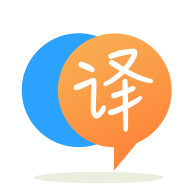
[英]How to get program to ask for input for either one or two answer depending on answers list has
[英]How to break a loop depending on answer from input, then move on with the rest of the program
嗨,所以我對編碼非常陌生,我正在為我的高中項目制作二十一點游戲,我正在要求用戶擊打或站立,當他們擊中時再次詢問他們直到他們破產或站,我想要它,所以一旦他們站起來要求他們擊球或站立休息的循環,但繼續使用代碼的 rest,這是經銷商/計算機擊球或站立的邏輯。 但是當他們站起來時,它只會停止整個程序。 我該如何解決? 代碼還沒有完全完成,我很抱歉可能是一團糟。
# Import the required modules
import random
# Print welcome message
print('Welcome To Blackjack!')
# State the rules for the game
print('This is blackjack. Rules are simple whoever has the biggest sum of the cards at the end wins. Unless someone gets 21 / blackjack or goes over 21 (bust). Bust is when a player goes over 21 and the lose. Once it is your turn you can choose to hit or stand, if you hit you get another card, if you stand it becomes the other persons turn. Your goal is to get the closest number to 21 without going over it. The game uses no suit cards. The values of the face cards are a Jack = 10, Queen = 10, King = 10, Ace = 11 or 1 whatever works the best. ')
# Global Variables
deck = [2, 3, 4, 5, 6, 7, 8, 9, 10, 2, 3, 4, 5, 6, 7, 8, 9, 10, 2, 3, 4, 5, 6, 7, 8, 9, 10, 2, 3, 4, 5, 6, 7, 8, 9, 10, 'J', 'Q', 'K', 'A']
dealer_cards = []
player_cards = []
print('Dealing...')
# Create the dealcard function to deal a card when needed
def dealcard(turn):
card = random.choice(deck)
turn.append(card)
deck.remove(card) # Removes the card out of the deck so it cant repeat.
# Create the function to calculate the total cards that each player has
def totalvalue(turn):
totalvalue = 0
facecards = ['J', 'Q', 'K']
for card in turn:
if card in range(1, 11):
totalvalue += card # This adds the cards together
elif card in facecards: # Checks if a card is a face card (J, Q, K,)
totalvalue += 10 # This gives value to face cards
else: # This checks if they get an ace and what works the best in case when they get an ace
if totalvalue > 11: # If total is over 11 Ace counts as 1
totalvalue += 1
else: # If total is under 11 Ace counts as 11
totalvalue += 11
return totalvalue
for dealing in range(2):
dealcard(dealer_cards)
dealcard(player_cards)
print(f"The dealer's cards are {dealer_cards} and the total amount is {totalvalue(dealer_cards)}")
print(f"Your cards are {player_cards} and your total amount is {totalvalue(player_cards)}")
while True:
# Take input from user
playerchoice = (input('Would you like to \n1.Hit \nor \n2.Stay\n'))
# Check what choice user chose and execute it
if playerchoice == '1':
dealcard(player_cards)
print(f"You now have a total of {totalvalue(player_cards)} with these cards {player_cards}")
continue
# If they chose to stand move on to dealers / computers turn
if playerchoice == '2':
print(f"Your cards stayed the same {player_cards} with a total of {totalvalue(player_cards)}")
print("What will the dealer do?...")
break
# Create dealer logic
if totalvalue(dealer_cards) >= 18:
print(f"The dealer chose to stand their cards are {dealer_cards} with a total of {totalvalue(dealer_cards)}")
if totalvalue(dealer_cards) < 16:
dealcard(dealer_cards)
print(f"The dealer chose to hit their new cards are {dealer_cards} and their total is {totalvalue(dealer_cards)}")
if totalvalue(dealer_cards) == totalvalue(player_cards):
print(f"Its a tie you both have {totalvalue(player_cards)}")
if totalvalue(dealer_cards) == 21:
print(f"The dealer got blackjack! You lose...")
break
我想要它,所以一旦他們堅持要求他們擊球或站立休息,但繼續使用代碼的 rest
您當前的 while 循環while True:
只是無限重復。 相反,您需要一個條件來指定何時要繼續。 例如,你可以做
while playerchoice == '1':
請注意,此更改還需要對您的代碼進行更多更改。 特別是,您將不再需要if
語句,因為只有當用戶選擇“打我”時,while 循環的主體才會重復。 在 while 循環之后,“stand”的邏輯將是 go。 我把細節留給你弄清楚。
我認為你要求的是這樣的。 我不確定該程序是否應該繼續運行以便您可以玩多個游戲,或者它只需要能夠玩一個游戲。 如果它必須能夠玩多個游戲,您會遇到更新全局值的問題。 要使用您擁有的代碼解決此問題,您必須在每次玩新游戲時復制全局值。 正如你所看到的,這看起來不太漂亮,但我試圖讓它盡可能接近你寫的內容。 我發布的游戲中仍然存在一些錯誤,因此您仍然必須復制套牌並以與歸還玩家卡相同的方式歸還套牌。 但那是你要做的:-)
啟動 function 復制您已有的變量並返回它們:
def start():
for dealing in range(2):
copy_dealer_cards = dealer_cards.copy()
copy_player_cards = player_cards.copy()
dealcard(copy_dealer_cards)
dealcard(copy_player_cards)
print(f"The dealer's cards are {copy_dealer_cards} and the total amount is {totalvalue(copy_dealer_cards)}")
print(f"Your cards are {copy_player_cards} and your total amount is {totalvalue(copy_player_cards)}")
return (copy_dealer_cards, copy_player_cards)
比較結果。 在這里,您可以使用返回值來記錄玩家和庄家各自贏了多少局:
def comp(player_res, dealer_res):
if dealer_res>player_res:
print("Dealer wins\n\n")
return 1
if dealer_res<player_res:
print("Player wins\n\n")
return -1
if dealer_res == player_res:
print(f"Its a tie you both have {player_res}")
return 0
玩游戲的主要 function 具有一些更新的邏輯和條件。 以及稍作修改的 while 循環。
def run() -> None:
d_cards , p_cards = start()
player_stay = False
dealer_stay = False
while not(dealer_stay and player_stay):
# Take input from user
if not player_stay:
playerchoice = (input('Would you like to \n1.Hit \nor \n2.Stay\n'))
# Check what choice user chose and execute it
if playerchoice == '1':
dealcard(p_cards)
print(f"You now have a total of {totalvalue(p_cards)} with these cards {p_cards}")
# If they chose to stand move on to dealers / computers turn
if playerchoice == '2':
print(f"Your cards stayed the same {p_cards} with a total of {totalvalue(p_cards)}")
print("What will the dealer do?...")
player_stay = True
if not dealer_stay:
# Create dealer logic
if totalvalue(d_cards) >= 17:
print(f"The dealer chose to stand their cards are {d_cards} with a total of {totalvalue(d_cards)}")
dealer_stay = True
if totalvalue(d_cards) <= 16:
dealcard(d_cards)
print(f"The dealer chose to hit their new cards are {d_cards} and their total is {totalvalue(d_cards)}")
#If bust
if totalvalue(p_cards) > 21:
print(f"Player bust! You lose...\n\n")
break
if totalvalue(d_cards) > 21:
print(f"Dealer bust! You win...\n\n")
break
#If blakcjack
if totalvalue(p_cards) == 21:
print(f"The player got blackjack! You win...\n\n")
break
if totalvalue(d_cards) == 21:
print(f"The dealer got blackjack! You lose...\n\n")
break
#If hit then evaluate
if dealer_stay and player_stay:
comp(totalvalue(p_cards), totalvalue(d_cards))
print("New round begins...")
break
run()
run()
希望這可以幫助:-)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.