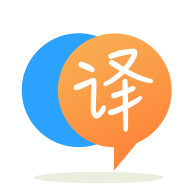
[英]Flutter AspNet Core SocketException (SocketException: OS Error: Connection timed out, errno = 110, address = 192.168.1.87, port = 46186)
[英]Unhandled Exception: SocketException: OS Error: Connection timed out, errno = 110, address = api.generaliot.in, port = 45700 flutter
import 'dart:convert';
import 'dart:io';
import 'package:custom_searchable_dropdown/custom_searchable_dropdown.dart';
import 'package:expandable/expandable.dart';
import 'package:flutter_spinkit/flutter_spinkit.dart';
import 'package:http/http.dart' as http;
import 'package:flutter/material.dart';
import 'package:http/http.dart';
import 'package:shared_preferences/shared_preferences.dart';
import 'useful_screens/classes.dart';
import 'useful_screens/constants.dart';
import 'equipments_and_points_details.dart';
class View_equipments extends StatefulWidget {
const View_equipments({Key? key}) : super(key: key);
@override
_View_equipmentsState createState() => _View_equipmentsState();
}
class _View_equipmentsState extends State<View_equipments> {
List<Department> selected_departments = [];
Map equipments_dept = {};
late int len_map;
late String token;
late DateTime start;
bool processing = true;
void get_departments_list() async {
SharedPreferences prefs = await SharedPreferences.getInstance();
token = prefs.getString('token')!;
var query_params = {'token': token, 'site_id': '2'};
Uri url = Uri.parse('$BASE_URL/companies/sites/departments/list')
.replace(queryParameters: query_params);
Response response = await http.get(
url,
headers: headers,
);
List department_ids = jsonDecode(response.body)["department_ids"];
//getting the list of departments
var url_1 = Uri.parse('${BASE_URL}/companies/sites/departments/info');
Response response_1 = await http.post(url_1,
headers: headers,
body: jsonEncode({'token': token, 'department_ids': department_ids}));
var data = jsonDecode(response_1.body)["departments"];
selected_departments = List.generate(
data.length,
(i) => Department(
id: data[i]['id'], name: data[i]['name'], site: data[i]['site']));
get_equipments();
}
// Future<Map> get_equipments() async {
// Map<String, List<Equipment>> equipments_dept = {};
// SharedPreferences prefs = await SharedPreferences.getInstance();
// String token = prefs.getString('token')!;
// for (int i = 0; i < selected_departments.length; i++) {
// var query_params = {
// 'token': token,
// 'department_id': '${selected_departments[i].id}'
// };
// var url = Uri.parse(
// '${BASE_URL}/companies/sites/departments/equipments/list',
// ).replace(queryParameters: query_params);
// Response response = await http.get(
// // Uri.parse('${BASE_URL}/companies/sites/users/info'),
// url,
// headers: {
// // HttpHeaders.authorizationHeader: "Token $token",
// HttpHeaders.contentTypeHeader: "application/json"
// },
// );
//
// var query_params_1 = {
// 'token': token,
// 'equipment_ids': jsonDecode(response.body)['equipment_ids']
// };
// var url_1 =
// Uri.parse('${BASE_URL}/companies/sites/departments/equipments/info');
// Response response_1 = await http.post(url_1,
// headers: {
// // HttpHeaders.authorizationHeader: "Token $token",
// HttpHeaders.contentTypeHeader: "application/json"
// },
// body: jsonEncode(query_params_1));
// List equipments = jsonDecode(response_1.body)['equipments'];
//
// equipments_dept[selected_departments[i].name] = List.generate(
// equipments.length,
// (i) => Equipment(
// id: equipments[i]['id'],
// name: equipments[i]['name'],
// department: equipments[i]['department'],
// make: equipments[i]['make'],
// model: equipments[i]['model'],
// commision_date: equipments[i]['commision_date'],
// sump_capacity: equipments[i]['sump_capacity'],
// hac_code: equipments[i]['hac_code'],
// longitude: equipments[i]['longitude'],
// latitude: equipments[i]['latitude']),
// );
// }
// //Now we have the equipments with their respective departments
// return equipments_dept;
// }
void get_equipments() async {
SharedPreferences prefs = await SharedPreferences.getInstance();
String token = prefs.getString('token')!;
for (int i = 0; i < selected_departments.length; i++) {
var query_params = {
'token': token,
'department_id': '${selected_departments[i].id}'
};
Response response = await http.get(
Uri.parse(
'${BASE_URL}/companies/sites/departments/equipments/list',
).replace(queryParameters: query_params),
headers: headers);
// print('equipmentids');
// print(response.statusCode);
// print(response.body); //include them in a list and display
var query_params_1 = {
'token': token,
'equipment_ids': jsonDecode(response.body)['equipment_ids']
};
Response response_1 = await http.post(
Uri.parse('${BASE_URL}/companies/sites/departments/equipments/info'),
headers: headers,
body: jsonEncode(query_params_1));
// print(response_1.statusCode);
// print(response_1.body); //include them in a list and display
List equipments = jsonDecode(response_1.body)['equipments'];
equipments_dept[selected_departments[i].name] = List.generate(
equipments.length,
(i) => Equipment(
id: equipments[i]['id'],
name: equipments[i]['name'],
department: equipments[i]['department'],
make: equipments[i]['make'],
model: equipments[i]['model'],
commision_date: equipments[i]['commision_date'],
sump_capacity: equipments[i]['sump_capacity'],
hac_code: equipments[i]['hac_code'],
longitude: equipments[i]['longitude'],
latitude: equipments[i]['latitude']),
);
setState(() {
processing = false;
});
}
//Now we have the equipments with their respective departments
}
@override
Widget build(BuildContext context) {
get_departments_list();
return Scaffold(
appBar: AppBar(
title: Text('Equipments'),
centerTitle: true,
),
body: SingleChildScrollView(
scrollDirection: Axis.vertical,
child: !processing
? Center(
child: SpinKitDoubleBounce(
color: Colors.white,
size: 100,
),
)
: Column(
children: List.generate(
selected_departments.length,
(i) => dept_dropdown(selected_departments[i].name,
equipments_dept[selected_departments[i]], context)),
)));
}
}
Widget dept_dropdown(String dept, List<Equipment>? equipments, context) {
print(dept);
print(equipments);
if (equipments == null) {
print('empty');
return Center(
child: Container(
margin: EdgeInsets.fromLTRB(0, 20, 0, 10),
height: 100,
width: MediaQuery.of(context).size.width * 0.9,
decoration: BoxDecoration(color: Colors.blue),
),
);
}
int len = equipments.length;
print('Hello');
return ExpandableNotifier(
child: Expandable(
collapsed: Row(
children: [
Expanded(
child: ExpandableButton(
child: Container(
// padding: EdgeInsets.symmetric(vertical: 10),
child: Column(
children: [
Row(
mainAxisAlignment: MainAxisAlignment.start,
children: [
Expanded(
child: Text(dept,
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold,
)),
flex: 8,
),
Expanded(
child: Icon(Icons.add),
flex: 4,
),
],
)
],
),
),
),
)
],
),
expanded: Column(
children: [
ExpandableButton(
child: Container(
// padding: EdgeInsets.symmetric(vertical: 10),
child: Column(
children: [
Row(
mainAxisAlignment: MainAxisAlignment.start,
children: [
Expanded(
child: Text(
dept,
style: TextStyle(
fontSize: 20,
fontWeight: FontWeight.bold,
),
),
flex: 8,
),
Expanded(
child: Icon(Icons.minimize_outlined),
flex: 4,
),
],
)
],
),
),
),
Column(
children: List.generate(
len,
(i) => InkWell(
onTap: () {
Navigator.push(context,
MaterialPageRoute(builder: (context) {
return EquipmentPoints(
equipment: equipments[i],
);
}));
}, //Todo Set ontap method
child: Row(
children: [
Container(
height: 100,
padding: EdgeInsets.fromLTRB(20, 7, 0, 7),
decoration: BoxDecoration(
color: Colors.white,
borderRadius: BorderRadius.circular(10)),
child: Column(
children: [
Text('Name: ${equipments[i].name}'),
SizedBox(height: 10),
Text('Make: ${equipments[i].make}'),
SizedBox(height: 10),
Text('Model: ${equipments[i].model}'),
SizedBox(height: 10),
Text(
'Year of Commissioning: ${equipments[i].commision_date}'),
SizedBox(height: 10),
Text(
'Sump Capacity: ${equipments[i].sump_capacity}'),
SizedBox(height: 10),
Text('HAC code: ${equipments[i].hac_code}'),
SizedBox(height: 10),
Text(
'Points: ${equipments[i].name}'), //Todo ask about points
SizedBox(height: 10),
Text(
'Tickets: ${equipments[i].name}'), // Todo Make it dynamic ie if any tickets
SizedBox(height: 10),
Text(
'Last Serviced date: ${equipments[i].name}'), //Todo findout
SizedBox(height: 10),
Text(
'Next Planned date: ${equipments[i].name}'), //Todo findout
SizedBox(height: 10),
],
),
)
],
),
))),
],
),
),
);
}
這是我的代碼,我在其中向 api 發出數據請求並在我的前端展示它。 我面臨以下錯誤。
[錯誤:flutter/lib/ui/ui_dart_state.cc(209)] 未處理的異常:SocketException:OS 錯誤:連接超時,errno = 110,地址 = api.generaliot.in,端口 = 45700 E/flutter (26453): #0 _NativeSocket.startConnect (dart:io-patch/socket_patch.dart:681:35) E/flutter (26453): #1 _RawSocket.startConnect (dart:io-patch/socket_patch.dart:1808:26) E/顫振(26453): #2 RawSocket.startConnect (dart:io-patch/socket_patch.dart:27:23) E/flutter (26453): #3 RawSecureSocket.startConnect (dart:io/secure_socket.dart:237:22) E /flutter (26453): #4 SecureSocket.startConnect (dart:io/secure_socket.dart:60:28) E/flutter (26453): #5 _ConnectionTarget.connect (dart:_http/http_impl.dart:2437:24) E /flutter (26453): #6 _HttpClient._getConnection.connect (dart:_http/http_impl.dart:2808:12) E/flutter (26453): #7 _HttpClient._getConnection (dart:_http/http_impl.dart:2813:12 ) E/flutter (26453): #8 _HttpClient._openUrl ( dart:_http/http_impl.dart:2697:12) E/flutter (26453): #9 _HttpClient.openUrl (dart:_http/http_impl.dart:2569:7) E/flutter (26453): #10 IOClient.send (包:http/src/io_client.dart:35:38) E/flutter (26453): #11 BaseClient._sendUnstreamed (包:http/src/base_client.dart:93:38) E/flutter (26453): #12 BaseClient.post(包:http/src/base_client.dart:32:7)E/flutter (26453): #13 post。 (包:http/http.dart:69:16)E/flutter(26453):#14 _withClient(包:http/http.dart:164:20)E/flutter(26453):#15 post(包:http /http.dart:68:5) E/flutter (26453): #16 _View_equipmentsState.get_equipments (package:ncair_app/equipments.dart:133:40) E/flutter (26453):
任何解決方案
我使用 https 而不是 http 解決了這個問題。希望這可以節省您的大量時間。
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.