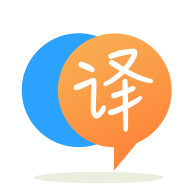
[英]Why am I getting an error : TypeError: Cannot read property 'map' of undefined?
[英]why am i getting a TypeError of undefined map?
我正在做一個 React 項目,我收到了未定義的 map 的錯誤。 我們目前也在做一些測試,我們是 mocking 一個包含詳細信息的數組。 我不知道這是語法錯誤還是我沒有正確傳遞道具。
這是我的代碼
事件.js
import Button from 'react-bootstrap/Button'
class Event extends Component {
state = {
collapsed: true,
}
handleClick = () => {
this.setState({
collapsed: !this.state.collapsed,
});
};
render() {
const { event } = this.props;
const { collapsed } = this.state;
return (
<div className="event">
<h2 className="summary">{event.summary}</h2>
<p className="start-date">
{event.start.dateTime}
</p>
<p className="end-date">
{event.end.dateTime}
</p>
<p className="location">
{event.location}
</p>
<Button variant="outline-info"
className={`${collapsed ? "show" : "hide"}-details`}
onClick={this.handleClick}
>
{collapsed ? "Show Details" : "Hide Details"}
</Button>
{!collapsed &&
<div className={`extra-details ${this.state.collapsed ? "hide" : "show"}`}>
<p className="event-description">{event.description}</p>
</div>
}
</div>
)
}
}
export default Event;
事件列表.js:
import Button from 'react-bootstrap/Button'
class Event extends Component {
state = {
collapsed: true,
}
handleClick = () => {
this.setState({
collapsed: !this.state.collapsed,
});
};
render() {
const { event } = this.props;
const { collapsed } = this.state;
return (
<div className="event">
<h2 className="summary">{event.summary}</h2>
<p className="start-date">
{event.start.dateTime}
</p>
<p className="end-date">
{event.end.dateTime}
</p>
<p className="location">
{event.location}
</p>
<Button variant="outline-info"
className={`${collapsed ? "show" : "hide"}-details`}
onClick={this.handleClick}
>
{collapsed ? "Show Details" : "Hide Details"}
</Button>
{!collapsed &&
<div className={`extra-details ${this.state.collapsed ? "hide" : "show"}`}>
<p className="event-description">{event.description}</p>
</div>
}
</div>
)
}
}
export default Event;
應用程序.js
import './App.css';
import EventList from './EventList';
import CitySearch from './CitySearch';
import NumberOfEvents from './NumberOfEvents';
function App() {
return (
<div className="App">
<CitySearch />
<NumberOfEvents />
<EventList />
</div>
);
}
export default App;
錯誤出現在 EventList.js 文件中enter image description here
const mockData = [
{
"kind": "calendar#event",
"etag": "\"3181161784712000\"",
"id": "4eahs9ghkhrvkld72hogu9ph3e_20200519T140000Z",
"status": "confirmed",
"htmlLink": "https://www.google.com/calendar/event?eid=NGVhaHM5Z2hraHJ2a2xkNzJob2d1OXBoM2VfMjAyMDA1MTlUMTQwMDAwWiBmdWxsc3RhY2t3ZWJkZXZAY2FyZWVyZm91bmRyeS5jb20",
"created": "2020-05-19T19:17:46.000Z",
"updated": "2020-05-27T12:01:32.356Z",
"summary": "Learn JavaScript",
"description": "Have you wondered how you can ask Google to show you the list of the top ten must-see places in London? And how Google presents you the list? How can you submit the details of an application? Well, JavaScript is doing these. :) \n\nJavascript offers interactivity to a dull, static website. Come, learn JavaScript with us and make those beautiful websites.",
"location": "London, UK",
"creator": {
"email": "fullstackwebdev@careerfoundry.com",
"self": true
},
"organizer": {
"email": "fullstackwebdev@careerfoundry.com",
"self": true
},
"start": {
"dateTime": "2020-05-19T16:00:00+02:00",
"timeZone": "Europe/Berlin"
},
"end": {
"dateTime": "2020-05-19T17:00:00+02:00",
"timeZone": "Europe/Berlin"
},
"recurringEventId": "4eahs9ghkhrvkld72hogu9ph3e",
"originalStartTime": {
"dateTime": "2020-05-19T16:00:00+02:00",
"timeZone": "Europe/Berlin"
},
"iCalUID": "4eahs9ghkhrvkld72hogu9ph3e@google.com",
"sequence": 0,
"reminders": {
"useDefault": true
},
"eventType": "default"
},
{
"kind": "calendar#event",
"etag": "\"3181159875584000\"",
"id": "3qtd6uscq4tsi6gc7nmmtpqlct_20200520T120000Z",
"status": "confirmed",
"htmlLink": "https://www.google.com/calendar/event?eid=M3F0ZDZ1c2NxNHRzaTZnYzdubW10cHFsY3RfMjAyMDA1MjBUMTIwMDAwWiBmdWxsc3RhY2t3ZWJkZXZAY2FyZWVyZm91bmRyeS5jb20",
"created": "2020-05-19T19:14:30.000Z",
"updated": "2020-05-27T11:45:37.792Z",
"summary": "React is Fun",
"description": "Love HTML, CSS, and JS? Want to become a cool front-end developer? \n\nReact is one of the most popular front-end frameworks. There is a huge number of job openings for React developers in most cities. \n\nJoin us in our free React training sessions and give your career a new direction. ",
"location": "Berlin, Germany",
"creator": {
"email": "fullstackwebdev@careerfoundry.com",
"self": true
},
"organizer": {
"email": "fullstackwebdev@careerfoundry.com",
"self": true
},
"start": {
"dateTime": "2020-05-20T14:00:00+02:00",
"timeZone": "Europe/Berlin"
},
"end": {
"dateTime": "2020-05-20T15:00:00+02:00",
"timeZone": "Europe/Berlin"
},
"recurringEventId": "3qtd6uscq4tsi6gc7nmmtpqlct",
"originalStartTime": {
"dateTime": "2020-05-20T14:00:00+02:00",
"timeZone": "Europe/Berlin"
},
"iCalUID": "3qtd6uscq4tsi6gc7nmmtpqlct@google.com",
"sequence": 0,
"reminders": {
"useDefault": true
},
"eventType": "default"
}
]
export { mockData };
```
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.