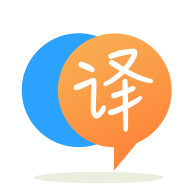
[英]C++ NetBeans error: expected unqualified-id before 'int'
[英]Error: " expected unqualified-id before 'int' ", array, argument, function,
我對 C++ 還很陌生,目前正在通過認證來學習這種語言。 我以前只使用過Python等語言。
我發現了類似的帖子,但沒有一個與我的代碼有關。
我有以下代碼來創建一個十六進制游戲。 我正在嘗試使用簡單的 function 來在玩家每次移動時顯示棋盤。
一開始我盡量保持代碼盡可能簡單(限制指針和庫的使用)。
我有這個錯誤: hex_game.cpp:9:47: error: expected unqualified-id before 'int' 9 | void display_current_array(array[size][size], int size){ |
以下是我的代碼,希望有人可以幫助:
#include <iostream>
#include <stdlib.h>
using namespace std;
#include <vector>
#include <array>
// a void function to display the array after every moove
void display_current_array(array[size][size], int size){
//s=arr.size();
for (int i = 0; i < size; i++)
{
for (int j = 0; j < size; j++)
{
cout<<array[i][j]<<endl;
}
}
}
int main(){
// the HEX game is a game of size cases represented by an array either filled with a color;
// int size =11; //as asked but we can play on a differnt board
int size;
// ask the player to give a board size
cout << "What is the size of your Hex Board ? ";
cin>> size;
// create the array to represent the board
int array[size][size];
// the player will choose colors that we will store as an enum type
enum colors {BLUE, RED};
// initialize the array: all positions are 0
for (int i = 0; i < size; i++)
{
for (int j = 0; j < size; j++)
{
array[i][j]=0;
}
}
display_current_array(array, size);
}
在標准 C++中,數組的大小必須是編譯時間常數。 所以當你寫:
int size;
cin>> size;
int array[size][size]; //NOT STANDARD C++
語句array[size][size];
不是標准的 c++。
第二,當你寫道:
void display_current_array(array[size][size], int size){
//...
}
請注意,在 function display_current_array
的第一個參數中,您沒有指定數組包含的元素類型。 所以這將導致你得到的錯誤。
避免這些並發症的更好方法是使用像std::vector
這樣的動態大小的容器,如下所示:
#include <iostream>
#include <vector>
// a void function to display the vector after every moove
void display_current_array(const std::vector<std::vector<int>> &vec){//note the vector is passed by reference to avoid copying
for(auto &row: vec)
{
for(auto &col: row)
{
std::cout << col ;
}
std::cout<<std::endl;
}
}
int main(){
int size;
// ask the player to give a board size
std::cout << "What is the size of your Hex Board ? ";
std::cin>> size;
// create the 2D STD::VECTOR with size rows and size columns to represent the board
std::vector<std::vector<int>> vec(size, std::vector<int>(size));
// the player will choose colors that we will store as an enum type
enum colors {BLUE, RED};
//NO NEED TO INITIALIZE EACH INDIVIDUAL CELL IN THE 2D VECTOR SINCE THEY ARE ALREADY INITIALIED TO 0
display_current_array(vec);
}
注意:
以上程序的output可以看這里。
你不是在問問題,只是提到一個錯誤。
因此,這是避免該錯誤的方法:
void display_current_array(int array[size][size], int size)
{}
當然,它會給您帶來另一個錯誤:
error: 'size' was not declared in this scope
void display_current_array(int array[size][size], int size)
這就是 πάντα ῥεῖ 在評論中所暗示的。
所以,避免可變大小數組的嘗試。
void display_current_array(int array[][], int size)
{}
這讓你
main.cpp:5:40: error: declaration of 'array' as multidimensional array must have bounds for all dimensions except the first
void display_current_array(int array[][], int size)
那么......再次插入一個size
? 不,出於同樣的原因,它被刪除了。 您可以擺脫該錯誤:
void display_current_array(int array[][42], int size)
{}
但可以肯定的是,這正是您不想要的(恆定大小)。
因此,我們得到了 BoP 的評論,即您應該(或者甚至嘗試)使用std::array
。 一旦明確決定(與嘗試 C 風格的可變長度 arrays 相比,這在 C++ 中是不允許的),只需查找詳細信息的教程即可。
編寫此代碼的更好方法是使用 STL 向量容器。 如果你想用 arrays 來做,你需要了解指針。
#include <iostream>
#include <stdlib.h>
#include <vector>
using namespace std;
// a void function to display the array after every moove
void display_current_array(vector<vector<int>> &array, int size){ // & means pass-by-reference
//s=arr.size();
for (int i = 0; i < size; i++)
{
for (int j = 0; j < size; j++)
{
cout << array[i][j] << endl;
}
}
}
int main(){
// the HEX game is a game of size cases represented by an array either filled with a color;
// int size =11; //as asked but we can play on a differnt board
int size;
// ask the player to give a board size
cout << "What is the size of your Hex Board ? ";
cin >> size;
// create the array to represent the board
vector<vector<int>> array;
// the player will choose colors that we will store as an enum type
enum colors {BLUE, RED};
// initialize the array: all positions are 0
for (int i = 0; i < size; i++)
{
vector<int> row;
for (int j = 0; j < size; j++)
{
row.push_back(0);
}
array.push_back(row);
}
display_current_array(array, size);
}
在這里,我使用 & 操作數通過引用傳遞了向量。
void display_current_array(vector<vector<int>> &array, int size)
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.