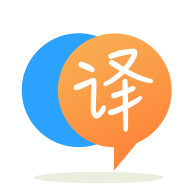
[英]Flutter TextField does not follow ListTiles within ReorderableListView
[英]How to create space between ListTiles in Flutter ReorderableListView
您可以只用底部的填充將您的 listTile 包裝為“分隔符”,這可能並不理想,因為填充將成為小部件的一部分,在拖動時會可見。
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
static const String _title = 'Flutter Code Sample';
@override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: Scaffold(
appBar: AppBar(title: const Text(_title)),
body: const MyStatefulWidget(),
),
);
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({Key? key}) : super(key: key);
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
final List<int> _items = List<int>.generate(50, (int index) => index);
@override
Widget build(BuildContext context) {
final ColorScheme colorScheme = Theme.of(context).colorScheme;
final Color oddItemColor = colorScheme.primary.withOpacity(0.05);
final Color evenItemColor = colorScheme.primary.withOpacity(0.15);
return ReorderableListView(
padding: const EdgeInsets.symmetric(horizontal: 40),
children: <Widget>[
for (int index = 0; index < _items.length; index++)
Padding(
key: Key('$index'),
padding: const EdgeInsets.only(bottom: 4),
child: ListTile(
tileColor: _items[index].isOdd ? oddItemColor : evenItemColor,
title: Text('Item ${_items[index]}'),
)),
],
onReorder: (int oldIndex, int newIndex) {
setState(() {
if (oldIndex < newIndex) {
newIndex -= 1;
}
final int item = _items.removeAt(oldIndex);
_items.insert(newIndex, item);
});
},
);
}
}
使用 ReorderableListView 似乎無法將空間添加為不可重新排序的小部件,即使添加將 ListTiles 與 AbsorbPointer 交錯的虛擬項目仍然會使它們可重新排序。 所以上面的方法至少是可行的
children: <Widget>[
for (int index = 0; index < _items.length; index++)
if (index.isOdd)
ListTile(
key: Key('$index'),
tileColor: _items[index].isOdd ? oddItemColor : evenItemColor,
title: Text('Item ${_items[index]}'),
)
else if (index.isEven)
AbsorbPointer(
key: Key('$index'),
child: SizedBox.square(
key: Key('$index'),
dimension: 40,
),
),
],
將您的 ListTile 包裝在一列中,並使用 SizedBox 分隔列表項。 在列中使用鍵,而不是在 ListTile 中。
import 'package:flutter/material.dart';
void main() => runApp(const MyApp());
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
static const String _title = 'Flutter Code Sample';
@override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: Scaffold(
appBar: AppBar(title: const Text(_title)),
body: const MyStatefulWidget(),
),
);
}
}
class MyStatefulWidget extends StatefulWidget {
const MyStatefulWidget({Key? key}) : super(key: key);
@override
State<MyStatefulWidget> createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
final List<int> _items = List<int>.generate(50, (int index) => index);
@override
Widget build(BuildContext context) {
final ColorScheme colorScheme = Theme.of(context).colorScheme;
final Color oddItemColor = colorScheme.primary.withOpacity(0.05);
final Color evenItemColor = colorScheme.primary.withOpacity(0.15);
return ReorderableListView(
padding: const EdgeInsets.symmetric(horizontal: 40),
children: <Widget>[
for (int index = 0; index < _items.length; index++)
Column(
key: Key('$index'),
children: [
ListTile(
tileColor: _items[index].isOdd ? oddItemColor : evenItemColor,
title: Text('Item ${_items[index]}'),
),
SizedBox(
height: 5,
),
],
),
],
onReorder: (int oldIndex, int newIndex) {
setState(() {
if (oldIndex < newIndex) {
newIndex -= 1;
}
final int item = _items.removeAt(oldIndex);
_items.insert(newIndex, item);
});
},
);
}
}
這就像 ViewList.separated 和 ReorderableList 的混合物
import 'dart:math';
import 'package:flutter/gestures.dart';
import 'package:flutter/material.dart';
class CustomReorderableListView extends ReorderableListView {
CustomReorderableListView.separated({
Key? key,
required IndexedWidgetBuilder itemBuilder,
required IndexedWidgetBuilder separatorBuilder,
required int itemCount,
required ReorderCallback onReorder,
double? itemExtent,
Widget? prototypeItem,
ReorderItemProxyDecorator? proxyDecorator,
bool buildDefaultDragHandles = true,
EdgeInsets? padding,
Widget? header,
Axis scrollDirection = Axis.vertical,
bool reverse = false,
ScrollController? scrollController,
bool? primary,
ScrollPhysics? physics,
bool shrinkWrap = false,
double anchor = 0.0,
double? cacheExtent,
DragStartBehavior dragStartBehavior = DragStartBehavior.start,
ScrollViewKeyboardDismissBehavior keyboardDismissBehavior =
ScrollViewKeyboardDismissBehavior.manual,
String? restorationId,
Clip clipBehavior = Clip.hardEdge,
}) : super.builder(
key: key,
itemCount: max(0, itemCount * 2 - 1),
itemBuilder: (BuildContext context, int index) {
if (index % 2 == 1) {
final separator = separatorBuilder.call(context, index);
if (separator.key == null) {
return KeyedSubtree(
key: ValueKey('ReorderableSeparator${index}Key'),
child: IgnorePointer(child: separator),
);
}
return separator;
}
return itemBuilder.call(context, index ~/ 2);
},
onReorder: (int oldIndex, int newIndex) {
if (oldIndex < newIndex) {
newIndex -= 1;
}
if (oldIndex % 2 == 1) {
//separator - should never happen
return;
}
if ((oldIndex - newIndex).abs() == 1) {
//moved behind the top/bottom separator
return;
}
newIndex = oldIndex > newIndex && newIndex % 2 == 1
? (newIndex + 1) ~/ 2
: newIndex ~/ 2;
oldIndex = oldIndex ~/ 2;
onReorder.call(oldIndex, newIndex);
},
itemExtent: itemExtent,
prototypeItem: prototypeItem,
proxyDecorator: proxyDecorator,
buildDefaultDragHandles: buildDefaultDragHandles,
padding: padding,
header: header,
scrollDirection: scrollDirection,
reverse: reverse,
scrollController: scrollController,
primary: primary,
physics: physics,
shrinkWrap: shrinkWrap,
anchor: anchor,
cacheExtent: cacheExtent,
dragStartBehavior: dragStartBehavior,
keyboardDismissBehavior: keyboardDismissBehavior,
restorationId: restorationId,
clipBehavior: clipBehavior,
);
}
if ((oldIndex - newIndex).abs() == 1) {
return;
}
if (oldIndex > newIndex && newIndex % 2 == 1) {
newIndex = (newIndex + 1) ~/ 2;
} else if (oldIndex > newIndex && newIndex % 2 != 1) {
newIndex = (newIndex) ~/ 2;
} else if (newIndex == 0) {
newIndex = (newIndex ~/ 2);
} else {
newIndex = (newIndex ~/ 2) + 1;
}
oldIndex = oldIndex ~/ 2;
onReorder.call(oldIndex, newIndex);
},
itemExtent: itemExtent,
prototypeItem: prototypeItem,
proxyDecorator: proxyDecorator,
buildDefaultDragHandles: buildDefaultDragHandles,
padding: padding,
header: header,
scrollDirection: scrollDirection,
reverse: reverse,
scrollController: scrollController,
primary: primary,
physics: physics,
shrinkWrap: shrinkWrap,
anchor: anchor,
cacheExtent: cacheExtent,
dragStartBehavior: dragStartBehavior,
keyboardDismissBehavior: keyboardDismissBehavior,
restorationId: restorationId,
clipBehavior: clipBehavior,
);
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.