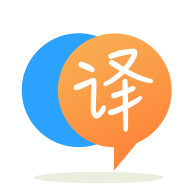
[英]How to check if username already exist in the sql database before one can create new account using java
[英]How to check Username is already exist in the database or not sql server
下面是我的代碼,我想在其中添加查詢並顯示用戶名存在的 toast 消息。 首先,我使用 Asynctask 方法在數據庫中插入值,但現在我不想重復用戶名。 用戶名應該是唯一的。 因此,當用戶鍵入用戶名時,它會突然檢查它是否存在於數據庫中並顯示 toast 消息。
package com.example.software2.bloodbankmanagement;
import android.annotation.SuppressLint;
import android.content.Context;
import android.content.Intent;
import android.graphics.drawable.BitmapDrawable;
import android.os.AsyncTask;
import android.os.Build;
import android.os.Bundle;
import android.os.Handler;
import android.os.Looper;
import android.os.StrictMode;
import android.os.VibrationEffect;
import android.os.Vibrator;
import android.provider.MediaStore;
import android.text.Editable;
import android.util.Log;
import android.view.View;
import android.view.animation.Animation;
import android.view.animation.AnimationUtils;
import android.view.inputmethod.EditorInfo;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.EditText;
import android.widget.RadioButton;
import android.widget.RadioGroup;
import android.widget.Spinner;
import android.widget.TextView;
import android.widget.Toast;
import androidx.annotation.RequiresApi;
import androidx.appcompat.app.AppCompatActivity;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResultSet;
import java.sql.Statement;
import java.io.ByteArrayOutputStream;
import java.io.FileNotFoundException;
import java.io.IOError;
import java.io.InputStream;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.List;
import android.annotation.SuppressLint;
import android.content.Intent;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.net.Uri;
import android.os.Bundle;
import android.os.Environment;
import android.os.StrictMode;
import android.util.AndroidRuntimeException;
import android.util.Base64;
import android.util.Log;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.ImageView;
import android.widget.ProgressBar;
import android.widget.TextView;
import android.widget.Toast;
public class RegisterActivity extends AppCompatActivity implements AdapterView.OnItemSelectedListener {
EditText name, email, name2, password,
contactno, Address, city, Age, username, gender;
Button registerbtn;
public static final int REQUEST_LOAD_IMAGE = 1;
ImageView img;
Button btnupload;
TextView auth;
byte[] byteArray;
ProgressBar progressBar;
String encodedImage;
Spinner spinner;
Connection con;
String gender1 = "male";
CheckBox blood;
RadioGroup radioGroup;
private static String value;
public String getvalue(){
return value;
}
Statement stmt;
static boolean isAllFieldsChecked = true;
private Context context;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.register);
RadioGroup rg = (RadioGroup) findViewById(R.id.radioGroup);
RadioButton gender_radio_male = (RadioButton) findViewById(R.id.Male);
RadioButton gender_radio_female = (RadioButton) findViewById(R.id.Female);
blood = findViewById(R.id.checkBox);
name = (EditText) findViewById(R.id.name);
name2 = (EditText) findViewById(R.id.name2);
username = (EditText) findViewById(R.id.username);
img = (ImageView) findViewById(R.id.img);
Age = (EditText) findViewById(R.id.age);
gender = (EditText) findViewById(R.id.gender);
btnupload = (Button) findViewById(R.id.btnupload);
progressBar = (ProgressBar) findViewById(R.id.progressBar);
email = (EditText) findViewById(R.id.email);
password = (EditText) findViewById(R.id.password);
contactno = (EditText) findViewById(R.id.contactno);
Address = (EditText) findViewById(R.id.Address);
progressBar.setVisibility(View.GONE);
city = findViewById(R.id.city);
registerbtn = (Button) findViewById(R.id.regbtn);
registerbtn.setOnClickListener(new View.OnClickListener() {
@RequiresApi(api = Build.VERSION_CODES.O)
@Override
public void onClick(View v) {
city.onEditorAction(EditorInfo.IME_ACTION_DONE);
isAllFieldsChecked = CheckAllFields();
new registeruser().execute("");
}
});
btnupload.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent galleryIntent = new Intent(Intent.ACTION_PICK, MediaStore.Images.Media.EXTERNAL_CONTENT_URI);
startActivityForResult(galleryIntent, REQUEST_LOAD_IMAGE);
}
});
}
@SuppressLint("NonConstantResourceId")
public void onRadioButtonClicked(View v) {
switch (v.getId()) {
case R.id.Male:
gender1 = "male";
break;
case R.id.Female:
gender1 = "female";
break;
}
}
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == REQUEST_LOAD_IMAGE && resultCode == RESULT_OK && null != data) {
Bitmap originBitmap = null;
Uri selectedImage = data.getData();
InputStream imageStream;
try {
imageStream = getContentResolver().openInputStream(selectedImage);
originBitmap = BitmapFactory.decodeStream(imageStream);
} catch (FileNotFoundException e) {
Toast.makeText(getApplicationContext(), (CharSequence) e, Toast.LENGTH_LONG).show();
}
if (originBitmap != null) {
this.img.setImageBitmap(originBitmap);
Bitmap image = ((BitmapDrawable) img.getDrawable()).getBitmap();
ByteArrayOutputStream byteArrayOutputStream = new ByteArrayOutputStream();
image.compress(Bitmap.CompressFormat.JPEG, 100, byteArrayOutputStream);
encodedImage = Base64.encodeToString(byteArrayOutputStream.toByteArray(), Base64.DEFAULT);
//new DoFetchList().execute("");
//new registeruser().execute("");
}
}
}
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position, long id) {
// On selecting a spinner item
final TextView textView = findViewById(R.id.auth);
String item = parent.getItemAtPosition(position).toString();
textView.setText(item);
}
public void onNothingSelected(AdapterView<?> arg0) {
}
public class registeruser extends AsyncTask<String, String , String>{
String z = "";
Boolean isSuccess = false;
@Override
protected void onPreExecute() {
Toast.makeText(RegisterActivity.this,"Please wait",Toast.LENGTH_LONG).show();
}
@SuppressLint("SetTextI18n")
@Override
protected void onPostExecute(String s) {
Toast.makeText(RegisterActivity.this, "Registration Successful", Toast.LENGTH_SHORT).show();
}
@Override
protected String doInBackground(String... strings) {
try{
con = connectionClass(MainActivity.ConnectionClass.un.toString(), MainActivity.ConnectionClass.pass.toString(), MainActivity.ConnectionClass.db.toString(), MainActivity.ConnectionClass.ip.toString());
if(con == null){
z = "Check Your Internet Connection";
}
else{
try {
final TextView textView = findViewById(R.id.auth);
stmt = con.createStatement();
String sql = "INSERT INTO register (FirstName,LastName,Age,gender,Email,Username,password,contactNo,Address,city,img,bloodgrp) VALUES ('"+name.getText()+"','"+name2.getText()+"',"+Age.getText()+",'"+gender1.trim()+"','"+email.getText()+"','"+username.getText()+"','"+password.getText()+"','"+contactno.getText()+"','"+Address.getText()+"','"+city.getText()+"','"+encodedImage+"','"+textView.getText()+"');";
stmt.executeUpdate(sql);
}
catch (Exception e){
z = "Username already exist";
}
StringBuilder result = new StringBuilder();
if(blood.isChecked()){
result.append("selected i want to be a donor");
result.toString().trim();
try {
stmt = con.createStatement();
String sql1 = "INSERT INTO DONOR (FirstName,LastName,contactNo,Address,city) VALUES ('"+name.getText()+"','"+name2.getText()+"','"+contactno.getText()+"','"+Address.getText()+"','"+city.getText()+"')";
stmt.executeUpdate(sql1);
// String sql2 = "insert into register (img) values ('"+ encodedImage+"')";
// PreparedStatement prstmt = con.prepareStatement(sql2);
// prstmt.execute();
Toast.makeText(RegisterActivity.this,"",Toast.LENGTH_LONG).show();
}
catch (Exception e){
z = "Please enter all fields and correct information";
}
}
// stmt.executeUpdate(sql);
}
}catch (Exception e){
z = "Please enter all fields and correct information";
//Toast.makeText(RegisterActivity.this, "Username is already exist", Toast.LENGTH_SHORT).show();
}
return z;
}
}
@SuppressLint("NewApi")
public Connection connectionClass(String user, String password, String database, String server){
StrictMode.ThreadPolicy policy = new StrictMode.ThreadPolicy.Builder().permitAll().build();
StrictMode.setThreadPolicy(policy);
Connection connection = null;
String connectionURL;
try{
Class.forName("net.sourceforge.jtds.jdbc.Driver");
connectionURL = "jdbc:jtds:sqlserver://" + server+"/" + database + ";user=" + user + ";password=" + password + ";";
connection = DriverManager.getConnection(connectionURL);
}catch (Exception e){
Log.e("SQL Connection Error : ", e.getMessage());
}
return connection;
}
}
欲了解更多詳情,請單擊此處
有兩種可能的解決方案,
1、寫一個檢查查詢,確保相同的數據是否已經存在於DB中
像 "int x = Select count(*) from register where username="+username.getText()
如果 x 值為 0,則可以插入新記錄,否則顯示錯誤消息。
if(x==0){
// insert the values into table
}else{
// show your error message
}
2、每次插入成功MySql返回一個long值,使用這個返回值可以確認插入數據到表中。
public class registeruser extends AsyncTask<String, String , String>{
String z = "";
Boolean isSuccess = false;
@Override
protected void onPreExecute() {
Toast.makeText(RegisterActivity.this,"Please wait",Toast.LENGTH_LONG).show();
}
@SuppressLint("SetTextI18n")
@Override
protected void onPostExecute(String s) {
Toast.makeText(RegisterActivity.this, "Registration Successful", Toast.LENGTH_SHORT).show();
}
@Override
protected String doInBackground(String... strings) {
try{
con = connectionClass(MainActivity.ConnectionClass.un.toString(), MainActivity.ConnectionClass.pass.toString(), MainActivity.ConnectionClass.db.toString(), MainActivity.ConnectionClass.ip.toString());
if(con == null){
z = "Check Your Internet Connection";
}
else{
try {
final TextView textView = findViewById(R.id.auth);
stmt = con.createStatement();
String checkQry = "Select count(*) from register where Username="+"'"+username.getText()+"'";
int count = stmt.executeUpdate(checkQry)
if(count == 0){
String sql = "INSERT INTO register (FirstName,LastName,Age,gender,Email,Username,password,contactNo,Address,city,img,bloodgrp) VALUES ('"+name.getText()+"','"+name2.getText()+"',"+Age.getText()+",'"+gender1.trim()+"','"+email.getText()+"','"+username.getText()+"','"+password.getText()+"','"+contactno.getText()+"','"+Address.getText()+"','"+city.getText()+"','"+encodedImage+"','"+textView.getText()+"');";
stmt.executeUpdate(sql);
}else{
z = "Username already exist";
}
}
catch (Exception e){
z = "Username already exist";
}
StringBuilder result = new StringBuilder();
if(blood.isChecked()){
result.append("selected i want to be a donor");
result.toString().trim();
try {
stmt = con.createStatement();
String sql1 = "INSERT INTO DONOR (FirstName,LastName,contactNo,Address,city) VALUES ('"+name.getText()+"','"+name2.getText()+"','"+contactno.getText()+"','"+Address.getText()+"','"+city.getText()+"')";
stmt.executeUpdate(sql1);
// String sql2 = "insert into register (img) values ('"+ encodedImage+"')";
// PreparedStatement prstmt = con.prepareStatement(sql2);
// prstmt.execute();
Toast.makeText(RegisterActivity.this,"",Toast.LENGTH_LONG).show();
}
catch (Exception e){
z = "Please enter all fields and correct information";
}
}
// stmt.executeUpdate(sql);
}
}catch (Exception e){
z = "Please enter all fields and correct information";
//Toast.makeText(RegisterActivity.this, "Username is already exist", Toast.LENGTH_SHORT).show();
}
return z;
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.