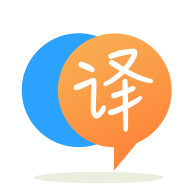
[英]When i run this program it does not print the required output given by the called function for checking for balanced parenthesis?
[英]Generate all balanced parenthesis for a given N
// Header files
#include<iostream>
#include<vector>
#include<stack>
using namespace std;
// This function checks for well formedness of the passed string.
bool valid(string s)
{
stack<char> st;
for(int i=0;i<s.length();i++)
{
char x=s[i];
if(x=='(')
{
st.push(x);
continue;
}
if(st.empty())
return false;
st.pop();
}
return st.empty();
}
// This function generates all combination of parenthesis and only checks well formedness of string whose length is equal to n.
void generate(int n,string s)
{
if(s.length()==n)
{
bool balanced = valid(s);
if(balanced)
cout<<s<<endl;
return;
}
generate(n,s+"(");
generate(n,s+")");
}
// Driver code
int main()
{
int n;
cin>>n;
generate(n,"");
return 0;
}
我想要實現的是生成最長為 n 的所有可能的括號。 並檢查大小為 N 的如此形成的字符串的良好形成/平衡。我的程序終止而不打印任何內容。 請幫忙!
例如,對於 n=3,輸入:N = 3 Output:((()))
(()())
(())()
()(())
()()()
順序無所謂。
我的 output 沒有顯示任何內容。
參考: https://practice.geeksforgeeks.org/problems/generate-all-possible-parentheses/1
//c++ program to print all the combinations of
balanced parenthesis.
#include <bits/stdc++.h>
using namespace std;
//function which generates all possible n pairs
of balanced parentheses.
//open : count of the number of open parentheses
used in generating the current string s.
//close : count of the number of closed
parentheses used in generating the current string
s.
//s : currently generated string/
//ans : a vector of strings to store all the
valid parentheses.
void generateParenthesis(int n, int open, int
close, string s, vector<string> &ans){
//if the count of both open and close
parentheses reaches n, it means we have generated
a valid parentheses.
//So, we add the currently generated string s
to the final ans and return.
if(open==n && close==n){
ans.push_back(s);
return;
}
//At any index i in the generation of the
string s, we can put an open parentheses only if
its count until that time is less than n.
if(open<n){
generateParenthesis(n, open+1, close, s+"{",
ans);
}
//At any index i in the generation of the string
s, we can put a closed parentheses only if its
count until that time is less than the count of
open parentheses.
if(close<open){
generateParenthesis(n, open, close+1, s+"}",
ans);
}
}
int main() {
int n = 3;
//vector ans is created to store all the
possible valid combinations of the parentheses.
vector<string> ans;
//initially we are passing the counts of open
and close as 0, and the string s as an empty
string.
generateParenthesis(n,0,0,"",ans);
//Now, here we print out all the combinations.
for(auto s:ans){
cout<<s<<endl;
}
return 0;
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.