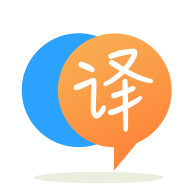
[英]JavaScript classes, how does one apply “Separation of Concerns” and “Don't repeat Yourself” (DRY) in practice
[英]I have these controllers that deletes a user, i want to implement DRY(Don't repeat yourself) principle and make the res json message dynamic
我想為下面的代碼實現 DRY 原則,刪除兩個不同的用戶,並且我想根據提供給 find 方法的 model 的詳細信息實現動態響應
//This code here deletes the student
exports.deleteStudent = async (req, res) => {
try {
let ID = req.query.id
let exactStudent = await Student.findById(ID)
if (!exactStudent) {
return res.status(400).json({ error: "Student with the specified ID not present" })
}
await Student.findByIdAndDelete(ID);
res.status(200).json({ success: 'Student Deleted successfully ' })
} catch (error) {
throw error
}
}
//這里的代碼刪除了老師
exports.deleteTeacher = async (req, res) => {
try {
let ID = req.query.id
let exactTeacher = await Teacher.findById(ID)
if (!exactTeacher) {
return res.status(400).json({ error: "Teacher with the specified ID not present" })
}
await Teacher.findByIdAndDelete(ID);
res.status(200).json({ error: 'Teacher Deleted successfully ' })
} catch (error) {
throw error
}
}
據我了解你的問題,你需要這樣的東西:
async function deletePerson(Model, id) => {
try {
return await Model.findByIdAndDelete(id);
} catch (error) {
throw error;
}
}
// Inside the controllers
exports.deleteStudent = async (req, res) => {
try {
const deletedEntity = await deletePerson(Student, req.query.id);
if (!deletedEntity) {
return res.status(400).json({ error: "Student with the specified ID not present" })
}
res.status(200).json({ error: 'Student Deleted successfully ' })
} catch (error) {
throw error
}
}
exports.deleteTeacher = async (req, res) => {
try {
const deletedEntity = await deletePerson(Teacher, req.query.id);
if (!deletedEntity) {
return res.status(400).json({ error: "Teacher with the specified ID not present" })
}
res.status(200).json({ error: 'Teacher Deleted successfully ' })
} catch (error) {
throw error
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.