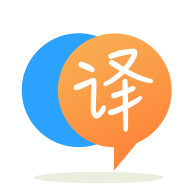
[英]Iam using android studio and firestore. In my application when admin adds details it is successfully added but it doesn't shows when user logins
[英]How to check when the user logins for the first time Android Studio SQLite
我有一個注冊 Activirty,我可以在其中創建各種用戶,並且用戶名和密碼保存在數據庫中。 當我登錄時,它會將我重定向到一個活動。 我的需要是,如果這是我第一次登錄,它應該加載 CreateProfile Activity,如果不是,它應該加載 Profile Activity。 現在我用 Toast 消息制作它,但它總是加載 toast 消息“正常登錄”。
這是 DBHelper:
package com.example.sqlite;
import android.content.ContentValues;
import android.content.Context;
import android.database.Cursor;
import android.database.sqlite.SQLiteDatabase;
import android.database.sqlite.SQLiteOpenHelper;
import android.widget.Toast;
import androidx.annotation.Nullable;
public class DBHelper extends SQLiteOpenHelper {
private Context context;
private static final String DATABASE_NAME = "Database.db";
private static final int DATABASE_VERSION = 1;
// USERS TABLE
private static final String TABLE_USERS = "USERS";
private static final String COLUMN_ID = "user_id";
private static final String COLUMN_USERNAME = "username";
private static final String COLUMN_PASSWORD = "password";
private static final String COLUMN_FIRST_TIME = "first_time";
// PROFILE TABLE
private static final String TABLE_PROFILE = "PROFILE";
//private static final String COLUMN_PROF_ID = "profile_id";
private static final String COLUMN_FIRSTNAME = "firstname";
private static final String COLUMN_LASTNAME = "lastname";
private static final String COLUMN_AGE = "age";
private static final String COLUMN_WEIGHT = "weight";
private static final String COLUMN_HEIGHT = "height";
// private static final String COLUMN_GENDER = "gender";
public DBHelper(@Nullable Context context) {
super(context, DATABASE_NAME, null, DATABASE_VERSION);
this.context = context;
}
@Override
public void onCreate(SQLiteDatabase db) {
//String usersQuery = "CREATE TABLE " + TABLE_USERS + " (" + COLUMN_ID + " INTEGER PRIMARY KEY AUTOINCREMENT, " + COLUMN_USERNAME + " TEXT, " + COLUMN_PASSWORD + " INTEGER " + ")";
//String profileQuery = "CREATE TABLE " + TABLE_PROFILE + " (" + COLUMN_ID + " INTEGER , " + COLUMN_FIRST_NAME + " TEXT, " + COLUMN_LAST_NAME + " TEXT, " + COLUMN_AGE + " INTEGER, " + COLUMN_WEIGHT + " INTEGER, " + COLUMN_HEIGHT + " INTEGER " + ")";
String usersQuery = String.format("CREATE TABLE IF NOT EXISTS %s ( %s INTEGER PRIMARY KEY AUTOINCREMENT, %s TEXT, %s INTEGER, %s INTEGER DEFAULT 1 )",TABLE_USERS,COLUMN_ID,COLUMN_USERNAME,COLUMN_PASSWORD,COLUMN_FIRST_TIME);
String profileQuery = String.format("CREATE TABLE IF NOT EXISTS %s ( %s TEXT, %s TEXT, %s INTEGER, %s INTEGER, %s INTEGER)",TABLE_PROFILE,COLUMN_FIRSTNAME,COLUMN_LASTNAME, COLUMN_AGE, COLUMN_WEIGHT, COLUMN_HEIGHT);
db.execSQL(usersQuery);
db.execSQL(profileQuery);
}
@Override
public void onUpgrade(SQLiteDatabase db, int i, int i1) {
db.execSQL("DROP TABLE IF EXISTS " + TABLE_USERS);
db.execSQL("DROP TABLE IF EXISTS " + TABLE_PROFILE);
onCreate(db);
}
public Boolean registerUser(String username, String password){
SQLiteDatabase db = this.getWritableDatabase();
ContentValues cv = new ContentValues();
cv.put(COLUMN_USERNAME, username);
cv.put(COLUMN_PASSWORD, password);
long result = db.insert(TABLE_USERS, null, cv);
db.close();
if (result != -1)
{
Toast.makeText(context, "Registration Successful", Toast.LENGTH_SHORT).show();
return true;
}
else
return false;
}
Cursor readAllData() {
String query = "SELECT * FROM " + TABLE_USERS;
SQLiteDatabase db = this.getReadableDatabase();
Cursor cursor = null;
if (db != null) {
cursor = db.rawQuery(query, null);
}
return cursor;
}
// function that checks if user exists in the database
public Boolean checkUsername(String username)
{
SQLiteDatabase myDB = this.getWritableDatabase();
Cursor cursor = myDB.rawQuery("select * from users where username = ?", new String[] {username}); // if the data exists the cursor will have some data
// check if cursor has any data or not
if (cursor.getCount() > 0)
{
return true; // cursor has some data (user exists)
}
else
{
return false;
}
}
public Boolean checkUsernamePassword (String username, String password) // check the username and password
{
SQLiteDatabase myDB = this.getWritableDatabase();
Cursor cursor = myDB.rawQuery("select * from users where username = ? and password = ?", new String[] {username, password});
if (cursor.getCount() > 0) // if the data exists then return true
{
return true;
}
else
{
return false;
}
}
public Boolean insertProfileData(String user_id, String firstname, String lastname, String age, String weight, String height) {
SQLiteDatabase db = this.getWritableDatabase();
ContentValues cv = new ContentValues();
cv.put(COLUMN_ID, user_id);
cv.put(COLUMN_FIRSTNAME, firstname);
cv.put(COLUMN_LASTNAME, lastname);
cv.put(COLUMN_AGE, age);
cv.put(COLUMN_WEIGHT, weight);
cv.put(COLUMN_HEIGHT, height);
int cursor= (int) db.insert(TABLE_PROFILE, null, cv);
db.close();
if (cursor > 0)
return true;
else
return false;
}
Cursor readProfileInfo() {
String query = "SELECT * FROM " + TABLE_PROFILE;
SQLiteDatabase db = this.getReadableDatabase();
Cursor cursor = null;
if (db != null) {
cursor = db.rawQuery(query, null);
}
return cursor;
}
public int fetch_username(String username) {
SQLiteDatabase db = this.getWritableDatabase();
Cursor cursor = db.rawQuery("select * from users where username = '"+username +"'" , null);
if(cursor.moveToFirst()){
do{
return cursor.getInt(0);
}while (cursor.moveToNext());
}
else
return -1;
}
public String fetch_profileData(String id) {
SQLiteDatabase db = this.getWritableDatabase();
Cursor cursor = db.rawQuery("select * from PROFILE where user_id = '"+id +"'" , null);
if(cursor.moveToFirst()){
do{
return cursor.getString(1);
}while (cursor.moveToNext());
}
else
return null;
}
public boolean check_first_time(String user_id) {
SQLiteDatabase db = this.getWritableDatabase();
Cursor cursor = db.rawQuery("select * from users where user_id = '"+user_id +"'" , null);
if(cursor.moveToFirst()){
do{
int value = cursor.getInt(2);
if(value == 1){
return true;
}
else{
return false;
}
}while (cursor.moveToNext());
}
else
return false;
}
public boolean update_first_time_status(String user_id) {
SQLiteDatabase db = this.getWritableDatabase();
ContentValues values = new ContentValues();
values.put(COLUMN_FIRST_TIME,0);
int cursor= db.update(TABLE_USERS, values, "user_id=?", new String[] {String.valueOf(user_id)});
db.close();
if (cursor > 0)
return true;
else
return false;
}
}
登錄活動:
package com.example.sqlite;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.content.SharedPreferences;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
public class LoginActivity extends AppCompatActivity {
TextView signUp;
EditText username, password;
Button loginBtn;
DBHelper myDB;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_login);
myDB = new DBHelper(LoginActivity.this);
signUp = (TextView) findViewById(R.id.tv_signUp);
username = (EditText) findViewById(R.id.tv_fullname);
password = (EditText) findViewById(R.id.tv_age);
loginBtn = (Button) findViewById(R.id.loginBtn);
signUp.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
startActivity(new Intent(LoginActivity.this, RegisterActivity.class));
}
});
loginBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
String user = username.getText().toString().trim();
String pass = password.getText().toString().trim();
if (user.equals("") || pass.equals(""))
{
Toast.makeText(LoginActivity.this, "Fill all the fields", Toast.LENGTH_SHORT).show();
}
else
{
// check username and password if they exist/equal in the database - if yes login to the app
Boolean userPassCheckResult = myDB.checkUsernamePassword(user, pass);
if (userPassCheckResult)
{
int user_id = myDB.fetch_username(user);
if(myDB.check_first_time(String.valueOf(user_id)))
{
Toast.makeText(LoginActivity.this, "! FIRST LOGIN !", Toast.LENGTH_LONG).show();
//Intent intent = new Intent(LoginActivity.this, CreateProfileActivity.class);
//startActivity(intent);
myDB.update_first_time_status(String.valueOf(user_id));
}
else
{
Toast.makeText(LoginActivity.this, "normal login..", Toast.LENGTH_LONG).show();
//Intent intent = new Intent(LoginActivity.this, ProfileActivity.class);
//startActivity(intent);
}
}
else
{
Toast.makeText(LoginActivity.this, "Invalid Credentials", Toast.LENGTH_SHORT).show();
}
}
}
});
}
}
如果您需要 RegisterActivity:
package com.example.sqlite;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class RegisterActivity extends AppCompatActivity {
TextView tv_signIn;
EditText username, password, repeatPass;
Button registerBtn;
DBHelper db;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_register);
username = findViewById(R.id.username_input);
password = findViewById(R.id.password_input);
repeatPass = findViewById(R.id.repeatPass);
registerBtn = findViewById(R.id.signUpBtn);
tv_signIn = findViewById(R.id.tv_signIn);
//DBHelper myDB = new DBHelper(this);
tv_signIn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(RegisterActivity.this, LoginActivity.class);
startActivity(intent);
}
});
registerBtn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
DBHelper myDB = new DBHelper(RegisterActivity.this);
String user = username.getText().toString().trim();
String pass = password.getText().toString().trim();
String repass = repeatPass.getText().toString().trim();
if (user.isEmpty())
{
username.setError("Username is required!");
username.requestFocus();
return;
}
if (pass.isEmpty())
{
password.setError("Password is required!");
password.requestFocus();
return;
}
if (pass.length() < 6)
{
password.setError("Min password length should be 6 characters!");
password.requestFocus();
return;
}
else if (pass.equals(repass))
{
Boolean usercheckResult = myDB.checkUsername(user);
if (!usercheckResult) {
Boolean checkInsertData = myDB.registerUser(user, pass); // add user - Register
if (checkInsertData) {
Toast.makeText(RegisterActivity.this, "Registration Successful", Toast.LENGTH_SHORT).show();
Intent intent = new Intent(RegisterActivity.this, com.example.sqlite.LoginActivity.class);
startActivity(intent);
} else {
Toast.makeText(RegisterActivity.this, "Failed", Toast.LENGTH_SHORT).show();
}
} else {
Toast.makeText(RegisterActivity.this, "User already exists. \n Please Sign In", Toast.LENGTH_SHORT).show();
}
} else {
Toast.makeText(RegisterActivity.this, "Passwords do not Match!", Toast.LENGTH_SHORT).show();
repeatPass.requestFocus();
return;
}
}
});
}
}
你有:-
Cursor cursor = db.rawQuery("select * from users where user_id = '"+user_id +"'" , null);
if(cursor.moveToFirst()){
do{
int value = cursor.getInt(2);
if(value == 1){
return true;
}
else{
return false;
}
}while (cursor.moveToNext());
}
SQL SELECT * FROM users....
將返回與 users 表 (4) 中的列一樣多的列,其中getInt(2)
將檢索第三列(密碼)是每一個可能是 1嗎? (修辭)
我相信你想要getInt(3) 。 但是,最好不要硬編碼偏移量並使用getColumnIndex()
方法通過其名稱獲取列的索引。
如果通過user_id搜索,因為它是一個主鍵,那么它只能返回一個1。所以你不需要循環通過cursor。
所以你可以: -
public boolean check_first_time(String user_id) {
SQLiteDatabase db = this.getWritableDatabase();
boolean rv = false;
Cursor cursor = db.rawQuery("SELECT * from users where user_id=?" ,new String[]{user_id});
if(cursor.moveToFirst()){
rv = cursor.getInt(cursor.getColumnIndex("first_time")) != 1
}
cursor.close();
return rv;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.