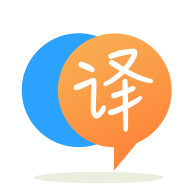
[英]How to fix 'Unknown word' error when bundling css file in webpack
[英]How to fix Error with alchemy-web3 - Webpack < 5
交互.js
require('dotenv').config();
const alchemyKey = process.env.REACT_APP_ALCHEMY_KEY;
import { createAlchemyWeb3 } from "@alch/alchemy-web3";
const web3 = createAlchemyWeb3(alchemyKey);
const contractABI = require('../contract-abi.json')
const contractAddress = "My_Contract_address";
export const mintNFT = async(url, name, description) => {
//error handling
if (url.trim() === "" || (name.trim() === "" || description.trim() === "")) {
return {
success: false,
status: "❗Please make sure all fields are completed before minting.",
}
}
const tokenURI = "ipfs://My_URI"
window.contract = await new web3.eth.Contract(contractABI, contractAddress);
//set up your Ethereum transaction
const transactionParameters = {
to: contractAddress, // Required except during contract publications.
from: window.ethereum.selectedAddress, // must match user's active address.
'data': window.contract.methods.mintPublic(window.ethereum.selectedAddress, tokenURI).encodeABI()//make call to NFT smart contract
};
//sign the transaction via Metamask
try {
const txHash = await window.ethereum
.request({
method: 'eth_sendTransaction',
params: [transactionParameters],
});
return {
success: true,
status: "✅ Check out your transaction on Etherscan: https://rinkeby.etherscan.io/tx/" + txHash
}
} catch (error) {
return {
success: false,
status: "😥 Something went wrong: " + error.message
}
}
}
export const connectWallet = async () => {
if (window.ethereum) {
try {
const addressArray = await window.ethereum.request({
method: "eth_requestAccounts",
});
const obj = {
status: "👆🏽 Write a message in the text-field above.",
address: addressArray[0],
};
return obj;
} catch (err) {
return {
address: "",
status: "😥 " + err.message,
};
}
} else {
return {
address: "",
status: (
<span>
<p>
{" "}
🦊{" "}
<a target="_blank" rel="noreferrer" href={`https://metamask.io/download.html`}>
You must install Metamask, a virtual Ethereum wallet, in your
browser.
</a>
</p>
</span>
),
};
}
};
export const getCurrentWalletConnected = async () => {
if (window.ethereum) {
try {
const addressArray = await window.ethereum.request({
method: "eth_accounts",
});
if (addressArray.length > 0) {
return {
address: addressArray[0],
status: "👆🏽 Write a message in the text-field above.",
};
} else {
return {
address: "",
status: "🦊 Connect to Metamask using the top right button.",
};
}
} catch (err) {
return {
address: "",
status: "😥 " + err.message,
};
}
} else {
return {
address: "",
status: (
<span>
<p>
{" "}
🦊{" "}
<a target="_blank" rel="noreferrer" href={`https://metamask.io/download.html`}>
You must install Metamask, a virtual Ethereum wallet, in your
browser.
</a>
</p>
</span>
),
};
}
};
我正在嘗試將煉金術連接到我的前端反應本機網站。 但是我收到了這個錯誤:
Compiled with problems:X
ERROR in ./node_modules/@alch/alchemy-web3/node_modules/eth-lib/lib/bytes.js 7:193-227
Module not found: Error: Can't resolve 'crypto' in '/Users/keyesbruh/Documents/Programming/nft-project/node_modules/@alch/alchemy-web3/node_modules/eth-lib/lib'
BREAKING CHANGE: webpack < 5 used to include polyfills for node.js core modules by default.
This is no longer the case. Verify if you need this module and configure a polyfill for it.
If you want to include a polyfill, you need to:
- add a fallback 'resolve.fallback: { "crypto": require.resolve("crypto-browserify") }'
- install 'crypto-browserify'
If you don't want to include a polyfill, you can use an empty module like this:
resolve.fallback: { "crypto": false }
ERROR in ./node_modules/@alch/alchemy-web3/node_modules/web3-eth-accounts/lib/index.js 31:74-91
Module not found: Error: Can't resolve 'crypto' in '/Users/keyesbruh/Documents/Programming/nft-project/node_modules/@alch/alchemy-web3/node_modules/web3-eth-accounts/lib'
BREAKING CHANGE: webpack < 5 used to include polyfills for node.js core modules by default.
This is no longer the case. Verify if you need this module and configure a polyfill for it.
If you want to include a polyfill, you need to:
- add a fallback 'resolve.fallback: { "crypto": require.resolve("crypto-browserify") }'
- install 'crypto-browserify'
If you don't want to include a polyfill, you can use an empty module like this:
resolve.fallback: { "crypto": false }
ERROR in ./node_modules/@alch/alchemy-web3/node_modules/web3-providers-http/lib/index.js 30:11-26
Module not found: Error: Can't resolve 'http' in '/Users/keyesbruh/Documents/Programming/nft-project/node_modules/@alch/alchemy-web3/node_modules/web3-providers-http/lib'
BREAKING CHANGE: webpack < 5 used to include polyfills for node.js core modules by default.
This is no longer the case. Verify if you need this module and configure a polyfill for it.
If you want to include a polyfill, you need to:
- add a fallback 'resolve.fallback: { "http": require.resolve("stream-http") }'
- install 'stream-http'
If you don't want to include a polyfill, you can use an empty module like this:
resolve.fallback: { "http": false }
ERROR in ./node_modules/@alch/alchemy-web3/node_modules/web3-providers-http/lib/index.js 32:12-28
Module not found: Error: Can't resolve 'https' in '/Users/keyesbruh/Documents/Programming/nft-project/node_modules/@alch/alchemy-web3/node_modules/web3-providers-http/lib'
BREAKING CHANGE: webpack < 5 used to include polyfills for node.js core modules by default.
This is no longer the case. Verify if you need this module and configure a polyfill for it.
If you want to include a polyfill, you need to:
- add a fallback 'resolve.fallback: { "https": require.resolve("https-browserify") }'
- install 'https-browserify'
If you don't want to include a polyfill, you can use an empty module like this:
resolve.fallback: { "https": false }
ERROR in ./node_modules/assert/assert.js
Module build failed (from ./node_modules/source-map-loader/dist/cjs.js):
Error: ENOENT: no such file or directory, open '/Users/keyesbruh/Documents/Programming/nft-project/node_modules/assert/assert.js'
ERROR in ./node_modules/cipher-base/index.js 3:16-43
Module not found: Error: Can't resolve 'stream' in '/Users/keyesbruh/Documents/Programming/nft-project/node_modules/cipher-base'
BREAKING CHANGE: webpack < 5 used to include polyfills for node.js core modules by default.
This is no longer the case. Verify if you need this module and configure a polyfill for it.
If you want to include a polyfill, you need to:
- add a fallback 'resolve.fallback: { "stream": require.resolve("stream-browserify") }'
- install 'stream-browserify'
If you don't want to include a polyfill, you can use an empty module like this:
resolve.fallback: { "stream": false }
ERROR in ./node_modules/dotenv/lib/main.js 24:11-24
Module not found: Error: Can't resolve 'fs' in '/Users/keyesbruh/Documents/Programming/nft-project/node_modules/dotenv/lib'
ERROR in ./node_modules/dotenv/lib/main.js 26:13-28
Module not found: Error: Can't resolve 'path' in '/Users/keyesbruh/Documents/Programming/nft-project/node_modules/dotenv/lib'
BREAKING CHANGE: webpack < 5 used to include polyfills for node.js core modules by default.
This is no longer the case. Verify if you need this module and configure a polyfill for it.
If you want to include a polyfill, you need to:
- add a fallback 'resolve.fallback: { "path": require.resolve("path-browserify") }'
- install 'path-browserify'
If you don't want to include a polyfill, you can use an empty module like this:
resolve.fallback: { "path": false }
ERROR in ./node_modules/dotenv/lib/main.js 28:11-24
Module not found: Error: Can't resolve 'os' in '/Users/keyesbruh/Documents/Programming/nft-project/node_modules/dotenv/lib'
BREAKING CHANGE: webpack < 5 used to include polyfills for node.js core modules by default.
This is no longer the case. Verify if you need this module and configure a polyfill for it.
If you want to include a polyfill, you need to:
- add a fallback 'resolve.fallback: { "os": require.resolve("os-browserify/browser") }'
- install 'os-browserify'
If you don't want to include a polyfill, you can use an empty module like this:
resolve.fallback: { "os": false }
ERROR in ./node_modules/eth-lib/lib/bytes.js 9:193-227
Module not found: Error: Can't resolve 'crypto' in '/Users/keyesbruh/Documents/Programming/nft-project/node_modules/eth-lib/lib'
BREAKING CHANGE: webpack < 5 used to include polyfills for node.js core modules by default.
This is no longer the case. Verify if you need this module and configure a polyfill for it.
If you want to include a polyfill, you need to:
- add a fallback 'resolve.fallback: { "crypto": require.resolve("crypto-browserify") }'
- install 'crypto-browserify'
If you don't want to include a polyfill, you can use an empty module like this:
resolve.fallback: { "crypto": false }
ERROR in ./node_modules/xhr2-cookies/dist/xml-http-request.js 37:11-26
Module not found: Error: Can't resolve 'http' in '/Users/keyesbruh/Documents/Programming/nft-project/node_modules/xhr2-cookies/dist'
BREAKING CHANGE: webpack < 5 used to include polyfills for node.js core modules by default.
This is no longer the case. Verify if you need this module and configure a polyfill for it.
If you want to include a polyfill, you need to:
- add a fallback 'resolve.fallback: { "http": require.resolve("stream-http") }'
- install 'stream-http'
If you don't want to include a polyfill, you can use an empty module like this:
resolve.fallback: { "http": false }
ERROR in ./node_modules/xhr2-cookies/dist/xml-http-request.js 39:12-28
Module not found: Error: Can't resolve 'https' in '/Users/keyesbruh/Documents/Programming/nft-project/node_modules/xhr2-cookies/dist'
BREAKING CHANGE: webpack < 5 used to include polyfills for node.js core modules by default.
This is no longer the case. Verify if you need this module and configure a polyfill for it.
If you want to include a polyfill, you need to:
- add a fallback 'resolve.fallback: { "https": require.resolve("https-browserify") }'
- install 'https-browserify'
If you don't want to include a polyfill, you can use an empty module like this:
resolve.fallback: { "https": false }
ERROR in ./node_modules/xhr2-cookies/dist/xml-http-request.js 41:9-22
Module not found: Error: Can't resolve 'os' in '/Users/keyesbruh/Documents/Programming/nft-project/node_modules/xhr2-cookies/dist'
BREAKING CHANGE: webpack < 5 used to include polyfills for node.js core modules by default.
This is no longer the case. Verify if you need this module and configure a polyfill for it.
If you want to include a polyfill, you need to:
- add a fallback 'resolve.fallback: { "os": require.resolve("os-browserify/browser") }'
- install 'os-browserify'
If you don't want to include a polyfill, you can use an empty module like this:
resolve.fallback: { "os": false }
我之前在以下線程中解決了這個錯誤: ERROR: Webpack < 5 after installed web3 and implementation into React Native App.js
但那是通過以不同的方式導入 web3。 這個煉金術我不確定是否有其他方法可以導入它。 謝謝。
我最近也遇到了這個問題。 根據我的研究,我發現這是 Webpack 的一個已知問題。 我使用的是 react-scripts 5.0.0 版。 對我來說最簡單的解決方案是在我的項目中使用早期版本的 react-scripts,刪除我的 node_modules 目錄並重新運行npm install
。
// package.json
{
...normal package.json attributes
"dependencies": {
...other dependencies,
"react-scripts": "4.0.3"
}
}
聲明:本站的技術帖子網頁,遵循CC BY-SA 4.0協議,如果您需要轉載,請注明本站網址或者原文地址。任何問題請咨詢:yoyou2525@163.com.