect(classBToTest.functionofClassBToTest.toStrictEqual("Hello Something");
});
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.