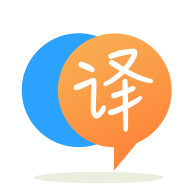
[英]React Hook "useRouter" is called in function "article" that is neither a React function component nor a custom React Hook function
[英]React Hook "useForecast" is called in function "getSearch" that is neither a React function component nor a custom React Hook function
在這里反應新手!
在我一直從事的 React 天氣儀表板項目中,我一遍又一遍地遇到同樣的問題。 我專注於連接提交函數和我創建的預測 function 以從我正在使用的 OpenWeatherMap API 檢索 json 數據。 在使用我創建的回調 function(從子組件中獲取搜索到的城市值)時,會引發上面列出的錯誤。 由於我沒有打算將回調 function 'getSearch' 用作 function 組件或自定義 React Hook,因此我不知道還有什么可以嘗試修復此錯誤。 下面是我的父組件“overview.js”和子組件“recentSearches.js”的所有代碼:
概述.js
import React, {useState} from 'react';
import './overview.css';
import { RecentSearches } from '../Recent Searches/recentSearches';
import { Hourly } from '../Hourly/hourly';
import { Fiveday } from '../5 Day Forecast/fiveday';
require('dotenv').config();
const useForecast = async (city) => {
// const api_key = process.env.API_KEY;
const api_key = '2220f5a5d83243938f764759211310';
var BASE_URL = `http://api.weatherapi.com/v1/forecast.json?key=${api_key}&q=${city}&days=3&aqi=no&alerts=no`;
const response = await fetch(BASE_URL);
const data = await response.json();
console.log(data);
// collects all of the current weather info for your search
const currentTempInfo = {
city: data.location.name,
state: data.location.region,
epochDate: data.location.localtime_epoch,
message: data.current.condition.text,
wicon: data.current.condition.icon,
currentTemp: data.current.temp_f,
currentHighTemp: data.forecast.forecastday[0].day.maxtemp_f,
currentLowTemp: data.forecast.forecastday[0].day.mintemp_f,
feelsLike: data.current.feelslike_f,
humidity: data.current.humidity,
rainLevel: data.current.precip_in,
// hourlyTemps is an array, starts from midnight(index 0) and goes every hour until 11 pm(index 23)
hourlyTemps: data.forecast.forecastday[0].hour.map((entry) => {
let epochTime, temp;
[epochTime, temp] = [entry.time_epoch, entry.temp_f];
return [epochTime, temp];
})
};
const daycardInfo = [];
// this for loop triggers and creates an array with all necessary values for the
for (var x in data) {
const fcDayDates = data.forecast.forecastday[x].date_epoch;
const dayInfo = data.forecast.forecastday[x].day;
const dayValues = {
dates: fcDayDates,
message: dayInfo.condition.text,
wicon: dayInfo.condition.icon,
maxTemp: dayInfo.maxtemp_f,
minTemp: dayInfo.mintemp_f,
avgTemp: dayInfo.avgtemp_f
};
// pushes dayValues object into daycardInfor array
daycardInfo.push(dayValues);
};
// this updates the state with the forecast for the city entered
data = {
currentTempInfo: currentTempInfo,
daycardInfo: daycardInfo
};
// this spits out the newly created forecast object
return data;
};
export function Overview() {
const [search, setSearch] = useState(null);
//
const getSearch = (searchedCity) => {
setSearch(searchedCity);
useForecast(search);
};
return (
<div>
<div class='jumbotron' id='heading-title'>
<h1>Welcome to <strong>Weathered</strong>!</h1>
<h3>A Simple Weather Dashboard </h3>
</div>
<div class='container-fluid' id='homepage-skeleton'>
<div class='d-flex' id='center-page'>
<RecentSearches callback={callback}/>
<Hourly />
</div>
</div>
<Fiveday />
</div>
)
};
最近搜索.js
import React, {useState} from 'react';
import './recentSearches.css';
export function RecentSearches({getSearch}) {
const [city, setCity] = useState('');
const [recents, setRecents] = useState([]);
const onSubmit = e => {
e.preventDefault();
if (!city || city === '') {
return;
} else {
addRecent(city);
getSearch(city);
}
}
const onChange = (e) => {
setCity(e.target.value);
};
// function which takes in searched entry and adds entry to recent searches array
function addRecent(newSearch) {
if (recents.includes(newSearch)) {
return;
} else {
setRecents((prev) => [newSearch, ...prev]);
}
// clear our search bar entry
setCity('');
};
const searchAgain = (e) => {
const recent = e.target.innerHTML;
setCity(recent);
}
return (
<section id='search-bar-recents'>
<h3 class='m-3'>Recents </h3>
<div id='insert-recent-buttons'>{recents.map((entry, index) => <h5 key={index} onClick={searchAgain} value={entry}>{entry}</h5>)}</div>
<form onSubmit={onSubmit} class="m-3">
<label className="form-label" for="search-bar">Search A City</label><br/>
<input className='form-text' id="search-bar" type='text' value={city} placeholder="Las Vegas, etc." onChange={onChange}/>
<input className='form-button' id='search-button' type='submit' value='Search'/>
</form>
</section>
)
};
任何幫助/提示/注釋表示贊賞! 謝謝!
rules-of-hooks lint 插件使用命名約定來確定哪些函數是自定義鈎子。 由於您的 function 以use
開頭,因此 lint 插件假定它是一個鈎子,因此它必須遵循鈎子的規則。 但是你的 function 沒有使用任何 react 的內置鈎子,也沒有調用任何自定義鈎子,所以它實際上不需要遵循鈎子的規則。
修復只是重命名 function,所以 lint 插件不認為它是自定義鈎子。 也許fetchForecast
。
const fetchForecast = async (city) => {
// const api_key = process.env.API_KEY;
const api_key = '2220f5a5d83243938f764759211310';
var BASE_URL = `http://api.weatherapi.com/v1/forecast.json?key=${api_key}&q=${city}&days=3&aqi=no&alerts=no`;
// ...
}
// Used like:
const getSearch = async (searchedCity) => {
setSearch(searchedCity);
const forecast = await fetchForecast(searchedCity);
};
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.