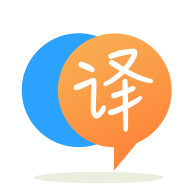
[英]mongooseError: Operation `chatbots.findOne()` buffering timed out after 10000ms at Timeout.<anonymous>
[英]Not able to get response , showing Operation `users.findOne()` buffering timed out after 10000ms`
我在發出 POST 登錄請求時遇到錯誤。
我在這里Netlify 上部署了前端,在這里Heroku 上部署了后端。 這是我的后端日志https://dashboard.heroku.com/apps/my-notebook-mohit/logs 。
我正進入(狀態
`users.findOne()` buffering timed out after 10000ms
在 heroku 日志中找不到原因。
登錄頁面.js
import React, { useState } from "react";
import { useHistory } from "react-router-dom";
import imgpath from "../assets/notepic.jpg";
import { motion } from "framer-motion";
const Login = (props) => {
let history = useHistory();
let port = process.env.PORT;
....
const handleSubmit = async (e) => {
e.preventDefault();
const response = await fetch(`https://my-notebook-mohit.herokuapp.com:/api/auth/login`, {
method: "POST",
headers: {
"Content-Type": "application/json",
},
body: JSON.stringify({
email: credentials.email,
password: credentials.password,
}),
});
const json = await response.json();
if (json.success === true) {
//storing the authtoken
localStorage.setItem("token", json.authToken);
props.showAlert("User logged in successfully", "info");
history.push("/");
} else {
props.showAlert("invalid Credentials", "danger");
}
console.log(json);
};
return (
.....
<form onSubmit={handleSubmit} className="login-form">
.....
....
</form>
...
...
};
export default Login;
auth.js (見路線2)
const express = require("express");
const { body, validationResult } = require("express-validator");
const router = express.Router();
const User = require("../models/User");
const bcrypt = require("bcryptjs");
const jwt = require("jsonwebtoken");
const JWT_SECRET = "mohitisagood$boy";
const fecthUser = require("../middleware/fetchUser");
//ROUTE 1 :Creating a User :POST - "/api/auth/createuser"
router.post(
"/createuser",
[
body("name", "Name must have at least 3 characters").isLength({ min: 3 }),
body("email", "Enter a valid email").isEmail(),
],
async (req, res) => {
let success = false;
//If there are errors, then return bad request + Errors
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({success, errors: errors.array() });
}
try {
//Check whether email exists
let user = await User.findOne({ email: req.body.email });
//console.log("user.nemail = " + user);
if (user) {
//console.log(user.email);
return res.status(400).json({success, error: "Email Already Taken" });
}
//hashing the password here
const salt = await bcrypt.genSalt(10);
const secPass = await bcrypt.hash(req.body.password, salt);
//user is created
user = await User.create({
name: req.body.name,
email: req.body.email,
password: secPass,
});
//passing the id as data to make jwt token
const data = {
user: {
id: user.id,
},
};
const authToken = jwt.sign(data, JWT_SECRET);
//console.log(authToken)
success = true;
//res.json(user);
res.json({success, authToken });
} catch (error) {
console.log(error.message);
res.status(500).send("internal server error");
}
}
);
//ROUTE 2 :Authenticating a User :POST - "/api/auth/login"
router.post(
"/login",
body("email", "Enter a valid email").isEmail(),
async (req, res) => {
//If there are errors, then return bad request + Errors
const errors = validationResult(req);
if (!errors.isEmpty()) {
return res.status(400).json({ errors: errors.array() });
}
const { email, password } = req.body;
let success = false;
try {
let user = await User.findOne({ email });
// console.log("user - "+user)
if (!user) {
res.status(400).json({success,error:"Login with correct credentials!"});
}
//compare the pwd bw 'hash of pwd entered' & 'hash from your pwdDB'
const passwordCompare = await bcrypt.compare(password, user.password);
if (!passwordCompare) {
res.status(400).json({success,error:"Login with correct credentials!"});
}
//nodemon crashes whenever PASSWORD === NULL
const payload = {
user: {
id: user.id,
},
};
const authToken = jwt.sign(payload, JWT_SECRET);
success = true;
res.json({ success, authToken });
} catch (error) {
console.log(error.message);
res.status(500).send("Internal Server Error");
}
}
);
//ROUTE 3 :Get Loggedin user details :GET - "/api/auth/getUser" LOGIN REQUIRED;
router.get("/getUser", fecthUser, async (req, res) => {
try {
const userid = req.user.id; //gets userid from fetchUser middleware fn
let user = await User.findById(userid);
res.send(user);
} catch (error) {
console.log(error.message);
res.status(500).send("Internal Server Error");
}
});
module.exports = router;
附上錯誤截圖
用戶模式
const mongoose = require("mongoose");
const { Schema } = mongoose;
const UserSchema = new Schema({
name: {
type: String,
required: true,
},
email: {
type: String,
required: true,
},
password: {
type: String,
required: true,
validate(value) {
if(value.length < 5) {
throw new Error( "Minimum length is 5 characters");
}
else if (!value.match(/\d/) || !value.match(/[a-zA-Z]/) ) {
throw new Error(
"Password must contain at least one letter and one number"
);
}
}
},
date: {
type: Date,
default: Date.now,
},
});
const User = mongoose.model("users", UserSchema);
module.exports = User;
來自 heroku 的日志
2022-01-19T13:53:34.121500+00:00 heroku[web.1]: Starting process with command `npm start`
2022-01-19T13:53:35.255548+00:00 app[web.1]:
2022-01-19T13:53:35.255561+00:00 app[web.1]: > my-notebook-backend@1.0.0 start
2022-01-19T13:53:35.255561+00:00 app[web.1]: > node index.js
2022-01-19T13:53:35.255562+00:00 app[web.1]:
2022-01-19T13:53:35.891508+00:00 heroku[web.1]: State changed from starting to up
2022-01-19T13:53:36.331033+00:00 heroku[router]: at=info method=GET path="/" host=my-notebook-mohit.herokuapp.com request_id=1f84a49c-8785-4483-a148-1ee8e2d02733 fwd="116.179.32.83" dyno=web.1 connect=0ms service=6ms status=404 bytes=415 protocol=http
2022-01-19T13:53:35.670089+00:00 app[web.1]: my-notebook backend listening at http://localhost:28800
2022-01-19T13:54:05.041186+00:00 heroku[router]: at=info method=OPTIONS path="/api/auth/login" host=my-notebook-mohit.herokuapp.com request_id=ce943a7c-d99d-41a9-965f-7d9f420c0abc fwd="157.34.168.68" dyno=web.1 connect=0ms service=3ms status=204 bytes=301 protocol=https
2022-01-19T13:54:05.563326+00:00 app[web.1]: Connected to Mongo Successfully!
2022-01-19T13:54:15.670120+00:00 heroku[router]: at=info method=POST path="/api/auth/login" host=my-notebook-mohit.herokuapp.com request_id=9527d47f-e054-4f83-bb95-7e99582dab31 fwd="157.34.168.68" dyno=web.1 connect=0ms service=10030ms status=500 bytes=272 protocol=https
2022-01-19T13:54:15.666381+00:00 app[web.1]: Operation `users.findOne()` buffering timed out after 10000ms
2022-01-19T13:54:17.866396+00:00 app[web.1]: Operation `users.findOne()` buffering timed out after 10000ms
2022-01-19T13:54:17.868120+00:00 heroku[router]: at=info method=POST path="/api/auth/login" host=my-notebook-mohit.herokuapp.com request_id=4e9561f7-1050-4afb-9f6b-bf04c876b858 fwd="157.34.168.68" dyno=web.1 connect=0ms service=10007ms status=500 bytes=272 protocol=https
當您的數據庫未連接時,會發生這種類型的日志,因此請檢查以下事項:
mongoose.connect(......)
代碼加載我遇到了這個錯誤,經過幾次搜索,這是因為數據庫有問題。
對於那些使用 MongoDB 的人
檢查mongoDB左側的.network訪問部分。添加您的IP地址。 對我來說,我只是在測試,所以我允許從任何地方訪問。 (我不建議這樣做)。
試一試。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.