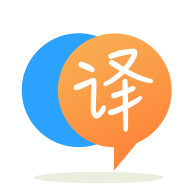
[英]How does one call an overloaded method from a child class from a parent class variable in C++
[英]How to call child class method from parent class in c++
是否可以從父 class 訪問子 class 方法以及如何訪問? 例如我有這個代碼:
城市.cc:
City::City(unsigned int M, unsigned int N)
{
this->M = M;
this->N = N;
for(unsigned int i = 0; i < M; i++)
{
for(unsigned int j = 0; j < N; j++)
{
Cell cell = Cell();
matrix[i][j] = &cell;
}
}
}
int City::getResidentCapacity()
{
int total_capacity = 0;
for(unsigned int i = 0; i < M; i++)
{
for(unsigned int j = 0; j < N; j++)
{
if(matrix[i][j]->selfType() == "Residential")
{
total_capacity += matrix[i][j]->getResidentCapacity();
}
}
}
return total_capacity;
}
單元格.hh:
#pragma once
#include <string>
using namespace std;
class Cell
{
private:
float price;
float electricity;
public:
Cell(float price, float electricity);
Cell();
float getElectricity();
float getPrice();
virtual string selfType();
};
細胞.cc:
#include "Cell.hh"
Cell::Cell(float price, float electricity) : price(price), electricity(electricity) {}
Cell::Cell() : price(0), electricity(0) {}
float Cell::getElectricity()
{
return electricity;
}
float Cell::getPrice()
{
return price;
}
住宅.hh:
#pragma once
#include "Cell.hh"
class Residential : public Cell
{
private:
float price = 1;
float electricity = 1;
int residentCapacity;
public:
Residential(float price, float electricity) : Cell(price, electricity) {}
int getResidentCapacity();
string selfType();
};
住宅.cc:
#include "Residential.hh"
string Residential::selfType()
{
return "Residential";
}
int Residential::getResidentCapacity()
{
return residentCapacity;
}
當我嘗試運行代碼時,出現以下錯誤:
City.cc:在成員 function 'int City::getResidentCapacity()':City.cc:88:38:錯誤:'class Cell' 沒有名為'getResidentCapacity'的成員 88 | 總容量 += 矩陣[i][j]->getResidentCapacity();
我該如何解決?
鑒於 class Fruit
:
class Fruit
{
public:
virtual void print_name();
};
還有一個孩子:
class Orange : public Fruit
{
public:
void print_name()
{ std::cout << "Orange\n"; }
bool is_citrus() const { return true; }
};
如果我們希望父 class Fruit
調用Orange::is_citrus
,那將無法正常工作,因為父不知道是否有任何子,但也不知道子的任何方法。
因此,一種解決方案是在父 class 中創建一個虛擬 function ,子 class 可以實現:
class Fruit
{
public:
virtual bool has_a_peel() = 0;
virtual void print_name();
virtual void details()
{
if (has_a_peel())
{
std::cout << "peel the fruit before eating.\n";
}
}
};
class Orange : public Fruit
{
public:
void print_name()
{ std::cout << "Orange\n"; }
bool is_citrus() const { return true; }
bool has_a_peel()
{ return true; }
};
在上面的代碼中,Parent class Fruit
可以調用 child class has_a_peel
。
提醒:使用這種技術,所有子類都必須實現has_a_peel
class。 您可以從has_a_peel()
方法中刪除“= 0”,這意味着並非所有孩子都需要實現它。 但如果孩子這樣做了怎么辦?
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.